Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
aout.h
00001 /***************************************************************************//** 00002 * @file aout.h 00003 * @author PMallick (Pratyush.Mallick@analog.com) 00004 ******************************************************************************** 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 #ifndef AOUT_H 00014 #define AOUT_H 00015 00016 /******************************************************************************/ 00017 /***************************** Include Files **********************************/ 00018 /******************************************************************************/ 00019 00020 #include <stdint.h> 00021 00022 /******************************************************************************/ 00023 /********************** Macros and Constants Definitions **********************/ 00024 /******************************************************************************/ 00025 00026 /******************************************************************************/ 00027 /*************************** Types Declarations *******************************/ 00028 /******************************************************************************/ 00029 /** 00030 * @struct aout_init_param 00031 * @brief Structure holding the parameters for analog output initialization 00032 */ 00033 struct aout_init_param { 00034 /* Analog output pin number */ 00035 int32_t number; 00036 /* Min output range of DAC in volts */ 00037 float aout_min_v; 00038 /* Max output range of DAC in volts */ 00039 float aout_max_v; 00040 /* Analog output reference voltage */ 00041 float vref; 00042 /* Analog output platform specific functions */ 00043 const struct aout_platform_ops *platform_ops; 00044 }; 00045 00046 /** 00047 * @struct aout_desc 00048 * @brief Structure holding analog output descriptor 00049 */ 00050 struct aout_desc { 00051 /* Analog output pin number */ 00052 int32_t number; 00053 /* Min output value of DAC in volts */ 00054 float aout_min_v; 00055 /* Max output value of DAC in volts */ 00056 float aout_max_v; 00057 /* Analog output reference voltage */ 00058 float vref; 00059 /* Analog output platform specific functions */ 00060 const struct aout_platform_ops *platform_ops; 00061 /* Analog extra parameters (device specific) */ 00062 void *extra; 00063 }; 00064 00065 /** 00066 * @struct aout_platform_ops 00067 * @brief Structure holding analog output function pointers that 00068 * point to the platform specific function 00069 */ 00070 struct aout_platform_ops { 00071 /** Analog output initialization function pointer */ 00072 int32_t(*init)(struct aout_desc **, const struct aout_init_param *); 00073 /** Analog output write function pointer */ 00074 int32_t(*write)(struct aout_desc *, float); 00075 /** Analog output remove function pointer */ 00076 int32_t(*remove)(struct aout_desc *); 00077 }; 00078 00079 /******************************************************************************/ 00080 /************************ Functions Declarations ******************************/ 00081 /******************************************************************************/ 00082 /* Write analog output voltage */ 00083 int32_t aout_set_voltage(struct aout_desc *desc, float value); 00084 00085 /* Initialize the analog output pin */ 00086 int32_t aout_init(struct aout_desc **desc, 00087 const struct aout_init_param *param); 00088 00089 /* Free the resources allocated by analog_out_init() */ 00090 int32_t aout_remove(struct aout_desc *desc); 00091 00092 #endif
Generated on Wed Jul 13 2022 14:37:51 by
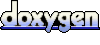