Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
ain.cpp
00001 /***************************************************************************//** 00002 * @file ain.cpp 00003 * @brief Implementation of mbed specific analog input functionality 00004 ******************************************************************************** 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 /******************************************************************************/ 00014 /***************************** Include Files **********************************/ 00015 /******************************************************************************/ 00016 #include <mbed.h> 00017 00018 // Platform drivers needs to be C-compatible to work with other drivers 00019 #ifdef __cplusplus 00020 extern "C" 00021 { 00022 #endif // _cplusplus 00023 #include "ain.h " 00024 #include "mbed_ain_aout_extra.h" 00025 #include "error.h" 00026 00027 /******************************************************************************/ 00028 /********************** Variables and User defined data types *****************/ 00029 /******************************************************************************/ 00030 00031 /******************************************************************************/ 00032 /************************ Functions Declarations ******************************/ 00033 /******************************************************************************/ 00034 00035 /******************************************************************************/ 00036 /************************ Functions Definitions *******************************/ 00037 /******************************************************************************/ 00038 /** 00039 * @brief Read voltage from analog input pin 00040 * @param desc[in] - Analog input descriptor 00041 * @param value[out] - Buffer to pass analog reading. 00042 * @return SUCCESS in case of success, FAILURE otherwise 00043 */ 00044 int32_t ain_get_voltage(struct ain_desc *desc, float *value) 00045 { 00046 /* Pointer to analog input object */ 00047 mbed::AnalogIn *analog_input; 00048 00049 if (desc && desc->extra) { 00050 analog_input = (AnalogIn *)((mbed_analog_in_desc *) 00051 desc->extra)->analog_in_obj; 00052 *value = analog_input->read_voltage(); 00053 00054 return SUCCESS; 00055 } 00056 return FAILURE; 00057 } 00058 00059 /** 00060 * @brief Initialize the Analog Input Pin 00061 * @param desc[in, out] - Analog input descriptor structure 00062 * @param param[in] - Structure that contains analog input 00063 * initialization parameters 00064 * @return SUCCESS in case of success, FAILURE otherwise 00065 */ 00066 int32_t ain_init(struct ain_desc **desc, 00067 const struct ain_init_param *param) 00068 { 00069 mbed::AnalogIn *analog_input; 00070 struct ain_desc *new_analog_in_desc; 00071 struct mbed_analog_in_desc *new_mbed_analog_in_desc; 00072 00073 if (!desc || !param ) { 00074 return FAILURE; 00075 } 00076 00077 /* Allocate memory for general analog input descriptor */ 00078 new_analog_in_desc = (ain_desc *)malloc(sizeof(ain_desc)); 00079 if (!new_analog_in_desc) { 00080 goto err_new_in_desc; 00081 } 00082 00083 /* Configure pin number and reference voltage*/ 00084 new_analog_in_desc->number = param->number; 00085 new_analog_in_desc->vref = param->vref; 00086 00087 /* Allocate memory for mbed specific analog input descriptor 00088 * for future use */ 00089 new_mbed_analog_in_desc = (mbed_analog_in_desc *)malloc(sizeof( 00090 mbed_analog_in_desc)); 00091 if (!new_mbed_analog_in_desc) { 00092 goto err_mbed_analog_in_desc; 00093 } 00094 00095 /* Create and initialize mbed analog input object */ 00096 analog_input = new AnalogIn((PinName)param->number, param->vref); 00097 if (analog_input) { 00098 new_mbed_analog_in_desc->analog_in_obj = analog_input; 00099 } 00100 00101 else { 00102 goto err_analog_in_instance; 00103 } 00104 00105 new_analog_in_desc->extra = (mbed_analog_in_desc *)new_mbed_analog_in_desc; 00106 00107 *desc = new_analog_in_desc; 00108 return SUCCESS; 00109 00110 err_analog_in_instance: 00111 free(new_mbed_analog_in_desc); 00112 err_mbed_analog_in_desc: 00113 free(new_analog_in_desc); 00114 err_new_in_desc: 00115 // Nothing to free 00116 00117 return FAILURE; 00118 } 00119 00120 /** 00121 * @brief Deallocate resources allocated by ain_init() 00122 * @param desc[in, out] - Analog input descriptor 00123 * @return SUCCESS in case of success, FAILURE otherwise. 00124 */ 00125 int32_t ain_remove(struct ain_desc *desc) 00126 { 00127 if (desc) { 00128 00129 if (((mbed_analog_in_desc *)desc->extra)->analog_in_obj) { 00130 delete((mbed::AnalogIn *)((mbed_analog_in_desc *)desc-> 00131 extra)->analog_in_obj); 00132 } 00133 00134 if ((mbed_analog_in_desc *)desc->extra) { 00135 free((mbed_analog_in_desc *)desc->extra); 00136 } 00137 00138 if (((mbed_analog_in_desc *)desc->extra)->analog_in_obj) { 00139 delete((mbed::AnalogOut *)((mbed_analog_in_desc *) 00140 desc->extra)->analog_in_obj); 00141 } 00142 00143 if ((mbed_analog_in_desc *)desc->extra) { 00144 free((mbed_analog_in_desc *)desc->extra); 00145 } 00146 00147 free(desc); 00148 00149 return SUCCESS; 00150 } 00151 00152 return FAILURE; 00153 } 00154 00155 /** 00156 * @brief Mbed specific analog input platform ops structure 00157 */ 00158 const struct ain_platform_ops mbed_ain_ops = { 00159 .init = &ain_init, 00160 .read = &ain_get_voltage, 00161 .remove = &ain_remove 00162 }; 00163 00164 // Platform drivers needs to be C-compatible to work with other drivers 00165 #ifdef __cplusplus 00166 } 00167 #endif // _cplusplus
Generated on Wed Jul 13 2022 14:37:51 by
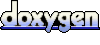