
This Socket Example Program Implements a TCP Socket, UDP Socket, and HTTPClient socket then uses each of these to perform basic I/O.
Dependencies: JSON M2XStreamClient-JMF WNCInterface mbed-rtos mbed
main.cpp
00001 /* ===================================================================== 00002 Copyright © 2016, Avnet (R) 00003 00004 Contributors: 00005 * James M Flynn, www.em.avnet.com 00006 00007 Licensed under the Apache License, Version 2.0 (the "License"); 00008 you may not use this file except in compliance with the License. 00009 You may obtain a copy of the License at 00010 00011 http://www.apache.org/licenses/LICENSE-2.0 00012 00013 Unless required by applicable law or agreed to in writing, 00014 software distributed under the License is distributed on an 00015 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00016 either express or implied. See the License for the specific 00017 language governing permissions and limitations under the License. 00018 00019 @file main.cpp / WNCInterface_HTTPS_Example 00020 @version 1.0 00021 @date Dec 2016 00022 00023 ======================================================================== */ 00024 00025 #include "mbed.h" 00026 00027 #include "WNCInterface.h" 00028 00029 #include "Socket/Socket.h" 00030 #include "Socket/TCPSocketConnection.h" 00031 #include "Socket/UDPSocket.h" 00032 00033 #define DEBUG 00034 #define MBED_PLATFORM 00035 00036 #include "HTTPClient.h" 00037 00038 #define STREAM_CNT 15 //when we test streaming, this is how many times to stream the string 00039 #define STR_SIZE 125*(STREAM_CNT+1) //use a fixed size string buffer based on the streaming data count 00040 #define CRLF "\n\r" 00041 00042 // 00043 // This example is setup to use MBED OS (5.2). It sets up a thread to call the different tests 00044 // because mbed tls is stack intensive and there is no way to modify the default stack size for 00045 // main. So when we create the test task, give it a large stack (x4 the default size). After 00046 // this the main() task will just spin. 00047 // 00048 00049 void https_test_thread(void); 00050 00051 int main() { 00052 Thread http_test(osPriorityNormal, DEFAULT_STACK_SIZE*4, NULL); 00053 00054 printf("Testing HTTPClient and HTTPSClient using the WNCInterface & Socket software" CRLF); 00055 00056 http_test.start(https_test_thread); 00057 while (true) { 00058 osDelay(500); 00059 } 00060 } 00061 00062 // 00063 // The two test functions do the same set of tests, the first one uses standard HTTP methods while 00064 // the second test uses HTTP methods in conjunction with a SSL/TLS connection to verify certificates. 00065 // 00066 00067 void test_http(void); //function tests the standard HTTPClient class 00068 void test_https(void); //function to test the HTTPSClient class 00069 00070 void https_test_thread(void) { 00071 int ret; 00072 00073 WNCInterface wnc; 00074 00075 ret = wnc.init(); 00076 printf("WNC Module %s initialized (%02X)." CRLF, ret?"IS":"IS NOT", ret); 00077 if( !ret ) { 00078 printf(" - - - - - - - ALL DONE - - - - - - - " CRLF); 00079 while(1); 00080 } 00081 00082 ret = wnc.connect(); 00083 printf("IP Address: %s " CRLF CRLF, wnc.getIPAddress()); 00084 00085 test_http(); 00086 test_https(); 00087 00088 wnc.disconnect(); 00089 printf(" - - - - - - - ALL DONE - - - - - - - " CRLF); 00090 while(1) {} 00091 } 00092 00093 00094 00095 //- - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 00096 // test the HTTP client class 00097 // 00098 void test_http(void) { 00099 HTTPClient http; 00100 char str[STR_SIZE]; 00101 int ret; 00102 00103 printf(">>>>>>>>>>>><<<<<<<<<<<<" CRLF); 00104 