
Adaptation of the esp8266-driver demo program to control a GOPRO HERO3+ camera. This demo can only take a photo
Fork of mbed-os-example-mbed5-wifi by
main.cpp
00001 /* WiFi Example 00002 * Copyright (c) 2016 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 /* adaptation pour demo commande camera GOPRO 00018 * C.Dupaty 04-2018 00019 */ 00020 00021 #include "mbed.h" 00022 #include "TCPSocket.h" 00023 00024 #define WIFI_ESP8266 1 00025 #define WIFI_IDW0XX1 2 00026 00027 #if TARGET_UBLOX_EVK_ODIN_W2 00028 #include "OdinWiFiInterface.h" 00029 OdinWiFiInterface wifi; 00030 00031 #elif TARGET_REALTEK_RTL8195AM 00032 #include "RTWInterface.h" 00033 RTWInterface wifi; 00034 00035 #else // External WiFi modules 00036 00037 #if MBED_CONF_APP_WIFI_SHIELD == WIFI_ESP8266 00038 #include "ESP8266Interface.h" 00039 ESP8266Interface wifi(MBED_CONF_APP_WIFI_TX, MBED_CONF_APP_WIFI_RX); 00040 #elif MBED_CONF_APP_WIFI_SHIELD == WIFI_IDW0XX1 00041 #include "SpwfSAInterface.h" 00042 SpwfSAInterface wifi(MBED_CONF_APP_WIFI_TX, MBED_CONF_APP_WIFI_RX); 00043 #endif // MBED_CONF_APP_WIFI_SHIELD == WIFI_IDW0XX1 00044 00045 #endif 00046 00047 Serial pc(USBTX,USBRX); 00048 00049 char photo[]="GET /bacpac/SH?t=goprofourcade&p=%01 HTTP/1.0\r\n\r\n"; // requete de prise de vue 00050 00051 00052 const char *sec2str(nsapi_security_t sec) 00053 { 00054 switch (sec) { 00055 case NSAPI_SECURITY_NONE: 00056 return "None"; 00057 case NSAPI_SECURITY_WEP: 00058 return "WEP"; 00059 case NSAPI_SECURITY_WPA: 00060 return "WPA"; 00061 case NSAPI_SECURITY_WPA2: 00062 return "WPA2"; 00063 case NSAPI_SECURITY_WPA_WPA2: 00064 return "WPA/WPA2"; 00065 case NSAPI_SECURITY_UNKNOWN: 00066 default: 00067 return "Unknown"; 00068 } 00069 } 00070 00071 int scan_demo(WiFiInterface *wifi) 00072 { 00073 WiFiAccessPoint *ap; 00074 00075 pc.printf("Scan:\n"); 00076 00077 int count = wifi->scan(NULL,0); 00078 00079 /* Limit number of network arbitrary to 15 */ 00080 count = count < 15 ? count : 15; 00081 00082 ap = new WiFiAccessPoint[count]; 00083 count = wifi->scan(ap, count); 00084 for (int i = 0; i < count; i++) 00085 { 00086 pc.printf("Network: %s secured: %s BSSID: %hhX:%hhX:%hhX:%hhx:%hhx:%hhx RSSI: %hhd Ch: %hhd\n", ap[i].get_ssid(), 00087 sec2str(ap[i].get_security()), ap[i].get_bssid()[0], ap[i].get_bssid()[1], ap[i].get_bssid()[2], 00088 ap[i].get_bssid()[3], ap[i].get_bssid()[4], ap[i].get_bssid()[5], ap[i].get_rssi(), ap[i].get_channel()); 00089 } 00090 pc.printf("%d networks available.\n", count); 00091 00092 delete[] ap; 00093 return count; 00094 } 00095 00096 void envoie_commande(NetworkInterface *net, char *sbuffer) 00097 { 00098 pc.printf("\x1B[33m"); //texte jaune 00099 TCPSocket socket; 00100 nsapi_error_t response; 00101 00102 pc.printf("Sending HTTP request to gopro...