
Program files for Example program for EVAL-AD7768-1
Dependencies: platform_drivers
ad77681_iio.c
00001 /***************************************************************************//** 00002 * @file ad77681_iio.c 00003 * @brief Implementation of AD7768-1 IIO application interfaces 00004 * @details This module acts as an interface for AD7768-1 IIO application 00005 ******************************************************************************** 00006 * Copyright (c) 2021 Analog Devices, Inc. 00007 * All rights reserved. 00008 * 00009 * This software is proprietary to Analog Devices, Inc. and its licensors. 00010 * By using this software you agree to the terms of the associated 00011 * Analog Devices Software License Agreement. 00012 *******************************************************************************/ 00013 00014 /******************************************************************************/ 00015 /***************************** Include Files **********************************/ 00016 /******************************************************************************/ 00017 00018 #include <stdint.h> 00019 #include <string.h> 00020 #include <stdio.h> 00021 #include <errno.h> 00022 00023 #include "app_config.h" 00024 #include "tinyiiod.h" 00025 #include "ad77681_iio.h" 00026 #include "ad77681_user_config.h" 00027 #include "ad77681_regs.h" 00028 #include "ad77681_data_capture.h" 00029 #include "adc_data_capture.h" 00030 #include "error.h" 00031 00032 /******************************************************************************/ 00033 /************************ Macros/Constants ************************************/ 00034 /******************************************************************************/ 00035 00036 /* AD77681 Channel Number */ 00037 #define AD77681_NUM_CHANNELS 1 00038 /* Bytes per sample (for ADC resolution of 24-bits) */ 00039 #define AD77681_BYTES_PER_SAMPLE sizeof(uint32_t) 00040 /* Number of data storage bits (needed for IIO client) */ 00041 #define AD77681_CHN_STORAGE_BITS (AD77681_BYTES_PER_SAMPLE * 8) 00042 /* AD77681 24 bits scale factor */ 00043 #define AD77681_SCALE_FACTOR (1 << ADC_RESOLUTION) 00044 /* AD77681 ADC data to Voltage conversion default scale factor for IIO client */ 00045 #define AD77681_DEFAULT_SCALE ((((float)(AD77681_VOLTAGE_REF / 1000.00) * 2) / AD77681_SCALE_FACTOR) * 1000) 00046 /* Register Max Address */ 00047 #define AD77681_REG_MAX_ADDR AD77681_REG_MCLK_COUNTER + 1 00048 /* Conv Mode Value after setting a single conversion mode */ 00049 #define SINGLE_MODE_CONV_STANDBY 6 00050 /* Conv Mode Value after setting a periodic conversion mode */ 00051 #define PERIODIC_MODE_CONV_STANDBY 7 00052 00053 /******************************************************************************/ 00054 /*************************** Types Declarations *******************************/ 00055 /******************************************************************************/ 00056 00057 /* IIO interface descriptor */ 00058 static struct iio_desc *p_ad77681_iio_desc; 00059 00060 /* Device name */ 00061 static const char dev_name[] = ACTIVE_DEVICE_NAME; 00062 00063 /* Pointer to the struct representing the AD77681 IIO device */ 00064 struct ad77681_dev *p_ad77681_dev_inst = NULL; 00065 00066 /* Pointer to the struct AD77681 status register */ 00067 struct ad77681_status_registers *p_ad77681_stat_reg = NULL; 00068 00069 /* Flag to trigger new data capture */ 00070 static bool adc_data_capture_started = false; 00071 00072 /* Scale value per channel */ 00073 