Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
pwm.cpp
00001 /***************************************************************************//** 00002 * @file pwm.cpp 00003 * @brief Implementation of PWM Mbed platform driver interfaces 00004 ******************************************************************************** 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * 00007 * All rights reserved. 00008 * 00009 * This software is proprietary to Analog Devices, Inc. and its licensors. 00010 * By using this software you agree to the terms of the associated 00011 * Analog Devices Software License Agreement. 00012 *******************************************************************************/ 00013 00014 /******************************************************************************/ 00015 /***************************** Include Files **********************************/ 00016 /******************************************************************************/ 00017 00018 #include <stdio.h> 00019 #include <mbed.h> 00020 00021 // Platform drivers needs to be C-compatible to work with other drivers 00022 #ifdef __cplusplus 00023 extern "C" 00024 { 00025 #endif // _cplusplus 00026 00027 #include "error.h" 00028 #include "pwm.h" 00029 #include "gpio.h" 00030 #include "pwm_extra.h" 00031 00032 /******************************************************************************/ 00033 /********************** Macros and Constants Definitions **********************/ 00034 /******************************************************************************/ 00035 00036 /******************************************************************************/ 00037 /********************** Variables and User defined data types *****************/ 00038 /******************************************************************************/ 00039 00040 /******************************************************************************/ 00041 /************************ Functions Declarations ******************************/ 00042 /******************************************************************************/ 00043 00044 /******************************************************************************/ 00045 /************************ Functions Definitions *******************************/ 00046 /******************************************************************************/ 00047 00048 /** 00049 * @brief Initialized the PWM interface 00050 * @param desc[in, out] - Pointer where the configured instance is stored 00051 * @param param[in] - Configuration information for the instance 00052 * @return SUCCESS in case of success, FAILURE otherwise. 00053 */ 00054 int32_t pwm_init(struct pwm_desc **desc, 00055 const struct pwm_init_param *param) 00056 { 00057 struct pwm_desc *new_pwm_desc; 00058 mbed::PwmOut *pwm; 00059 struct mbed_pwm_desc *new_mbed_pwm_desc; 00060 00061 if (!desc || !param) { 00062 return FAILURE; 00063 } 00064 00065 /* Allocate memory for general PWM descriptor */ 00066 new_pwm_desc = (pwm_desc *)malloc(sizeof(pwm_desc)); 00067 if (!new_pwm_desc) { 00068 goto err_new_pwm_desc; 00069 } 00070 00071 new_pwm_desc->id = param->id; // PWM Id 00072 new_pwm_desc->period_ns = param->period_ns; // PWM period 00073 00074 /* Create and initialize Mbed PWM object */ 00075 pwm = new PwmOut((PinName)((mbed_pwm_init_param *)(param->extra))->pwm_pin); 00076 if (!pwm) { 00077 goto err_pwm; 00078 } 00079 00080 /* Allocate memory for Mbed specific PWM descriptor for future use */ 00081 new_mbed_pwm_desc = (mbed_pwm_desc *)malloc(sizeof(mbed_pwm_desc)); 00082 if (!new_mbed_pwm_desc) { 00083 goto err_new_mbed_pwm_desc; 00084 } 00085 00086 new_mbed_pwm_desc->pwm_obj = (mbed::PwmOut *)pwm; 00087 new_pwm_desc->extra = (mbed_pwm_desc *)new_mbed_pwm_desc; 00088 00089 /* Configure Mbed PWM parameters */ 00090 pwm->period_us(param->period_ns / 1000); // Period in usec 00091 pwm->pulsewidth_us(param->duty_cycle_ns / 1000); // Duty cycle in usec 00092 pwm_disable(new_pwm_desc); 00093 00094 *desc = new_pwm_desc; 00095 00096 return SUCCESS; 00097 00098 err_new_mbed_pwm_desc: 00099 free(pwm); 00100 err_pwm: 00101 free(new_pwm_desc); 00102 err_new_pwm_desc: 00103 // Nothing to free 00104 00105 return FAILURE; 00106 } 00107 00108 00109 /** 00110 * @brief Enable the PWM interface 00111 * @param desc[in, out] - Pointer where the configured instance is stored 00112 * @return SUCCESS in case of success, FAILURE otherwise. 00113 */ 00114 int32_t pwm_enable(struct pwm_desc *desc) 00115 { 00116 mbed::PwmOut *pwm; 00117 00118 if (desc) { 00119 pwm = (mbed::PwmOut *)(((mbed_pwm_desc *)desc->extra)->pwm_obj); 00120 if (!pwm) { 00121 return FAILURE; 00122 } 00123 00124 pwm->resume(); 00125 00126 return SUCCESS; 00127 } 00128 00129 return FAILURE; 00130 } 00131 00132 00133 /** 00134 * @brief Disable the PWM interface 00135 * @param desc[in, out] - Pointer where the configured instance is stored 00136 * @return SUCCESS in case of success, FAILURE otherwise. 00137 */ 00138 int32_t pwm_disable(struct pwm_desc *desc) 00139 { 00140 mbed::PwmOut *pwm; 00141 00142 if (desc) { 00143 pwm = (mbed::PwmOut *)(((mbed_pwm_desc *)desc->extra)->pwm_obj); 00144 00145 if (!pwm) { 00146 return FAILURE; 00147 } 00148 00149 pwm->suspend(); 00150 00151 return SUCCESS; 00152 } 00153 00154 return FAILURE; 00155 } 00156 00157 00158 /** 00159 * @brief Remove the memory allocated for PWM device descriptors 00160 * @param desc[in, out] - Pointer where the configured instance is stored 00161 * @return SUCCESS in case of success, FAILURE otherwise. 00162 */ 00163 int32_t pwm_remove(struct pwm_desc *desc) 00164 { 00165 if (!desc) { 00166 return FAILURE; 00167 } 00168 00169 if (((mbed_pwm_desc *)desc->extra)->pwm_obj) { 00170 delete((mbed::PwmOut *)((mbed_pwm_desc *)desc->extra)->pwm_obj); 00171 } 00172 00173 free(desc); 00174 00175 return SUCCESS; 00176 } 00177 00178 00179 #ifdef __cplusplus // Closing extern c 00180 } 00181 #endif // _cplusplus
Generated on Wed Jul 13 2022 14:37:51 by
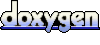