Platform drivers for Mbed.
Dependents: EVAL-CN0535-FMCZ EVAL-CN0535-FMCZ EVAL-AD568x-AD569x EVAL-AD7606 ... more
adc_data_capture.h
00001 /***************************************************************************//** 00002 * @file adc_data_capture.h 00003 * @brief Header for ADC data capture interfaces 00004 ******************************************************************************** 00005 * Copyright (c) 2021 Analog Devices, Inc. 00006 * All rights reserved. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 #ifndef _ADC_DATA_CAPTURE_H_ 00014 #define _ADC_DATA_CAPTURE_H_ 00015 00016 /******************************************************************************/ 00017 /***************************** Include Files **********************************/ 00018 /******************************************************************************/ 00019 00020 #include <stdint.h> 00021 #include <stdbool.h> 00022 #include <stddef.h> 00023 00024 /******************************************************************************/ 00025 /********************** Macros and Constants Definition ***********************/ 00026 /******************************************************************************/ 00027 00028 /******************************************************************************/ 00029 /********************** Variables and User Defined Data Types *****************/ 00030 /******************************************************************************/ 00031 00032 /** 00033 * @struct data_capture_ops 00034 * @brief Structure holding function pointers that points to device specific 00035 * data capture functions 00036 */ 00037 struct data_capture_ops { 00038 /* Perform operations required before single sample read */ 00039 int32_t(*single_sample_read_start_ops)(uint8_t input_chn); 00040 00041 /* Wait for conversion to finish on enabled channels and read conversion data */ 00042 int32_t(*perform_conv_and_read_sample)(uint32_t *read_data, uint8_t chn); 00043 00044 /* Enable operations required post single sample read */ 00045 int32_t(*single_sample_read_stop_ops)(uint8_t input_chn); 00046 00047 /* Perform operations required before continuous sample read */ 00048 int32_t(*continuous_sample_read_start_ops)(uint32_t chn_mask); 00049 00050 /* Read ADC raw sample/data */ 00051 int32_t(*read_converted_sample)(uint32_t *read_data, uint8_t input_chn); 00052 00053 /* Perform operations required post continuous sample read */ 00054 int32_t(*continuous_sample_read_stop_ops)(void); 00055 00056 /* Trigger next data conversion */ 00057 int32_t(*trigger_next_conversion)(void); 00058 }; 00059 00060 /******************************************************************************/ 00061 /************************ Public Declarations *********************************/ 00062 /******************************************************************************/ 00063 00064 int32_t read_single_sample(uint8_t input_chn, uint32_t *raw_data); 00065 size_t read_buffered_data(char *pbuf, 00066 size_t bytes, 00067 size_t offset, 00068 uint32_t active_chns_mask, 00069 uint8_t sample_size_in_bytes); 00070 void store_requested_samples_count(size_t bytes, uint8_t sample_size_in_bytes); 00071 void start_data_capture(uint32_t ch_mask, uint8_t num_of_chns); 00072 void stop_data_capture(void); 00073 void data_capture_callback(void *ctx, uint32_t event, void *extra); 00074 00075 #endif /* _ADC_DATA_CAPTURE_H_ */
Generated on Wed Jul 13 2022 14:37:51 by
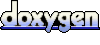