static float attr_scale_val[AD77681_NUM_CHANNELS] = { 00074 AD77681_DEFAULT_SCALE 00075 }; 00076 00077 /* Power mode values string representation */ 00078 static const char* power_mode_str[] = { 00079 "Eco-Mode", 00080 "Value-Not-Assigned", 00081 "Median-Mode", 00082 "Fast-Mode" 00083 }; 00084 00085 /* Conversion mode values string representation */ 00086 static const char* conv_mode_str[] = { 00087 "Continuous-Mode", 00088 "Continious-One-Shot-Mode", 00089 "Single-Mode", 00090 "Periodic-Mode", 00091 "Standby-Mode" 00092 }; 00093 00094 /* MCLK division values string representation */ 00095 static const char* mclk_division_str[] = { 00096 "AD77681_MCLK_DIV_16", 00097 "AD77681_MCLK_DIV_8", 00098 "AD77681_MCLK_DIV_4", 00099 "AD77681_MCLK_DIV_2" 00100 }; 00101 00102 /******************************************************************************/ 00103 /************************ Functions Prototypes ********************************/ 00104 /******************************************************************************/ 00105 00106 /******************************************************************************/ 00107 /************************ Functions Definitions *******************************/ 00108 /******************************************************************************/ 00109 00110 /*! 00111 * @brief Getter/Setter for the sampling frequency attribute value 00112 * @param device- pointer to IIO device structure 00113 * @param buf- pointer to buffer holding attribute value 00114 * @param len- length of buffer string data 00115 * @param channel- pointer to IIO channel structure 00116 * @param id- Attribute ID (optional) 00117 * @return Number of characters read/written 00118 * @Note This attribute is used to define the timeout period in IIO 00119 * client during data capture. 00120 * Timeout = (number of requested samples * (1/sampling frequency)) + 1sec 00121 * e.g. if sampling frequency = 64KSPS and requested samples = 400 00122 * Timeout = (400 * (1/64000)) + 1 = 1.00625sec = ~1sec 00123 */ 00124 static ssize_t get_sampling_frequency(void *device, 00125 char *buf, 00126 size_t len, 00127 const struct iio_ch_info *channel, 00128 intptr_t id) 00129 { 00130 return (ssize_t) sprintf(buf, "%d", (int32_t)AD77681_DEFAULT_SAMPLING_FREQ); 00131 } 00132 00133 static ssize_t set_sampling_frequency(void *device, 00134 char *buf, 00135 size_t len, 00136 const struct iio_ch_info *channel, 00137 intptr_t id) 00138 { 00139 /* NA- Can't set sampling frequency value */ 00140 return len; 00141 } 00142 00143 /*! 00144 * @brief Getter/Setter for the raw attribute value 00145 * @param device- pointer to IIO device structure 00146 * @param buf- pointer to buffer holding attribute value 00147 * @param len- length of buffer string data 00148 * @param channel- pointer to IIO channel structure 00149 * @return Number of characters read/written 00150 */ 00151 ssize_t get_raw(void *device, 00152 char *buf, 00153 size_t len, 00154 const struct iio_ch_info *channel, 00155 intptr_t id) 00156 { 00157 uint32_t adc_data_raw = 0; 00158 00159 /* Capture the raw adc data */ 00160 if (read_single_sample((uint32_t)channel->ch_num, &adc_data_raw) != FAILURE) { 00161 return (ssize_t) sprintf(buf, "%d", (int32_t)adc_data_raw); 00162 } 00163 00164 return - EINVAL; 00165 } 00166 00167 ssize_t set_raw(void *device, 00168 char *buf, 00169 size_t len, 00170 const struct iio_ch_info *channel, 00171 intptr_t id) 00172 { 00173 /* NA- Can't set raw value */ 00174 return len; 00175 } 00176 00177 /*! 