Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
irq_extra.h
00001 /***************************************************************************//** 00002 * @file irq_extra.h 00003 * @brief: Header containing extra types required for IRQ drivers 00004 ******************************************************************************** 00005 * Copyright (c) 2020-2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 #ifndef IRQ_EXTRA_H 00014 #define IRQ_EXTRA_H 00015 00016 00017 // Platform support needs to be C-compatible to work with other drivers 00018 #ifdef __cplusplus 00019 extern "C" 00020 { 00021 #endif 00022 00023 /******************************************************************************/ 00024 /***************************** Include Files **********************************/ 00025 /******************************************************************************/ 00026 00027 #include <stdbool.h> 00028 00029 /******************************************************************************/ 00030 /********************** Macros and Constants Definitions **********************/ 00031 /******************************************************************************/ 00032 00033 /******************************************************************************/ 00034 /*************************** Types Declarations *******************************/ 00035 /******************************************************************************/ 00036 00037 /** 00038 * @enum irq_id 00039 * @brief Interrupts IDs supported by the mbed irq driver 00040 */ 00041 enum irq_id { 00042 /** External interrupt ID1 */ 00043 EXTERNAL_INT_ID1, 00044 /** External interrupt ID2 */ 00045 EXTERNAL_INT_ID2, 00046 /** External interrupt ID3 */ 00047 EXTERNAL_INT_ID3, 00048 /** External interrupt ID4 */ 00049 EXTERNAL_INT_ID4, 00050 /** External interrupt ID5 */ 00051 EXTERNAL_INT_ID5, 00052 /** UART Rx interrupt ID1 */ 00053 UART_RX_INT_ID1, 00054 /** Ticker interrupt ID */ 00055 TICKER_INT_ID, 00056 /* Number of available interrupts */ 00057 NB_INTERRUPTS 00058 }; 00059 00060 /* 00061 * External IRQ events 00062 * */ 00063 typedef enum { 00064 EXT_IRQ_NONE, 00065 EXT_IRQ_RISE, 00066 EXT_IRQ_FALL 00067 } ext_irq_event; 00068 00069 /** 00070 * @struct mbed_irq_init_param 00071 * @brief Structure holding the extra parameters for Interrupt Request. 00072 */ 00073 typedef struct { 00074 uint32_t int_mode; // Interrupt mode (falling/rising etc) 00075 uint32_t ext_int_pin; // External Interrupt pin 00076 uint32_t ticker_period_usec; // Time period in usec for ticker event 00077 /* Application created peripheral object to attach/generate interrupt for (e.g. UART) */ 00078 void *int_obj_type; 00079 } mbed_irq_init_param; 00080 00081 /** 00082 * @struct mbed_irq_desc 00083 * @brief Structure holding the platform descriptor for Interrupt Request. 00084 */ 00085 typedef struct { 00086 uint32_t int_mode; // Interrupt mode (falling/rising etc) 00087 uint32_t ext_int_pin; // External Interrupt pin 00088 uint32_t ticker_period_usec; // Time period in usec for ticker event 00089 void *int_obj; // Interrupt specific object (e.g. InterruptIn, Ticker) 00090 } mbed_irq_desc; 00091 00092 #ifdef __cplusplus // Closing extern c 00093 } 00094 #endif 00095 00096 #endif // IRQ_EXTRA_H_
Generated on Wed Jul 13 2022 14:37:51 by
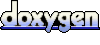