Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
spi_extra.h
00001 /***************************************************************************//** 00002 * @file spi_extra.h 00003 * @brief: Header containing extra types required for SPI interface 00004 ******************************************************************************** 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 #ifndef SPI_EXTRA_H 00014 #define SPI_EXTRA_H 00015 00016 // Platform support needs to be C-compatible to work with other drivers 00017 #ifdef __cplusplus 00018 extern "C" 00019 { 00020 #endif 00021 00022 /******************************************************************************/ 00023 /***************************** Include Files **********************************/ 00024 /******************************************************************************/ 00025 #include <stdio.h> 00026 #include <stdbool.h> 00027 00028 /******************************************************************************/ 00029 /********************** Macros and Constants Definitions **********************/ 00030 /******************************************************************************/ 00031 00032 /******************************************************************************/ 00033 /********************** Variables and User defined data types *****************/ 00034 /******************************************************************************/ 00035 00036 /* 00037 * Note: The structure members are not strongly typed, as this file is included 00038 * in application specific '.c' files. The mbed code structure does not 00039 * allow inclusion of mbed driver files (e.g. mbed.h) into '.c' files. 00040 * All the members are hence typecasted to mbed specific type during 00041 * spi init and read/write operations. 00042 **/ 00043 00044 /** 00045 * @struct mbed_spi_init_param 00046 * @brief Structure holding the SPI init parameters for mbed platform. 00047 */ 00048 typedef struct mbed_spi_init_param { 00049 uint8_t spi_miso_pin; // SPI MISO pin (PinName) 00050 uint8_t spi_mosi_pin; // SPI MOSI pin (PinName) 00051 uint8_t spi_clk_pin; // SPI CLK pin (PinName) 00052 bool use_sw_csb; // Software/Hardware control of CSB pin 00053 } mbed_spi_init_param; 00054 00055 /** 00056 * @struct mbed_spi_desc 00057 * @brief SPI specific descriptor for the mbed platform. 00058 */ 00059 typedef struct mbed_spi_desc { 00060 void *spi_port; // SPI port instance (mbed::SPI) 00061 void *csb_gpio; // SPI chip select gpio instance (DigitalOut) 00062 bool use_sw_csb; // Software/Hardware control of CSB pin 00063 } mbed_spi_desc; 00064 00065 /******************************************************************************/ 00066 /************************ Functions Declarations ******************************/ 00067 /******************************************************************************/ 00068 00069 00070 #ifdef __cplusplus // Closing extern c 00071 } 00072 #endif 00073 00074 #endif /* SPI_EXTRA_H */
Generated on Wed Jul 13 2022 14:37:51 by
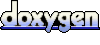