00178 * @brief Getter/Setter for the scale attribute value 00179 * @param device- pointer to IIO device structure 00180 * @param buf- pointer to buffer holding attribute value 00181 * @param len- length of buffer string data 00182 * @param channel- pointer to IIO channel structure 00183 * @return Number of characters read/written 00184 */ 00185 ssize_t get_scale(void* device, 00186 char* buf, 00187 size_t len, 00188 const struct iio_ch_info* channel, 00189 intptr_t id) 00190 { 00191 return (ssize_t)sprintf(buf, "%f", attr_scale_val[channel->ch_num]); 00192 } 00193 00194 ssize_t set_scale(void* device, 00195 char* buf, 00196 size_t len, 00197 const struct iio_ch_info* channel, 00198 intptr_t id) 00199 { 00200 float scale; 00201 00202 (void)sscanf(buf, "%f", &scale); 00203 00204 if (scale > 0.0) { 00205 attr_scale_val[channel->ch_num] = scale; 00206 return len; 00207 } 00208 00209 return -EINVAL; 00210 } 00211 00212 /*! 00213 * @brief Getter for the power mode available values 00214 * @param device- pointer to IIO device structure 00215 * @param buf- pointer to buffer holding attribute value 00216 * @param len- length of buffer string data 00217 * @param channel- pointer to IIO channel structure 00218 * @param id- Attribute ID (optional) 00219 * @return Number of characters read/written 00220 */ 00221 static ssize_t get_power_mode_available(void *device, 00222 char *buf, 00223 size_t len, 00224 const struct iio_ch_info *channel, 00225 intptr_t id) 00226 { 00227 return sprintf(buf, "%s", "Eco-Mode Value-Not-Assigned Median-Mode Fast-Mode"); 00228 } 00229 00230 /*! 00231 * @brief Setter for the power mode available values 00232 * @param device- pointer to IIO device structure 00233 * @param buf- pointer to buffer holding attribute value 00234 * @param len- length of buffer string data 00235 * @param channel- pointer to IIO channel structure 00236 * @param id- Attribute ID (optional) 00237 * @return Number of characters read/written 00238 */ 00239 static ssize_t set_power_mode_available(void *device, 00240 char *buf, 00241 size_t len, 00242 const struct iio_ch_info *channel, 00243 intptr_t id) 00244 { 00245 /* NA- Can't set error value */ 00246 return len; 00247 } 00248 00249 /*! 00250 * @brief Getter/Setter for the power mode attribute value 00251 * @param device- pointer to IIO device structure 00252 * @param buf- pointer to buffer holding attribute value 00253 * @param len- length of buffer string data 00254 * @param channel- pointer to IIO channel structure 00255 * @return Number of characters read/written 00256 */ 00257 ssize_t get_power_mode(void *device, 00258 char *buf, 00259 size_t len, 00260 const struct iio_ch_info *channel, 00261 intptr_t id) 00262 { 00263 uint8_t power_mode_value = 0; 00264 00265 if (ad77681_spi_read_mask(device, 00266 AD77681_REG_POWER_CLOCK, 00267 AD77681_POWER_CLK_PWRMODE_MSK, 00268 &power_mode_value) == SUCCESS) { 00269 return (ssize_t)sprintf(buf, "%s", power_mode_str[power_mode_value]); 00270 } 00271 00272 return -EINVAL; 00273 } 00274 00275 ssize_t set_power_mode(void *device, 00276 char *buf, 00277 size_t len, 00278 const struct iio_ch_info *channel, 00279 intptr_t id) 00280 { 00281 uint8_t power_mode_value; 00282 00283 for (power_mode_value = 0; 00284 power_mode_value < (uint8_t)ARRAY_SIZE(power_mode_str); power_mode_value++) { 00285 if (!strncmp(buf, power_mode_str[power_mode_value], 00286 strlen(power_mode_str[power_mode_value]))) { 00287 break; 00288 } 00289 } 00290 00291 if (power_mode_value < (uint8_t)ARRAY_SIZE(power_mode_str)) { 00292 if (ad77681_set_power_mode(device, power_mode_value) == SUCCESS) { 00293 return len; 00294 } 00295 } 00296 00297 return -EINVAL; 00298 } 00299 00300 /*! 