
EVAL-AD7124 Mbed Example Program.
Dependencies: adi_console_menu platform_drivers
ad7124_support.c
00001 /*! 00002 ***************************************************************************** 00003 @file: ad7124_support.c 00004 00005 @brief: Provides useful support functions for the AD7124 NoOS driver 00006 00007 @details: 00008 ----------------------------------------------------------------------------- 00009 Copyright (c) 2019, 2020 Analog Devices, Inc. 00010 All rights reserved. 00011 00012 This software is proprietary to Analog Devices, Inc. and its licensors. 00013 By using this software you agree to the terms of the associated 00014 Analog Devices Software License Agreement. 00015 *****************************************************************************/ 00016 00017 #include <stdbool.h> 00018 #include "ad7124_support.h" 00019 00020 // Public Functions 00021 /* 00022 * @brief helper function get the setup setting for an ADC channel 00023 * 00024 * @param dev The device structure. 00025 * 00026 * @param channel ADC channel to get Setup for. 00027 * 00028 * @return value of setup field in channel configuration. 00029 */ 00030 uint8_t ad7124_get_channel_setup(struct ad7124_dev *dev, uint8_t channel) 00031 { 00032 return (dev->regs[AD7124_Channel_0 + channel].value >> 12) & 0x7; 00033 } 00034 00035 00036 /* 00037 * @brief helper function get the PGA setting for an ADC channel 00038 * 00039 * @param dev The device structure. 00040 * 00041 * @param channel ADC channel to get Setup for. 00042 * 00043 * @return value of PGA field in the setup for an ADC channel. 00044 */ 00045 uint8_t ad7124_get_channel_pga(struct ad7124_dev *dev, uint8_t channel) 00046 { 00047 uint8_t setup = ad7124_get_channel_setup(dev, channel); 00048 00049 return (dev->regs[AD7124_Config_0 + setup].value) & 0x07; 00050 } 00051 00052 00053 /* 00054 * @brief helper function get the bipolar setting for an ADC channel 00055 * 00056 * @param dev The device structure. 00057 * 00058 * @param channel ADC channel to get bipolar mode for. 00059 * 00060 * @return value of bipolar field in the setup for an ADC channel. 00061 */ 00062 bool ad7124_get_channel_bipolar(struct ad7124_dev *dev, uint8_t channel) 00063 { 00064 uint8_t setup = ad7124_get_channel_setup(dev, channel); 00065 00066 return ((dev->regs[AD7124_Config_0 + setup].value >> 11) & 0x1) ? true : false; 00067 } 00068 00069 00070 /* 00071 * @brief converts ADC sample value to voltage based on gain setting 00072 * 00073 * @param dev The device structure. 00074 * 00075 * @param channel ADC channel to get Setup for. 00076 * 00077 * @param sample Raw ADC sample 00078 * 00079 * @return Sample ADC value converted to voltage. 00080 * 00081 * @note The conversion equation is implemented for simplicity, 00082 * not for accuracy or performance 00083 * 00084 */ 00085 float ad7124_convert_sample_to_voltage(struct ad7124_dev *dev, uint8_t channel, 00086 uint32_t sample) 00087 { 00088 bool isBipolar = ad7124_get_channel_bipolar(dev, channel); 00089 uint8_t channelPGA = ad7124_get_channel_pga(dev, channel); 00090 00091 float convertedValue; 00092 00093 if (isBipolar) { 00094 convertedValue = ( ((float)sample / (1 << (AD7124_ADC_N_BITS -1))) -1 ) * \ 00095 (AD7124_REF_VOLTAGE / AD7124_PGA_GAIN(channelPGA)); 00096 } else { 00097 convertedValue = ((float)sample * AD7124_REF_VOLTAGE)/(AD7124_PGA_GAIN( 00098 channelPGA) * \ 00099 (1 << AD7124_ADC_N_BITS)); 00100 } 00101 00102 return (convertedValue); 00103 }
Generated on Fri Jul 15 2022 23:06:34 by
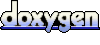