
Example program for EVAL-AD5770R
Dependencies: adi_console_menu platform_drivers
ad5770r_console_app.c
00001 /*! 00002 ***************************************************************************** 00003 @file: ad5770r_console_app.c 00004 00005 @brief: Implementation for the menu functions that handle the AD5770R 00006 00007 @details: 00008 ----------------------------------------------------------------------------- 00009 * 00010 Copyright (c) 2020-2021 Analog Devices, Inc. All Rights Reserved. 00011 00012 This software is proprietary to Analog Devices, Inc. and its licensors. 00013 By using this software you agree to the terms of the associated 00014 Analog Devices Software License Agreement. 00015 ******************************************************************************/ 00016 00017 /* includes */ 00018 #include <stdio.h> 00019 #include <stdlib.h> 00020 #include <string.h> 00021 #include <stdbool.h> 00022 #include <stdint.h> 00023 #include <assert.h> 00024 00025 #include "app_config.h" 00026 00027 #include "delay.h" 00028 #include "error.h" 00029 #include "gpio.h" 00030 #include "spi.h" 00031 #include "platform_support.h" 00032 #include "ad5770r.h" 00033 00034 #include "ad5770r_console_app.h" 00035 #include "ad5770r_user_config.h" 00036 #include "ad5770r_reset_config.h" 00037 00038 /* defines */ 00039 #define TOGGLE_MUX_BUFFER 1000 00040 #define TOGGLE_DIODE_EXT_BIAS 1001 00041 #define MENU_CHANNEL_OFFSET 100 00042 00043 /* Private Variables */ 00044 static struct ad5770r_dev * pAd5770r_dev = NULL; 00045 00046 static struct ad5770r_channel_switches sw_ldac_shadow; 00047 00048 // GPIO descriptor and init parameters for the HW LDACB pin 00049 static gpio_desc * hw_ldacb_desc = NULL; 00050 static gpio_init_param hw_ldacb_init_param = { HW_LDACB, NULL }; 00051 00052 00053 // Forward Declaration 00054 static console_menu general_configuration_menu; 00055 static console_menu monitor_setup_menu; 00056 static console_menu dac_channel_configuration_menu; 00057 static console_menu dac_operations_menu; 00058 00059 // Public Functions 00060 00061 /*! 00062 * @brief Initialize the AD7124 device and the SPI port as required 00063 * 00064 * @details This resets and then writes the default register map value to 00065 * the device. A call to init the SPI port is made, but may not 00066 * actually do very much, depending on the platform 00067 */ 00068 int32_t ad5770r_app_initialize(void) 00069 { 00070 00071 // Create a new descriptor for HW LDACB 00072 if(gpio_get(&hw_ldacb_desc, &hw_ldacb_init_param) == FAILURE) { 00073 return FAILURE; 00074 } 00075 00076 // Set the direction of HW LDACB 00077 if((gpio_direction_output(hw_ldacb_desc, GPIO_OUT)) == FAILURE) { 00078 return FAILURE; 00079 } 00080 00081 // Set the default output state of HW LDACB 00082 if((gpio_set_value(hw_ldacb_desc, GPIO_HIGH)) == FAILURE) { 00083 return FAILURE; 00084 } 00085 00086 return(ad5770r_init(&pAd5770r_dev, &ad5770r_user_param)); 00087 } 00088 00089 // Private Functions 00090 00091 /** 00092 * Performs a software reset. 00093 * @param dev - The device structure. 