00301 * @brief Getter for the conv mode available values 00302 * @param device- pointer to IIO device structure 00303 * @param buf- pointer to buffer holding attribute value 00304 * @param len- length of buffer string data 00305 * @param channel- pointer to IIO channel structure 00306 * @param id- Attribute ID (optional) 00307 * @return Number of characters read/written 00308 */ 00309 static ssize_t get_conv_mode_available(void *device, 00310 char *buf, 00311 size_t len, 00312 const struct iio_ch_info *channel, 00313 intptr_t id) 00314 { 00315 return sprintf(buf, "%s", 00316 "Continuous-Mode Continious-One-Shot-Mode Single-Mode Periodic-Mode Standby-Mode"); 00317 } 00318 00319 /*! 00320 * @brief Setter for the conv mode available values 00321 * @param device- pointer to IIO device structure 00322 * @param buf- pointer to buffer holding attribute value 00323 * @param len- length of buffer string data 00324 * @param channel- pointer to IIO channel structure 00325 * @param id- Attribute ID (optional) 00326 * @return Number of characters read/written 00327 */ 00328 static ssize_t set_conv_mode_available(void *device, 00329 char *buf, 00330 size_t len, 00331 const struct iio_ch_info *channel, 00332 intptr_t id) 00333 { 00334 /* NA- Can't set error value */ 00335 return len; 00336 } 00337 00338 /*! 00339 * @brief Getter/Setter for the conversion mode attribute value 00340 * @param device- pointer to IIO device structure 00341 * @param buf- pointer to buffer holding attribute value 00342 * @param len- length of buffer string data 00343 * @param channel- pointer to IIO channel structure 00344 * @return Number of characters read/written 00345 */ 00346 ssize_t get_conv_mode(void* device, 00347 char* buf, 00348 size_t len, 00349 const struct iio_ch_info* channel, 00350 intptr_t id) 00351 { 00352 uint8_t conv_mode_value = 0; 00353 00354 if (ad77681_spi_read_mask(device, 00355 AD77681_REG_CONVERSION, 00356 AD77681_CONVERSION_MODE_MSK, 00357 &conv_mode_value) == SUCCESS) { 00358 if (conv_mode_value <= (uint8_t)ARRAY_SIZE(conv_mode_str)) { 00359 return (ssize_t)sprintf(buf, "%s", conv_mode_str[conv_mode_value]); 00360 } else if (conv_mode_value == SINGLE_MODE_CONV_STANDBY) { 00361 return (ssize_t)sprintf(buf, "%s", conv_mode_str[2]); 00362 } else if (conv_mode_value == PERIODIC_MODE_CONV_STANDBY) { 00363 return (ssize_t)sprintf(buf, "%s", conv_mode_str[3]); 00364 } 00365 } 00366 00367 return -EINVAL; 00368 } 00369 00370 ssize_t set_conv_mode(void* device, 00371 char* buf, 00372 size_t len, 00373 const struct iio_ch_info* channel, 00374 intptr_t id) 00375 { 00376 uint8_t conv_mode_value; 00377 00378 for (conv_mode_value = 0; conv_mode_value < (uint8_t)ARRAY_SIZE(conv_mode_str); 00379 conv_mode_value++) { 00380 if (!strncmp(buf, conv_mode_str[conv_mode_value], 00381 strlen(conv_mode_str[conv_mode_value]))) { 00382 break; 00383 } 00384 } 00385 00386 if (conv_mode_value < (uint8_t)ARRAY_SIZE(conv_mode_str)) { 00387 if (ad77681_set_conv_mode(device, conv_mode_value, AD77681_AIN_SHORT, 00388 false) == SUCCESS) { 00389 return len; 00390 } 00391 } 00392 00393 return -EINVAL; 00394 } 00395 00396 /*! 