\n"); 00103 00104 // Open a socket on the network interface, and create a TCP connection to www.arm.com 00105 socket.open(net); 00106 response = socket.connect("10.5.5.9", 80); 00107 if(0 != response) { 00108 pc.printf("Error connecting: %d\n", response); 00109 socket.close(); 00110 pc.printf("\x1B[37m"); //texte blanc 00111 return; 00112 } 00113 else pc.printf("Connexion etablie\r\n"); 00114 00115 // Send a simple http request 00116 // char sbuffer[] = "GET /bacpac/SH?t=goprofourcade&p=%01 HTTP/1.0\r\n\r\n"; 00117 // char sbuffer[] = "GET / HTTP/1.1\r\nhost: 10.5.5.9\r\n\r\n"; 00118 nsapi_size_t size = strlen(sbuffer); 00119 response = 0; 00120 while(size) 00121 { 00122 response = socket.send(sbuffer+response, size); 00123 if (response < 0) { 00124 pc.printf("Error sending data: %d\n", response); 00125 socket.close(); 00126 pc.printf("\x1B[37m"); //texte blanc 00127 return; 00128 } else { 00129 size -= response; 00130 // Check if entire message was sent or not 00131 pc.printf("sent %d [%.*s]\n", response, strstr(sbuffer, "\r\n")-sbuffer, sbuffer); 00132 } 00133 } 00134 00135 // Recieve a simple http response and print out the response line 00136 char rbuffer[64]; 00137 response = socket.recv(rbuffer, sizeof rbuffer); 00138 if (response < 0) { 00139 pc.printf("Error receiving data: %d\n", response); 00140 } else { 00141 pc.printf("recv %d [%.*s]\n", response, strstr(rbuffer, "\r\n")-rbuffer, rbuffer); 00142 pc.printf("%s\r\n",rbuffer); 00143 } 00144 00145 // Close the socket to return its memory and bring down the network interface 00146 socket.close(); 00147 pc.printf("\x1B[37m"); //texte blanc 00148 } 00149 00150 int main() 00151 { 00152 // int count = 0; 00153 pc.printf("\x1B[2J"); //efface ecran 00154 pc.printf("\x1B[0;0H"); // curseur en 0,0 00155 pc.printf("\x1B[37m"); //texte blanc 00156 pc.printf("-----------------------------------------------------------\r\n"); 00157 pc.printf("Exemple de controle de camera GOPRO par WIFI\r\n"); 00158 pc.printf("La camera GOPRO doit etre en marche, WIFI actif, mode photo\r\n"); 00159 pc.printf("-----------------------------------------------------------\r\n"); 00160 /* // Exploration 00161 count = scan_demo(&wifi); 00162 if (count == 0) { 00163 pc.printf("No WIFI APNs found - can't continue further.\n"); 00164 return -1; 00165 } 00166 */ 00167 pc.printf("\nTentative de connection sur la borne WIFI %s...\n", MBED_CONF_APP_WIFI_SSID); 00168 int ret = wifi.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, NSAPI_SECURITY_WPA_WPA2); 00169 if (ret != 0) { 00170 pc.printf("\nConnection error\n"); 00171 return -1; 00172 } 00173 00174 pc.printf("Connexion reussie\n\n"); 00175 pc.printf("MAC: %s\n", wifi.get_mac_address()); 00176 pc.printf("IP: %s\n", wifi.get_ip_address()); 00177 pc.printf("Netmask: %s\n", wifi.get_netmask()); 00178 pc.printf("Gateway: %s\n", wifi.get_gateway()); 00179 pc.printf("RSSI: %d\n\n", wifi.get_rssi()); 00180 00181 char * commande = photo; // un exemple pour prendre une photo 00182 // la camera doit activee être en mode photo 00183 char c='a'; 00184 while(c!='q') 00185 { 00186 while(pc.readable())c=pc.getc(); 00187 pc.printf("\r\nTaper q pour quitter\n\r"); 00188 pc.printf("ou n'importe quelle touche pour prendre une photo \n\r"); 00189 while(!pc.readable()); 00190 envoie_commande(&wifi,commande); 00191 } 00192 wifi.disconnect(); 00193 00194 pc.printf("Wifi deconnecte - fin du programme\n\r"); 00195 while(1); 00196 }
Generated on Sun Jul 24 2022 10:59:45 by
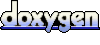