Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
aout.cpp
00001 /***************************************************************************//** 00002 * @file aout.cpp 00003 * @brief Implementation of mbed specific analog output functionality 00004 ******************************************************************************** 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 /******************************************************************************/ 00014 /***************************** Include Files **********************************/ 00015 /******************************************************************************/ 00016 #include <mbed.h> 00017 00018 // Platform drivers needs to be C-compatible to work with other drivers 00019 #ifdef __cplusplus 00020 extern "C" 00021 { 00022 #endif // _cplusplus 00023 #include "aout.h " 00024 #include "mbed_ain_aout_extra.h" 00025 #include "error.h" 00026 00027 /******************************************************************************/ 00028 /********************** Variables and User defined data types *****************/ 00029 /******************************************************************************/ 00030 00031 /******************************************************************************/ 00032 /************************ Functions Declarations ******************************/ 00033 /******************************************************************************/ 00034 00035 /******************************************************************************/ 00036 /************************ Functions Definitions *******************************/ 00037 /******************************************************************************/ 00038 /** 00039 * @brief Write voltage to analog output pin 00040 * @param desc[in] - Analog output descriptor 00041 * @param value[in] - Analog output value in volts 00042 * @return SUCCESS in case of success, FAILURE otherwise. 00043 */ 00044 int32_t aout_set_voltage(struct aout_desc *desc, float value) 00045 { 00046 /* Pointer to analog output object */ 00047 mbed::AnalogOut *analog_output; 00048 float volt_mapped; 00049 00050 /* Mbed AnalogOut APIs accept voltage in range from 0.0f 00051 * (representing DAC min output range / 0%) to 1.0f (representing 00052 * DAC max output range / 100%). Hence we need to map the voltage levels 00053 * before passing it to mbed APIs */ 00054 if (value >= desc->aout_min_v && value <= desc->aout_max_v) { 00055 volt_mapped = ((value - desc->aout_min_v) / 00056 (desc->aout_max_v - desc->aout_min_v)); 00057 } else { 00058 return -EINVAL; 00059 } 00060 00061 if (desc && desc->extra) { 00062 analog_output = (AnalogOut *)((mbed_analog_out_desc *) 00063 desc->extra)->analog_out_obj ; 00064 analog_output->write(volt_mapped); 00065 00066 return SUCCESS; 00067 } 00068 return FAILURE; 00069 } 00070 00071 /** 00072 * @brief Initialize the analog output pin 00073 * @param desc[in, out] - Analog output descriptor structure 00074 * @param param[in] - Structure that contains analog output 00075 * initialization parameters 00076 * @return SUCCESS in case of success, FAILURE otherwise 00077 */ 00078 int32_t aout_init(struct aout_desc **desc, 00079 const struct aout_init_param *param) 00080 { 00081 mbed::AnalogOut *analog_output; 00082 struct aout_desc *new_analog_out_desc; 00083 struct mbed_analog_out_desc *new_mbed_analog_out_desc; 00084 00085 if (!desc || !param ) { 00086 return FAILURE; 00087 } 00088 00089 /* Allocate memory for general analog output descriptor */ 00090 new_analog_out_desc = (aout_desc *)malloc(sizeof(aout_desc)); 00091 if (!new_analog_out_desc) { 00092 goto err_new_out_desc; 00093 } 00094 00095 /* Configure pin number,DAC max and min range */ 00096 new_analog_out_desc->number = param->number; 00097 new_analog_out_desc->aout_min_v = param->aout_min_v; 00098 new_analog_out_desc->aout_max_v = param->aout_max_v; 00099 00100 /* Allocate memory for mbed specific analog output descriptor 00101 * for future use */ 00102 new_mbed_analog_out_desc = (mbed_analog_out_desc *)malloc 00103 (sizeof(new_mbed_analog_out_desc)); 00104 if (!new_mbed_analog_out_desc) { 00105 goto err_mbed_analog_out_desc; 00106 } 00107 00108 /* Create and initialize mbed analog output object */ 00109 analog_output = new AnalogOut((PinName)param->number); 00110 if (analog_output) { 00111 new_mbed_analog_out_desc->analog_out_obj = analog_output; 00112 } else { 00113 goto err_analog_out_instance; 00114 } 00115 00116 new_analog_out_desc->extra = (mbed_analog_out_desc *) 00117 new_mbed_analog_out_desc; 00118 00119 *desc = new_analog_out_desc; 00120 return SUCCESS; 00121 00122 err_analog_out_instance: 00123 free(new_mbed_analog_out_desc); 00124 err_mbed_analog_out_desc: 00125 free(new_analog_out_desc); 00126 err_new_out_desc: 00127 // Nothing to free 00128 00129 return FAILURE; 00130 } 00131 00132 /** 00133 * @brief Deallocate resources allocated by aout_init() 00134 * @param desc[in, out] - Analog output descriptor 00135 * @return SUCCESS in case of success, FAILURE otherwise. 00136 */ 00137 int32_t aout_remove(struct aout_desc *desc) 00138 { 00139 if (desc) { 00140 00141 if (((mbed_analog_out_desc *)desc->extra)->analog_out_obj) { 00142 delete((mbed::AnalogIn *)((mbed_analog_out_desc *)desc-> 00143 extra)->analog_out_obj); 00144 } 00145 00146 if ((mbed_analog_out_desc *)desc->extra) { 00147 free((mbed_analog_out_desc *)desc->extra); 00148 } 00149 00150 if (((mbed_analog_out_desc *)desc->extra)->analog_out_obj) { 00151 delete((mbed::AnalogOut *)((mbed_analog_out_desc *) 00152 desc->extra)->analog_out_obj); 00153 } 00154 00155 if ((mbed_analog_out_desc *)desc->extra) { 00156 free((mbed_analog_out_desc *)desc->extra); 00157 } 00158 00159 free(desc); 00160 00161 return SUCCESS; 00162 } 00163 00164 return FAILURE; 00165 } 00166 00167 /** 00168 * @brief Mbed specific analog output platform ops structure 00169 */ 00170 const struct aout_platform_ops mbed_aout_ops = { 00171 .init = &aout_init, 00172 .write = &aout_set_voltage, 00173 .remove = &aout_remove 00174 }; 00175 00176 00177 // Platform drivers needs to be C-compatible to work with other drivers 00178 #ifdef __cplusplus 00179 } 00180 #endif // _cplusplus
Generated on Wed Jul 13 2022 14:37:51 by
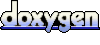