00397 * @brief Getter for the mclk division available values 00398 * @param device- pointer to IIO device structure 00399 * @param buf- pointer to buffer holding attribute value 00400 * @param len- length of buffer string data 00401 * @param channel- pointer to IIO channel structure 00402 * @param id- Attribute ID (optional) 00403 * @return Number of characters read/written 00404 */ 00405 00406 static ssize_t get_mclk_division_available(void *device, 00407 char *buf, 00408 size_t len, 00409 const struct iio_ch_info *channel, 00410 intptr_t id) 00411 { 00412 return sprintf(buf, "%s", 00413 "AD77681_MCLK_DIV_16 AD77681_MCLK_DIV_8 AD77681_MCLK_DIV_4 AD77681_MCLK_DIV_2"); 00414 } 00415 00416 /*! 00417 * @brief Setter for the mclk division available values 00418 * @param device- pointer to IIO device structure 00419 * @param buf- pointer to buffer holding attribute value 00420 * @param len- length of buffer string data 00421 * @param channel- pointer to IIO channel structure 00422 * @param id- Attribute ID (optional) 00423 * @return Number of characters read/written 00424 */ 00425 static ssize_t set_mclk_division_available(void *device, 00426 char *buf, 00427 size_t len, 00428 const struct iio_ch_info *channel, 00429 intptr_t id) 00430 { 00431 /* NA- Can't set error value */ 00432 return len; 00433 } 00434 00435 /*! 00436 * @brief Getter/Setter for the MCLK division attribute value 00437 * @param device- pointer to IIO device structure 00438 * @param buf- pointer to buffer holding attribute value 00439 * @param len- length of buffer string data 00440 * @param channel- pointer to IIO channel structure 00441 * @param channel- pointer to IIO channel structure 00442 * @return Number of characters read/written 00443 */ 00444 ssize_t get_mclk_division(void* device, 00445 char* buf, 00446 size_t len, 00447 const struct iio_ch_info* channel, 00448 intptr_t id) 00449 { 00450 uint8_t mclk_division_value = 0; 00451 00452 if (ad77681_spi_read_mask(device, 00453 AD77681_REG_POWER_CLOCK, 00454 AD77681_POWER_CLK_MCLK_DIV_MSK, 00455 &mclk_division_value) == SUCCESS) { 00456 return (ssize_t)sprintf(buf, "%s", mclk_division_str[mclk_division_value >> 4]); 00457 } 00458 00459 return -EINVAL; 00460 } 00461 00462 ssize_t set_mclk_division(void* device, 00463 char* buf, 00464 size_t len, 00465 const struct iio_ch_info* channel, 00466 intptr_t id) 00467 { 00468 uint8_t mclk_division_value = 0; 00469 uint8_t mclk_division_str_len = 0; 00470 00471 mclk_division_str_len = (uint8_t)ARRAY_SIZE(mclk_division_str); 00472 00473 for (uint8_t mclk_division_cntr = 0; mclk_division_cntr < mclk_division_str_len; 00474 mclk_division_cntr++) { 00475 if (!strncmp(buf, mclk_division_str[mclk_division_cntr], 00476 strlen(mclk_division_str[mclk_division_cntr]))) { 00477 mclk_division_value = mclk_division_cntr; 00478 break; 00479 } 00480 } 00481 00482 if (mclk_division_value < mclk_division_str_len) { 00483 if (ad77681_set_mclk_div(device, mclk_division_value) == SUCCESS) { 00484 return len; 00485 } 00486 } 00487 00488 return -EINVAL; 00489 } 00490 00491 /*! 00492 * @brief Get the actual register address value from the list 00493 * @param reg- Register address to read from 00494 * @param Reg_add - actual value of Register address 00495 * @return true in case of success, false value otherwise 00496 */ 00497 bool debug_get_reg_value(uint8_t reg, uint8_t* Reg_add) 00498 { 00499 bool ad77681_regs_debug_flg = false; 00500 uint8_t ad77681_regs_arr_cntr; 00501 00502 for (ad77681_regs_arr_cntr = 0; 00503 ad77681_regs_arr_cntr < (uint8_t)AD77681_REG_MAX_ADDR; 00504 ad77681_regs_arr_cntr++) { 00505 if (reg == ad77681_regs[ad77681_regs_arr_cntr]) { 00506 ad77681_regs_debug_flg = true; 00507 *Reg_add = reg; 00508 break; 00509 } 00510 } 00511 00512 return ad77681_regs_debug_flg; 00513 } 00514 00515 /*! 