00094 * @return SUCCESS in case of success, negative error code otherwise. 00095 */ 00096 int32_t ad5770r_software_reset(struct ad5770r_dev *dev) 00097 { 00098 int32_t ret; 00099 00100 if (!dev) 00101 return FAILURE; 00102 00103 ret = ad5770r_spi_reg_write(dev, 00104 AD5770R_INTERFACE_CONFIG_A, 00105 AD5770R_INTERFACE_CONFIG_A_SW_RESET_MSK | 00106 AD5770R_INTERFACE_CONFIG_A_ADDR_ASCENSION_MSB( 00107 dev->dev_spi_settings.addr_ascension)); 00108 00109 if (ret) 00110 return ret; 00111 00112 // Save the spi_desc pointer field 00113 spi_desc * spi_interface = dev->spi_desc; 00114 // Copy over the reset state of the device 00115 memcpy(dev, &ad5770r_dev_reset, sizeof(ad5770r_dev_reset)); 00116 // Restore the spi_desc pointer field 00117 dev->spi_desc = spi_interface; 00118 00119 return ret; 00120 } 00121 00122 /* 00123 * @brief Sends a reset command on the SPI to reset the device 00124 * 00125 * @details 00126 */ 00127 static int32_t do_software_reset(uint32_t id) 00128 { 00129 int32_t ret; 00130 00131 if ((ret = ad5770r_software_reset(pAd5770r_dev)) == SUCCESS) { 00132 printf(EOL " --- Software Reset Succeeded ---" EOL); 00133 } else { 00134 printf(EOL " *** Software Reset Failure: %d ***" EOL, ret); 00135 } 00136 adi_press_any_key_to_continue(); 00137 return(MENU_CONTINUE); 00138 } 00139 00140 /* 00141 * @brief Creates and initializes a device with user configuration 00142 * 00143 * @details 00144 */ 00145 static int32_t do_device_init(uint32_t id) 00146 { 00147 00148 if (ad5770r_init(&pAd5770r_dev, &ad5770r_user_param) == SUCCESS) { 00149 } else { 00150 printf("\n\r *** Error device init ***\n\r"); 00151 } 00152 00153 adi_press_any_key_to_continue(); 00154 return(MENU_CONTINUE); 00155 } 00156 00157 /* 00158 * @brief Removes the device from memory 00159 * 00160 * @details 00161 */ 00162 static int32_t do_device_remove(uint32_t id) 00163 { 00164 if (ad5770r_remove(pAd5770r_dev) != SUCCESS) { 00165 printf("\n\r *** Error doing device remove ***\n\r"); 00166 } 00167 00168 pAd5770r_dev = NULL; 00169 00170 adi_press_any_key_to_continue(); 00171 return(MENU_CONTINUE); 00172 } 00173 00174 /*! 00175 * @brief toggles the int/ext ref resistor option 00176 * 00177 * @details 00178 */ 00179 static int32_t do_toggle_ref_resistor(uint32_t id) 00180 { 00181 int32_t status; 00182 00183 if ((status = ad5770r_set_reference(pAd5770r_dev, 00184 !pAd5770r_dev->external_reference, 00185 pAd5770r_dev->reference_selector)) != SUCCESS) { 00186 printf(EOL " *** Error toggling ref resistor setting: %d" EOL, status); 00187 adi_press_any_key_to_continue(); 00188 } 00189 00190 return(MENU_CONTINUE); 00191 } 00192 00193 00194 /*! 00195 * @brief sets the int/ext refer configuration options 00196 * 00197 * @details 00198 */ 00199 static int32_t do_set_reference(uint32_t ref_option) 00200 { 00201 int32_t status; 00202 00203 if ((status = ad5770r_set_reference(pAd5770r_dev, 00204 pAd5770r_dev->external_reference, 00205 (enum ad5770r_reference_voltage)ref_option)) != SUCCESS) { 00206 printf(EOL " *** Error toggling ref resistor setting: %d" EOL, status); 00207 adi_press_any_key_to_continue(); 00208 } 00209 00210 return(MENU_CONTINUE); 00211 } 00212 00213 /*! 