printf(">>> TEST HTTPClient <<< "CRLF); 00105 printf(">>>>>>>>>>>><<<<<<<<<<<<" CRLF CRLF); 00106 00107 //GET data 00108 printf(" ** Fetch a page... **" CRLF); 00109 00110 ret = http.get("https://developer.mbed.org/media/uploads/mbed_official/hello.txt", str, STR_SIZE); 00111 if (!ret) { 00112 printf("Page fetched successfully - read %d characters" CRLF, strlen(str)); 00113 printf("<----->" CRLF "Result: %s" CRLF "<----->" CRLF, str); 00114 } 00115 else 00116 printf("Error - ret = %d - HTTP return code = %d" CRLF, ret, http.getHTTPResponseCode()); 00117 00118 //POST data 00119 HTTPMap map; 00120 HTTPText inText(str, STR_SIZE); 00121 00122 map.put("Hello", "World"); 00123 map.put("test", "1234"); 00124 00125 printf(CRLF CRLF " ** Post data... **" CRLF); 00126 ret = http.post("http://httpbin.org/post", map, &inText); 00127 if (!ret) { 00128 printf("Executed POST successfully - read %d characters" CRLF, strlen(str)); 00129 printf("<----->" CRLF ); 00130 printf("Result: %s" CRLF "<----->" CRLF, str); 00131 } 00132 else 00133 printf("Error - ret = %d - HTTP return code = %d" CRLF, ret, http.getHTTPResponseCode()); 00134 00135 //PUT data 00136 00137 strcpy(str, "This is a PUT test!"); 00138 HTTPText outText(str); 00139 printf(CRLF CRLF " ** Put data... **" CRLF); 00140 00141 ret = http.put("http://httpbin.org/put", outText, &inText); 00142 if (!ret) { 00143 printf("Executed PUT successfully - read %d characters" CRLF, strlen(str)); 00144 printf("<----->" CRLF ); 00145 printf("Result: %s" CRLF "<----->" CRLF, str); 00146 } 00147 else 00148 printf("Error - ret = %d - HTTP return code = %d" CRLF, ret, http.getHTTPResponseCode()); 00149 00150 //DELETE data 00151 printf(CRLF CRLF " ** Delete data... **" CRLF); 00152 ret = http.del("http://httpbin.org/delete", &inText); 00153 if (!ret) { 00154 printf("Executed DELETE successfully - read %d characters" CRLF, strlen(str)); 00155 printf("<----->" CRLF ); 00156 printf("Result: %s" CRLF "<----->" CRLF, str); 00157 } 00158 else 00159 printf("Error - ret = %d - HTTP return code = %d" CRLF, ret, http.getHTTPResponseCode()); 00160 00161 printf(CRLF CRLF " ** HTTP:stream data " INTSTR(STREAM_CNT) " times... **" CRLF); 00162 if( (ret=http.get("http://httpbin.org:80/stream/" INTSTR(STREAM_CNT), str,sizeof(str))) == HTTP_OK) { 00163 printf(CRLF "STREAM successfull - returned %d characters" CRLF, strlen(str)); 00164 printf("<----->" CRLF "Result:" CRLF "%s" CRLF "<----->" CRLF CRLF, str); 00165 } 00166 else 00167 printf(CRLF "STREAM FAILED!, returned %d" CRLF, ret); 00168 00169 00170 printf(CRLF CRLF ">>>>HTTP:Status..." CRLF); 00171 ret = http.get("http://httpbin.org/get?show_env=1", str, STR_SIZE); 00172 if (!ret) { 00173 printf("Executed STATUS successfully - read %d characters" CRLF, strlen(str)); 00174 printf("<----->" CRLF ); 00175 printf("Result: %s" CRLF "<----->" CRLF, str); 00176 } 00177 else { 00178 printf("Error - ret = %d - HTTP return code = -0x%04X" CRLF, ret, -http.getHTTPResponseCode()); 00179 } 00180 00181 } 00182 00183 00184 //- - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - 00185 // test the HTTPS client class 00186 // 00187 void test_https(void) { 00188 HTTPSClient *https = new HTTPSClient; //each time you call a Host, it sets the CA Certificate 00189 //for that host. Because we call two different hosts it 00190 //is easier to create the class on the heap rather than 00191 //statically... 