00516 * @brief Read the debug register value 00517 * @param dev- Pointer to IIO device instance 00518 * @param reg- Register address to read from 00519 * @param readval- Pointer to variable to read data into 00520 * @return SUCCESS in case of success, negative value otherwise 00521 */ 00522 int32_t debug_reg_read(void *dev, uint32_t reg, uint32_t *readval) 00523 { 00524 00525 bool ad77681_dev_debug_read_flg = false; 00526 uint8_t ad77681_dev_actual_reg_add = 0; 00527 00528 ad77681_dev_debug_read_flg = debug_get_reg_value((uint8_t)reg, 00529 &ad77681_dev_actual_reg_add); 00530 00531 /* Read the data from device */ 00532 if (ad77681_dev_debug_read_flg == true) { 00533 if ((ad77681_spi_reg_read(dev, (uint8_t)ad77681_dev_actual_reg_add, 00534 (uint8_t *)readval) == SUCCESS)) { 00535 // Shift 8 bits to get the uint8 value from uint32 00536 *readval = *readval >> 8; 00537 return SUCCESS; 00538 } 00539 } 00540 00541 return FAILURE; 00542 } 00543 00544 /*! 00545 * @brief Write into the debug register 00546 * @param dev- Pointer to IIO device instance 00547 * @param reg- Register address to write into 00548 * @param writeval- Register value to write 00549 * @return SUCCESS in case of success, negative value otherwise 00550 */ 00551 int32_t debug_reg_write(void *dev, uint32_t reg, uint32_t writeval) 00552 { 00553 bool ad77681_dev_debug_write_flg = false; 00554 uint8_t ad77681_dev_actual_reg_add = 0; 00555 00556 ad77681_dev_debug_write_flg = debug_get_reg_value((uint8_t)reg, 00557 &ad77681_dev_actual_reg_add); 00558 00559 if (ad77681_dev_debug_write_flg == true) { 00560 if (ad77681_spi_reg_write(dev, ad77681_dev_actual_reg_add, 00561 (uint8_t) writeval) == SUCCESS) { 00562 return SUCCESS; 00563 } 00564 } 00565 00566 return FAILURE; 00567 } 00568 00569 00570 /** 00571 * @brief Read buffer data corresponding to AD4170 IIO device 00572 * @param dev_instance[in] - IIO device instance 00573 * @param pbuf[out] - Pointer to output data buffer 00574 * @param offset[in] - Data buffer offset 00575 * @param bytes_count[in] - Number of bytes to read 00576 * @param ch_mask[in] - Channels select mask 00577 * @return SUCCESS in case of success or negative value otherwise 00578 */ 00579 static ssize_t iio_ad77681_read_data(void *dev_instance, 00580 char *pbuf, 00581 size_t offset, 00582 size_t bytes_count, 00583 uint32_t ch_mask) 00584 { 00585 if (adc_data_capture_started == false) { 00586 start_data_capture(ch_mask, AD77681_NUM_CHANNELS); 00587 adc_data_capture_started = true; 00588 } 00589 00590 /* Read the data stored into acquisition buffers */ 00591 return (ssize_t)read_buffered_data(pbuf, 00592 bytes_count, 00593 offset, 00594 ch_mask, 00595 AD77681_BYTES_PER_SAMPLE); 00596 } 00597 00598 /** 00599 * @brief Transfer the device data into memory (optional) 00600 * @param dev_instance[in] - IIO device instance 00601 * @param bytes_count[in] - Number of bytes to read 00602 * @param ch_mask[in] - Channels select mask 00603 * @return SUCCESS in case of success or negative value otherwise 00604 */ 00605 static ssize_t iio_ad77681_transfer_dev_data(void *dev_instance, 00606 size_t bytes_count, 00607 uint32_t ch_mask) 00608 { 00609 /* The function insures that data is first read into memory from the device. 