00214 * @brief Sets the Alarm Menu option bits 00215 * 00216 * @details 00217 */ 00218 static int32_t do_set_alarm(uint32_t alarm_id) 00219 { 00220 int32_t status; 00221 struct ad5770r_alarm_cfg alarm_config; 00222 00223 alarm_config = pAd5770r_dev->alarm_config; 00224 00225 switch(alarm_id) { 00226 case AD5770R_ALARM_CONFIG_OPEN_DRAIN_EN(1): 00227 alarm_config.open_drain_en = !alarm_config.open_drain_en; 00228 break; 00229 case AD5770R_ALARM_CONFIG_THERMAL_SHUTDOWN_EN(1): 00230 alarm_config.thermal_shutdown_en = !alarm_config.thermal_shutdown_en; 00231 break; 00232 case AD5770R_ALARM_CONFIG_BACKGROUND_CRC_EN(1): 00233 alarm_config.background_crc_en = !alarm_config.background_crc_en; 00234 break; 00235 case AD5770R_ALARM_CONFIG_TEMP_WARNING_ALARM_MASK(1): 00236 alarm_config.temp_warning_msk = !alarm_config.temp_warning_msk; 00237 break; 00238 case AD5770R_ALARM_CONFIG_OVER_TEMP_ALARM_MASK(1): 00239 alarm_config.over_temp_msk = !alarm_config.over_temp_msk; 00240 break; 00241 case AD5770R_ALARM_CONFIG_NEGATIVE_CHANNEL0_ALARM_MASK(1): 00242 alarm_config.neg_ch0_msk = !alarm_config.neg_ch0_msk; 00243 break; 00244 case AD5770R_ALARM_CONFIG_IREF_FAULT_ALARM_MASK(1): 00245 alarm_config.iref_fault_msk = !alarm_config.iref_fault_msk; 00246 break; 00247 case AD5770R_ALARM_CONFIG_BACKGROUND_CRC_ALARM_MASK(1): 00248 alarm_config.background_crc_msk = !alarm_config.background_crc_msk; 00249 break; 00250 default: 00251 // not a supported menu option 00252 assert(false); 00253 } 00254 00255 if ((status = ad5770r_set_alarm(pAd5770r_dev, 00256 &alarm_config)) != SUCCESS) { 00257 printf(EOL " *** Error setting alarm config: %d" EOL, status); 00258 adi_press_any_key_to_continue(); 00259 } 00260 00261 return(MENU_CONTINUE); 00262 } 00263 00264 /*! 00265 * @brief Sets the Channel Configuration option bits 00266 * 00267 * @details 00268 */ 00269 static void ch_switches_toggle(struct ad5770r_channel_switches *ch_switches, 00270 uint32_t channel_id) 00271 { 00272 switch(channel_id) { 00273 case AD5770R_CHANNEL_CONFIG_CH0_SHUTDOWN_B(1): 00274 ch_switches->en0 = !ch_switches->en0; 00275 break; 00276 case AD5770R_CHANNEL_CONFIG_CH1_SHUTDOWN_B(1): 00277 ch_switches->en1 = !ch_switches->en1; 00278 break; 00279 case AD5770R_CHANNEL_CONFIG_CH2_SHUTDOWN_B(1): 00280 ch_switches->en2 = !ch_switches->en2; 00281 break; 00282 case AD5770R_CHANNEL_CONFIG_CH3_SHUTDOWN_B(1): 00283 ch_switches->en3 = !ch_switches->en3; 00284 break; 00285 case AD5770R_CHANNEL_CONFIG_CH4_SHUTDOWN_B(1): 00286 ch_switches->en4 = !ch_switches->en4; 00287 break; 00288 case AD5770R_CHANNEL_CONFIG_CH5_SHUTDOWN_B(1): 00289 ch_switches->en5 = !ch_switches->en5; 00290 break; 00291 case AD5770R_CHANNEL_CONFIG_CH0_SINK_EN(1): 00292 ch_switches->sink0 = !ch_switches->sink0; 00293 break; 00294 default: 00295 // not a supported menu option 00296 assert(false); 00297 } 00298 } 00299 00300 /*! 