
newest version,
Embed:
(wiki syntax)
Show/hide line numbers
update.cpp
00001 /* 00002 * update.cpp 00003 * 00004 * Created on: Oct 16, 2015 00005 * Author: User 00006 */ 00007 00008 00009 #include <iostream> 00010 #include <string> 00011 #include "move_motor.h"; 00012 /* 00013 * takes state and num_on_inputs as input variables, if num_on_inputs means been in one state for 0.25s 00014 * it calls the motor movement function according to the state (left/right or key press) 00015 */ 00016 void update(std::string state, int &num_on_inputs) 00017 { 00018 //check if system has been in one state long enough to move 00019 if (num_on_inputs == 250) 00020 { 00021 //reset the number of on inputs so that once function finishes robot is 'reinitialised' 00022 num_on_inputs = 0; 00023 //run left/right motor, key press motor or do nothing depending on the state 00024 if (state == "left" or state == "right" or state == "keypress") 00025 { 00026 //function to be created and included as h file 00027 move_motor(state); 00028 //std::cout << "left or right \n"; 00029 } 00030 00031 else if (state == "rest") 00032 { 00033 //if at rest do nothing 00034 //std::cout << "rest "; 00035 } 00036 else 00037 { 00038 //throw an error somehow 00039 //std::cout << "error \n "; 00040 } 00041 } 00042 else if (num_on_inputs > 250) 00043 { 00044 //std::cout << "error \n "; 00045 } 00046 } 00047 00048 /* 00049 void update(std::string state, int &num_on_inputs) 00050 { 00051 //check if system has been in one state long enough to move 00052 if (num_on_inputs == 250) 00053 { 00054 //reset the number of on inputs so that once function finishes robot is 'reinitialised' 00055 num_on_inputs = 0; 00056 //run left/right motor, key press motor or do nothing depending on the state 00057 if (state == "left" or state == "right") 00058 { 00059 //function to be created and included as h file 00060 //move_horizontal_motor(state) 00061 std::cout << "left or right \n"; 00062 } 00063 else if (state == "keypress") 00064 { 00065 //function to be created and its h file included 00066 //move_keypress_motor() 00067 std::cout << "keypress \n "; 00068 } 00069 else if (state == "rest") 00070 { 00071 //if at rest do nothing 00072 std::cout << "rest "; 00073 } 00074 else 00075 { 00076 //throw an error somehow 00077 std::cout << "error \n "; 00078 } 00079 } 00080 else if (num_on_inputs > 250) 00081 { 00082 std::cout << "error \n "; 00083 } 00084 } 00085 00086 int main() 00087 { 00088 std::string state = "left"; 00089 int num_on_inputs = 250; 00090 00091 update(state, num_on_inputs); 00092 00093 std::cout << num_on_inputs << "!\n"; 00094 00095 return 0; 00096 } 00097 */ 00098 00099 00100
Generated on Thu Aug 4 2022 19:30:43 by
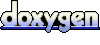