00610 * This function doesn't do any sort of data transfer but it make sure data 00611 * read and it's transfer to memory from device is happening in application through 00612 * iio_ad4130_read_data() function */ 00613 00614 /* Store the requested samples count value for data capture */ 00615 store_requested_samples_count(bytes_count, AD77681_BYTES_PER_SAMPLE); 00616 00617 return SUCCESS; 00618 } 00619 00620 /** 00621 * @brief Perform tasks before new data transfer 00622 * @param dev_instance[in] - IIO device instance 00623 * @param ch_mask[in] - Channels select mask 00624 * @return SUCCESS in case of success or negative value otherwise 00625 */ 00626 static int32_t iio_ad77681_start_transfer(void *dev_instance, uint32_t ch_mask) 00627 { 00628 return SUCCESS; 00629 } 00630 00631 00632 /** 00633 * @brief Perform tasks before end of current data transfer 00634 * @param dev_instance[in] - IIO device instance 00635 * @return SUCCESS in case of success or negative value otherwise 00636 */ 00637 static int32_t iio_ad77681_stop_transfer(void *dev) 00638 { 00639 adc_data_capture_started = false; 00640 stop_data_capture(); 00641 00642 return SUCCESS; 00643 } 00644 00645 /********************************************************* 00646 * IIO Attributes and Structures 00647 ********************************************************/ 00648 00649 /* IIOD channels attributes list */ 00650 struct iio_attribute channel_input_attributes[] = { 00651 { 00652 .name = "raw", 00653 .show = get_raw, 00654 .store = set_raw, 00655 }, 00656 { 00657 .name = "scale", 00658 .show = get_scale, 00659 .store = set_scale, 00660 }, 00661 00662 END_ATTRIBUTES_ARRAY 00663 }; 00664 00665 /* IIOD device (global) attributes list */ 00666 static struct iio_attribute global_attributes[] = { 00667 { 00668 .name = "sampling_frequency", 00669 .show = get_sampling_frequency, 00670 .store = set_sampling_frequency, 00671 }, 00672 { 00673 .name = "conv_mode_available", 00674 .show = get_conv_mode_available, 00675 .store = set_conv_mode_available, 00676 }, 00677 { 00678 .name = "conv_mode", 00679 .show = get_conv_mode, 00680 .store = set_conv_mode, 00681 }, 00682 { 00683 .name = "power_mode_available", 00684 .show = get_power_mode_available, 00685 .store = set_power_mode_available, 00686 }, 00687 { 00688 .name = "power_mode", 00689 .show = get_power_mode, 00690 .store = set_power_mode, 00691 }, 00692 { 00693 .name = "mclk_division_available", 00694 .show = get_mclk_division_available, 00695 .store = set_mclk_division_available, 00696 }, 00697 { 00698 .name = "mclk_division", 00699 .show = get_mclk_division, 00700 .store = set_mclk_division, 00701 }, 00702 00703 END_ATTRIBUTES_ARRAY 00704 00705 }; 00706 00707 /* IIOD debug attributes list */ 00708 static struct iio_attribute debug_attributes[] = { 00709 { 00710 .name = "direct_reg_access", 00711 .show = NULL, 00712 .store = NULL, 00713 }, 00714 00715 END_ATTRIBUTES_ARRAY 00716 }; 00717 00718 /* IIOD channels configurations */ 00719 struct scan_type chn_scan = { 00720 .sign = 's', 00721 .realbits = AD77681_CHN_STORAGE_BITS, 00722 .storagebits = AD77681_CHN_STORAGE_BITS, 00723 .shift = 0, 00724 .is_big_endian = false 00725 }; 00726 00727 /* IIOD Channel list */ 00728 static struct iio_channel iio_ad77681_channels[] = { 00729 { 00730 .name = "voltage0", 00731 .ch_type = IIO_VOLTAGE, 00732 .channel = 0, 00733 .scan_index = 0, 00734 .scan_type = &chn_scan, 00735 .attributes = channel_input_attributes, 00736 .ch_out = false, 00737 .indexed = true, 00738 }, 00739 }; 00740 00741 /** 00742 * @brief Init for reading/writing and parameterization of a 00743 * ad77681 IIO device 00744 * @param desc[in,out] - IIO device descriptor 00745 * @return SUCCESS in case of success, FAILURE otherwise 00746 */ 00747 static int32_t iio_ad77681_init(struct iio_device **desc) 00748 { 