00192 char str[STR_SIZE]; 00193 int ret; 00194 00195 https->setHost("developer.mbed.org"); 00196 https->addRootCACertificate(SSL_CA_PEM); //this is the CA certificate for mbed.org 00197 00198 printf(">>>>>>>>>>>><<<<<<<<<<<<" CRLF); 00199 printf(">>> TEST HTTPSClient <<<" CRLF); 00200 printf(">>>>>>>>>>>><<<<<<<<<<<<" CRLF CRLF); 00201 00202 //GET data 00203 00204 memset(str,0x00,STR_SIZE); 00205 printf(CRLF " ** HTTPS:Fetch a page... **" CRLF); 00206 00207 ret = https->get("https://developer.mbed.org/media/uploads/mbed_official/hello.txt", str, STR_SIZE); 00208 if (!ret){ 00209 printf("Page fetched successfully - read %d characters" CRLF, strlen(str)); 00210 printf("<----->" CRLF "Result: %s" CRLF "<----->" CRLF, str); 00211 } 00212 else 00213 printf("Error - ret = %d - HTTP return code = -0x%04X" CRLF, ret, -https->getHTTPResponseCode()); 00214 00215 delete https; //go ahead and delete the mbed.org hppts client 00216 https = new HTTPSClient; //and create one for httbin.org 00217 https->setHost("httpbin.org"); 00218 https->addRootCACertificate(SSL_CA_HTTPBIN); //set the CA certificate for httpbin.org 00219 00220 HTTPMap map; 00221 HTTPText inText(str, STR_SIZE); 00222 00223 //POST data 00224 map.put("https Testing", "https doing a Post"); 00225 printf(CRLF CRLF ">>>>HTTPS:Post data..." CRLF); 00226 ret = https->post("https://httpbin.org:443/post", map, &inText); 00227 if (!ret) { 00228 printf("Executed POST successfully - read %d characters" CRLF, strlen(str)); 00229 printf("<----->" CRLF ); 00230 printf("Result: %s" CRLF "<----->" CRLF, str); 00231 } 00232 else 00233 printf("Error - ret = %d - HTTP return code = -0x%04X" CRLF, ret, -https->getHTTPResponseCode()); 00234 00235 //PUT data 00236 memset(str,0x00,STR_SIZE); 00237 strcpy(str, "This is an HTTPS PUT test!"); 00238 HTTPText outText(str); 00239 printf(CRLF CRLF ">>>>HTTPS:Put data..." CRLF); 00240 ret = https->put("https://httpbin.org:443/put", outText, &inText); 00241 if (!ret) { 00242 printf("Executed PUT successfully - read %d characters" CRLF, strlen(str)); 00243 printf("<----->" CRLF ); 00244 printf("Result: %s" CRLF "<----->" CRLF, str); 00245 } 00246 else 00247 printf("Error - ret = %d - HTTP return code = -0x%04X" CRLF, ret, -https->getHTTPResponseCode()); 00248 00249 printf(CRLF CRLF ">>>>HTTPS:stream data..." CRLF); 00250 if( (ret=https->get("https://httpbin.org:443/stream/" INTSTR(STREAM_CNT), str, STR_SIZE)) == HTTP_OK) { 00251 printf(CRLF "STREAM successfull - returned %d characters" CRLF, strlen(str)); 00252 printf("<----->" CRLF "Result:" CRLF "%s" CRLF "<----->" CRLF, str); 00253 } 00254 else 00255 printf(CRLF "STREAM FAILED!, returned %d - HTTP return code = -x%04X" CRLF, ret, -https->getHTTPResponseCode()); 00256 00257 //DELETE data 00258 printf(CRLF CRLF ">>>>HTTPS:Delete data..." CRLF); 00259 ret = https->del("https://httpbin.org/delete", &inText); 00260 if (!ret) { 00261 printf("Executed DELETE successfully - read %d characters" CRLF, strlen(str)); 00262 printf("<----->" CRLF ); 00263 printf("Result: %s" CRLF "<----->" CRLF, str); 00264 } 00265 else { 00266 printf("Error - ret = %d - HTTP return code = -0x%04X" CRLF, ret, -https->getHTTPResponseCode()); 00267 } 00268 00269 printf(CRLF CRLF ">>>>HTTPS:Status..." CRLF); 00270 ret = https->get("https://httpbin.org:443/get?show_env=1", str, STR_SIZE); 00271 if (!ret) { 00272 printf("Executed STATUS successfully - read %d characters" CRLF, strlen(str)); 00273 printf("<----->" CRLF ); 00274 printf("Result: %s" CRLF "<----->" CRLF, str); 00275 } 00276 else { 00277 printf("Error - ret = %d - HTTP return code = -0x%04X" CRLF, ret, -https->getHTTPResponseCode()); 00278 } 00279 00280 delete https; //all done, delete the httpbin.org https client 00281 } 00282
Generated on Sat Jul 16 2022 21:48:51 by
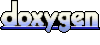