Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
timer.cpp
00001 /**************************************************************************//** 00002 * @file timer.cpp 00003 * @brief Implementation of Timer MBED Platform Driver Interfaces 00004 ******************************************************************************* 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 ******************************************************************************/ 00012 00013 /*****************************************************************************/ 00014 /***************************** Include Files *********************************/ 00015 /*****************************************************************************/ 00016 00017 #include <stdio.h> 00018 #include <mbed.h> 00019 00020 //Platform Drivers need to be C-compatible to work with other drivers 00021 #ifdef __cplusplus 00022 extern "C" 00023 { 00024 #endif //__cplusplus 00025 00026 #include "timer.h" 00027 #include "error.h" 00028 #include "timer_extra.h" 00029 using namespace std::chrono; 00030 00031 /*****************************************************************************/ 00032 /******************** Variables and User Defined Data Types ******************/ 00033 /*****************************************************************************/ 00034 00035 /*****************************************************************************/ 00036 /************************** Functions Declarations ***************************/ 00037 /*****************************************************************************/ 00038 00039 /*****************************************************************************/ 00040 /************************** Functions Definitions ****************************/ 00041 /*****************************************************************************/ 00042 00043 /** 00044 * @brief Initialize the timer peripheral 00045 * @param desc[in, out] - The Timer descriptor 00046 * @param init_param[in] - Structure that contains Timer Initialization Parameters 00047 * @return SUCCESS in case of success, FAILURE otherwise 00048 */ 00049 int32_t timer_init(struct timer_desc **desc, 00050 struct timer_init_param *param) 00051 { 00052 timer_desc *new_timer; // Pointer to timer descriptor 00053 mbed_timer_desc *mbed_desc; // Pointer to mbed timer descriptor 00054 mbed::Timer *timer; // Pointer to new Timer instance 00055 00056 if (desc && param) { 00057 new_timer = (timer_desc *)malloc(sizeof(timer_desc)); 00058 if (!new_timer) { 00059 goto err_new_desc; 00060 } 00061 new_timer->freq_hz = param->freq_hz; 00062 new_timer->id = param->id; 00063 new_timer->load_value = param->load_value; 00064 00065 mbed_desc = (mbed_timer_desc *)malloc(sizeof(mbed_timer_desc)); 00066 if (!mbed_desc) { 00067 goto err_mbed_desc; 00068 } 00069 00070 timer = new Timer(); 00071 if (!timer) { 00072 goto err_timer_instance; 00073 } 00074 00075 mbed_desc->timer = timer; 00076 new_timer->extra = (mbed_timer_desc *) mbed_desc; 00077 00078 *desc = new_timer; 00079 return SUCCESS; 00080 } 00081 return FAILURE; 00082 00083 err_timer_instance: 00084 free(mbed_desc); 00085 free(new_timer); 00086 err_mbed_desc: 00087 free(new_timer); 00088 err_new_desc: 00089 // Nothing to free 00090 00091 return FAILURE; 00092 } 00093 00094 00095 /** 00096 * @brief Start Timer. 00097 * @param desc[in] - The Timer Descriptor. 00098 * @return SUCCESS in case of success, FAILURE otherwise. 00099 */ 00100 int32_t timer_start(struct timer_desc *desc) 00101 { 00102 mbed::Timer *timer; 00103 00104 if (!desc) { 00105 return FAILURE; 00106 } 00107 timer = (Timer *)(((mbed_timer_desc *)(desc->extra))->timer); 00108 if (!timer) { 00109 return FAILURE; 00110 } 00111 timer->start(); 00112 00113 return SUCCESS; 00114 } 00115 00116 00117 /** 00118 * @brief Stop Timer. 00119 * @param desc[in] - The Timer Descriptor. 00120 * @return SUCCESS in case of success, FAILURE otherwise. 00121 */ 00122 int32_t timer_stop(struct timer_desc *desc) 00123 { 00124 mbed::Timer *timer; 00125 00126 if (!desc) { 00127 return FAILURE; 00128 } 00129 timer = (Timer *)(((mbed_timer_desc *)(desc->extra))->timer); 00130 if (!timer) { 00131 return FAILURE; 00132 } 00133 timer->stop(); 00134 00135 return SUCCESS; 00136 } 00137 00138 00139 /** 00140 * @brief Release all the resources allocated by Timer. 00141 * @param desc[in] - The Timer Descriptor. 00142 * @return SUCCESS in case of success, FAILURE otherwise 00143 */ 00144 int32_t timer_remove(struct timer_desc *desc) 00145 { 00146 if (desc) { 00147 // Free the timer object 00148 if ((Timer *)(((mbed_timer_desc *)(desc->extra))->timer)) { 00149 delete((Timer *)(((mbed_timer_desc *)(desc->extra))->timer)); 00150 } 00151 // Free the extra descriptor object 00152 if ((mbed_timer_desc *)(desc->extra)) { 00153 free((mbed_timer_desc *)(desc->extra)); 00154 } 00155 // Free the Timer descriptor 00156 free(desc); 00157 00158 return SUCCESS; 00159 } 00160 return FAILURE; 00161 } 00162 00163 00164 /** 00165 * @brief Get the elapsed time in nanoseconds. 00166 * @param desc[in] - The Timer descriptor 00167 * @param elapsed_time[out] - Pointer where the elapsed time value is stored 00168 * @return SUCCESS in case of success, FAILURE otherwise 00169 */ 00170 int32_t get_elapsed_time_in_nsec(struct timer_desc *desc, 00171 uint64_t *elapsed_time) 00172 { 00173 mbed::Timer *timer; 00174 00175 if (!desc) { 00176 return FAILURE; 00177 } 00178 00179 timer = (Timer *)(((mbed_timer_desc *)(desc->extra))->timer); 00180 if (!timer) { 00181 return FAILURE; 00182 } 00183 *elapsed_time = duration_cast<nanoseconds>(timer->elapsed_time()).count(); 00184 00185 return SUCCESS; 00186 } 00187 00188 #ifdef __cplusplus 00189 } 00190 #endif // __cplusplus
Generated on Wed Jul 13 2022 14:37:51 by
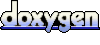