00749 struct iio_device *iio_ad77861_inst; 00750 00751 iio_ad77861_inst = calloc(1, sizeof(struct iio_device)); 00752 if (!iio_ad77861_inst) { 00753 return FAILURE; 00754 } 00755 00756 iio_ad77861_inst->num_ch = sizeof(iio_ad77681_channels) / sizeof( 00757 iio_ad77681_channels[0]); 00758 iio_ad77861_inst->channels = iio_ad77681_channels; 00759 iio_ad77861_inst->attributes = global_attributes; 00760 iio_ad77861_inst->debug_attributes = debug_attributes; 00761 iio_ad77861_inst->transfer_dev_to_mem = iio_ad77681_transfer_dev_data; 00762 iio_ad77861_inst->transfer_mem_to_dev = NULL; 00763 iio_ad77861_inst->read_data = iio_ad77681_read_data; 00764 iio_ad77861_inst->write_data = NULL; 00765 iio_ad77861_inst->prepare_transfer = iio_ad77681_start_transfer; 00766 iio_ad77861_inst->end_transfer = iio_ad77681_stop_transfer; 00767 iio_ad77861_inst->debug_reg_read = debug_reg_read; 00768 iio_ad77861_inst->debug_reg_write = debug_reg_write; 00769 00770 *desc = iio_ad77861_inst; 00771 00772 return SUCCESS; 00773 } 00774 00775 00776 /** 00777 * @brief Release resources allocated for AD77681 IIO device 00778 * @param desc[in] - IIO device descriptor 00779 * @return SUCCESS in case of success, FAILURE otherwise 00780 */ 00781 static int32_t iio_ad77681_remove(struct iio_desc *desc) 00782 { 00783 int32_t status; 00784 00785 if (!desc) { 00786 return FAILURE; 00787 } 00788 00789 status = iio_unregister(desc, (char *)dev_name); 00790 if (status != SUCCESS) { 00791 return FAILURE; 00792 } 00793 00794 return SUCCESS; 00795 } 00796 00797 /** 00798 * @brief Initialize the IIO interface for AD77681 IIO device 00799 * @return none 00800 * @return SUCCESS in case of success, FAILURE otherwise 00801 */ 00802 int32_t ad77681_iio_initialize(void) 00803 { 00804 int32_t init_status = FAILURE; 00805 00806 /* IIO device descriptor */ 00807 struct iio_device *p_iio_ad77681_dev; 00808 00809 /** 00810 * IIO interface init parameters 00811 */ 00812 struct iio_init_param iio_init_params = { 00813 .phy_type = USE_UART, 00814 { 00815 &uart_init_params 00816 } 00817 }; 00818 00819 /* Initialize AD77681 device and peripheral interface */ 00820 init_status = ad77681_setup(&p_ad77681_dev_inst, sad77681_init, 00821 &p_ad77681_stat_reg); 00822 if (init_status != SUCCESS) { 00823 return init_status; 00824 } 00825 00826 /* Initialize the IIO interface */ 00827 init_status = iio_init(&p_ad77681_iio_desc, &iio_init_params); 00828 if (init_status != SUCCESS) { 00829 return init_status; 00830 } 00831 00832 /* Initialize the AD77681 IIO application interface */ 00833 init_status = iio_ad77681_init(&p_iio_ad77681_dev); 00834 if (init_status != SUCCESS) { 00835 return init_status; 00836 } 00837 00838 /* Register AD77681 IIO interface */ 00839 init_status = iio_register(p_ad77681_iio_desc, 00840 p_iio_ad77681_dev, 00841 (char *)dev_name, 00842 p_ad77681_dev_inst, 00843 NULL, 00844 NULL); 00845 00846 if (init_status != SUCCESS) { 00847 return init_status; 00848 } 00849 00850 /* Init the system peripherals */ 00851 init_status = init_system(); 00852 if (init_status != SUCCESS) { 00853 return init_status; 00854 } 00855 00856 return init_status; 00857 } 00858 00859 /** 00860 * @brief Run the AD77681 IIO event handler 00861 * @return none 00862 * @details This function monitors the new IIO client event 00863 */ 00864 void ad77681_iio_event_handler(void) 00865 { 00866 while (1) { 00867 (void)iio_step(p_ad77681_iio_desc); 00868 } 00869 } 00870 00871 00872
Generated on Tue Jul 12 2022 19:15:23 by
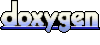