
Example program for SDP-K1 SDP connector peripherals.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /***************************************************************************** 00002 * Copyright (c) 2019 Analog Devices, Inc. 00003 * 00004 * All rights reserved. 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions are met: 00008 * - Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * - Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * - Modified versions of the software must be conspicuously marked as such. 00014 * - This software is licensed solely and exclusively for use with 00015 * processors/products manufactured by or for Analog Devices, Inc. 00016 * - This software may not be combined or merged with other code in any manner 00017 * that would cause the software to become subject to terms and 00018 * conditions which differ from those listed here. 00019 * - Neither the name of Analog Devices, Inc. nor the names of its 00020 * contributors may be used to endorse or promote products derived 00021 * from this software without specific prior written permission. 00022 * - The use of this software may or may not infringe the patent rights 00023 * of one or more patent holders. This license does not release you from 00024 * the requirement that you obtain separate licenses from these patent 00025 * holders to use this software. 00026 * 00027 * THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES, INC. AND CONTRIBUTORS "AS IS" 00028 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, 00029 * NON-INFRINGEMENT, TITLE, MERCHANTABILITY AND FITNESS FOR A 00030 * PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL ANALOG DEVICES, 00031 * INC. OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00032 * SPECIAL, EXEMPLARY, PUNITIVE OR CONSEQUENTIAL DAMAGES 00033 * (INCLUDING, BUT NOT LIMITED TO, DAMAGES ARISING OUT OF CLAIMS OF 00034 * INTELLECTUAL PROPERTY RIGHTS INFRINGEMENT; PROCUREMENT OF SUBSTITUTE 00035 * GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00036 * HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, 00037 * STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00038 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 00039 * POSSIBILITY OF SUCH DAMAGE. 00040 * 00041 * 20180927-7CBSD SLA 00042 *****************************************************************************/ 00043 00044 /* 00045 The SDP connector is a 120 pin connector used on Analog Devices controller 00046 boards to interface with ADI evaluation boards, over 450 ADI evaluation boards 00047 utilise this connector, a list of these boards can be found at 00048 https://www.analog.com/en/design-center/evaluation-hardware-and-software/evaluation-development-platforms/sdp.html#Compatible-Boards. 00049 00050 The following is an example program showing how to initialise and use SDP 00051 connector peripherals (using SPI and GPIOs as an example) on the SDP-K1 in mbed. 00052 Informtion on the pin name definitions for the SDP connector on the SDP-K1 can 00053 be found at https://os.mbed.com/platforms/SDP_K1/. 00054 The full list of peripherals available on the SDP connector include GPIOs, 00055 Timers, SPI, I2C, and UART. For further information on how to enable these 00056 peripherals, consult the mbed documentation https://os.mbed.com/docs/mbed-os/v5.15/apis/drivers.html 00057 */ 00058 #include "mbed.h" 00059 #include "platform/mbed_thread.h" 00060 00061 // Blinking rate in milliseconds 00062 #define SLEEP_TIME 500 00063 00064 // Initialise the digital pin LED1 as an output 00065 DigitalOut led1(LED1); 00066 00067 // Initialise the serial object with TX and RX pins 00068 Serial pc(USBTX, USBRX); 00069 00070 // Initialise the SDP connector SPI peripheral (mosi, miso, clk) 00071 SPI spi(SDP_SPI_MOSI, SDP_SPI_MISO, SDP_SPI_SCK); // SDP coonector pin no. (84, 83, 82) 00072 00073 // SDP SPI CS A (one of 3 available chip selects on the SDP connector) 00074 DigitalOut cs_a(SDP_SPI_CS_A); // SDP connector pin no. 85 00075 00076 // Initialise GPIOs, there are 8 available SDP connector GPIO's (GPIO 0-7) 00077 DigitalOut gpio1(SDP_GPIO_1); // GPIO pin 1 - SDP connector pin no. 78 00078 DigitalOut gpio2(SDP_GPIO_2); // GPIO pin 2 - SDP connector pin no. 44 00079 00080 // main() runs in its own thread in the OS 00081 int main() 00082 { 00083 00084 uint8_t receive; 00085 00086 // Default chip select high 00087 cs_a = 1; 00088 00089 // Setting GPIOs low initially 00090 gpio1 = 0; 00091 gpio2 = 0; 00092 00093 while(true){ 00094 00095 // Toggling GPIOs 00096 gpio1 = !gpio1; 00097 00098 gpio2 = !gpio2; 00099 00100 // Set CS low for SPI write 00101 cs_a = 0; 00102 // Performing SPI write/read (loopback if MOSI and MISO lines are connected) 00103 receive = spi.write(0x0A); 00104 // Set CS high after performing SPI write 00105 cs_a = 1; 00106 00107 // Toggling GPIOs 00108 gpio1 = !gpio1; 00109 00110 gpio2 = !gpio2; 00111 00112 // Blink LED and wait 500 ms 00113 led1 = !led1; 00114 thread_sleep_for(SLEEP_TIME); 00115 00116 } 00117 00118 }
Generated on Wed Jul 13 2022 07:29:17 by
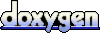