Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
i2c.cpp
00001 /***************************************************************************//** 00002 * @file i2c.cpp 00003 * @brief Implementation of I2C Mbed platform driver interfaces 00004 ******************************************************************************** 00005 * Copyright (c) 2019 - 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 /******************************************************************************/ 00014 /***************************** Include Files **********************************/ 00015 /******************************************************************************/ 00016 00017 #include <stdio.h> 00018 #include <mbed.h> 00019 00020 // Platform drivers needs to be C-compatible to work with other drivers 00021 #ifdef __cplusplus 00022 extern "C" 00023 { 00024 #endif // _cplusplus 00025 00026 #include "error.h" 00027 #include "i2c.h " 00028 #include "i2c_extra.h" 00029 00030 /******************************************************************************/ 00031 /********************** Macros and Constants Definitions **********************/ 00032 /******************************************************************************/ 00033 00034 /******************************************************************************/ 00035 /************************ Functions Declarations ******************************/ 00036 /******************************************************************************/ 00037 00038 /******************************************************************************/ 00039 /************************ Functions Definitions *******************************/ 00040 /******************************************************************************/ 00041 00042 /** 00043 * @brief Initialize the I2C communication peripheral. 00044 * @param desc - The I2C descriptor. 00045 * @param param - The structure that contains the I2C parameters. 00046 * @return SUCCESS in case of success, FAILURE otherwise. 00047 */ 00048 int32_t i2c_init_noos(struct i2c_desc **desc, 00049 const struct i2c_init_param *param) 00050 { 00051 mbed::I2C *i2c; // pointer to new I2C instance 00052 mbed_i2c_desc *mbed_desc; // pointer to mbed i2c desc 00053 i2c_desc *new_desc; 00054 00055 if (desc) { 00056 // Create an i2c descriptor object for the device 00057 new_desc = (i2c_desc *)malloc(sizeof(i2c_desc)); 00058 if (!new_desc) { 00059 goto err_new_desc; 00060 } 00061 00062 // Address passed in parameter shifted left by 1 to form 00063 // 7-bit i2c slave address (7 MSBs) and the LSB acts as 00064 // r/w bit during i2c read/write operations 00065 new_desc->slave_address = ((param->slave_address) << 1); 00066 00067 // Configure and instantiate I2C protocol 00068 i2c = new I2C( 00069 (PinName)(((mbed_i2c_init_param *)param->extra)->i2c_sda_pin), 00070 (PinName)(((mbed_i2c_init_param *)param->extra)->i2c_scl_pin)); 00071 00072 if (!i2c) { 00073 goto err_i2c; 00074 } 00075 00076 // Create the i2c mbed descriptor object to store new i2c instance 00077 mbed_desc = (mbed_i2c_desc *)malloc(sizeof(mbed_i2c_desc)); 00078 if (!mbed_desc) { 00079 goto err_mbed_desc; 00080 } 00081 00082 mbed_desc->i2c_port = (I2C *)i2c; 00083 new_desc->extra = (mbed_i2c_desc *)mbed_desc; 00084 00085 *desc = new_desc; 00086 00087 return SUCCESS; 00088 } 00089 00090 err_mbed_desc: 00091 free(i2c); 00092 err_i2c: 00093 free(new_desc); 00094 err_new_desc: 00095 // Nothing to free 00096 00097 return FAILURE; 00098 } 00099 00100 00101 /** 00102 * @brief Free the resources allocated by i2c_init_noos(). 00103 * @param desc - The I2C descriptor. 00104 * @return SUCCESS in case of success, FAILURE otherwise. 00105 */ 00106 int32_t i2c_remove(struct i2c_desc *desc) 00107 { 00108 if (desc) { 00109 // Free the I2C port object 00110 if ((I2C *)(((mbed_i2c_desc *)(desc->extra))->i2c_port)) { 00111 delete((I2C *)(((mbed_i2c_desc *)(desc->extra))->i2c_port)); 00112 } 00113 00114 // Free the I2C extra descriptor object 00115 if ((mbed_i2c_desc *)(desc->extra)) { 00116 free((mbed_i2c_desc *)(desc->extra)); 00117 } 00118 00119 // Free the I2C descriptor object 00120 free(desc); 00121 00122 return SUCCESS; 00123 } 00124 00125 return FAILURE; 00126 } 00127 00128 00129 /** 00130 * @brief Write data to a slave device. 00131 * @param desc - The I2C descriptor. 00132 * @param data - Buffer that stores the transmission data. 00133 * @param bytes_number - Number of bytes to write. 00134 * @param stop_bit - Stop condition control. 00135 * Example: 0 - A stop condition will not be generated; 00136 * 1 - A stop condition will be generated. 00137 * @return SUCCESS in case of success, FAILURE otherwise. 00138 */ 00139 00140 int32_t i2c_write_noos(struct i2c_desc *desc, 00141 uint8_t *data, 00142 uint8_t bytes_number, 00143 uint8_t stop_bit) 00144 { 00145 mbed::I2C *i2c; 00146 i2c = (I2C *)(((mbed_i2c_desc *)(desc->extra))->i2c_port); 00147 00148 /** 00149 The MBED I2C API is reversed for parameter 4 00150 Instead of stop_bit - it has 00151 @param repeated - Repeated start, true - don't send stop at end default value is false. 00152 Inverting here to keep the no-OS/platform_drivers API 00153 */ 00154 if (!(i2c->write(desc->slave_address, (char *)data, bytes_number, !stop_bit))) { 00155 return SUCCESS; 00156 } else { 00157 return FAILURE; 00158 } 00159 } 00160 00161 00162 /** 00163 * @brief Read data from a slave device. 00164 * @param desc - The I2C descriptor. 00165 * @param data - Buffer that will store the received data. 00166 * @param bytes_number - Number of bytes to read. 00167 * @param stop_bit - Stop condition control. 00168 * Example: 0 - A stop condition will not be generated; 00169 * 1 - A stop condition will be generated. 00170 * @return SUCCESS in case of success, FAILURE otherwise. 00171 */ 00172 int32_t i2c_read_noos(struct i2c_desc *desc, 00173 uint8_t *data, 00174 uint8_t bytes_number, 00175 uint8_t stop_bit) 00176 { 00177 mbed::I2C *i2c; 00178 i2c = (I2C *)(((mbed_i2c_desc *)(desc->extra))->i2c_port); 00179 00180 /** 00181 The MBED I2C API is reversed for parameter 4 00182 Instead of stop_bit - it has 00183 @param repeated - Repeated start, true - don't send stop at end default value is false. 00184 Inverting here to keep the no-OS/platform_drivers API 00185 */ 00186 if (!(i2c->read(desc->slave_address, (char *)data, bytes_number, !stop_bit))) { 00187 return SUCCESS; 00188 } else { 00189 return FAILURE; 00190 } 00191 } 00192 00193 #ifdef __cplusplus 00194 } 00195 #endif // _cplusplus
Generated on Wed Jul 13 2022 14:37:51 by
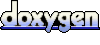