00301 * @brief Sets the Channel Configuration option bits 00302 * 00303 * @details 00304 */ 00305 static int32_t do_channel_config(uint32_t channel_id) 00306 { 00307 int32_t status; 00308 struct ad5770r_channel_switches channel_config; 00309 00310 channel_config = pAd5770r_dev->channel_config; 00311 00312 ch_switches_toggle(&channel_config, channel_id); 00313 00314 if ((status = ad5770r_channel_config(pAd5770r_dev, 00315 &channel_config)) != SUCCESS) { 00316 printf(EOL " *** Error setting channel config: %d" EOL, status); 00317 adi_press_any_key_to_continue(); 00318 } 00319 00320 return(MENU_CONTINUE); 00321 } 00322 00323 /*! 00324 * @brief prompts user for value to write to input register on channel 00325 * 00326 * @details 00327 */ 00328 static int32_t do_input_value(uint32_t channel_id) 00329 { 00330 int32_t status; 00331 uint16_t value; 00332 00333 printf(EOL "Enter Input register value (hex) for channel %d: " EOL, 00334 channel_id); 00335 value = adi_get_hex_integer(4); 00336 00337 /* Channels are 14-bits, mask off top 2 bits*/ 00338 value &= 0x3FFF; 00339 00340 if ((status = 00341 ad5770r_set_dac_input(pAd5770r_dev, value, 00342 (enum ad5770r_channels) channel_id)) != SUCCESS) { 00343 printf(EOL " *** Error writing DAC Input register: %d" EOL, status); 00344 adi_press_any_key_to_continue(); 00345 } 00346 00347 return(MENU_CONTINUE); 00348 } 00349 00350 /*! 00351 * @brief prompts user for value to write to input register on channel 00352 * 00353 * @details 00354 */ 00355 static int32_t do_dac_value(uint32_t channel_id) 00356 { 00357 int32_t status; 00358 uint16_t value; 00359 00360 printf(EOL "Enter DAC register value (hex) for channel %d: " EOL, channel_id); 00361 value = adi_get_hex_integer(4); 00362 00363 /* Channels are 14-bits, mask off top 2 bits*/ 00364 value &= 0x3FFF; 00365 00366 if ((status = 00367 ad5770r_set_dac_value(pAd5770r_dev, value, 00368 (enum ad5770r_channels) channel_id)) != SUCCESS) { 00369 printf(EOL " *** Error writing DAC value register: %d" EOL, status); 00370 adi_press_any_key_to_continue(); 00371 } 00372 00373 return(MENU_CONTINUE); 00374 } 00375 00376 /*! 00377 * @brief updating shadow SW LDAC, by toggling the channel bit 00378 * 00379 * @details 00380 */ 00381 static int32_t do_sw_ldac(uint32_t channel_id) 00382 { 00383 ch_switches_toggle(&sw_ldac_shadow, channel_id); 00384 00385 return(MENU_CONTINUE); 00386 } 00387 00388 /*! 00389 * @brief Writing SW LDAC to device 00390 * 00391 * @details 00392 */ 00393 static int32_t do_sw_ldac_write(uint32_t id) 00394 { 00395 int32_t status; 00396 00397 if ((status = ad5770r_set_sw_ldac(pAd5770r_dev, 00398 &sw_ldac_shadow)) != SUCCESS) { 00399 printf(EOL " *** Error writing SW LDAC: %d" EOL, status); 00400 adi_press_any_key_to_continue(); 00401 } 00402 00403 return(MENU_CONTINUE); 00404 } 00405 00406 /*! 00407 * @brief Toggles HW LDAC 00408 * 00409 * @details This toggles the LDAC pin on the device, but is independent to the driver 00410 * Therefore this does not update dac_values from input values. 00411 */ 00412 static int32_t do_hw_ldac_toggle(uint32_t id) 00413 { 00414 int32_t status; 00415 00416 do { 00417 if ((status = gpio_set_value(hw_ldacb_desc, GPIO_LOW)) == FAILURE) { 00418 break; 00419 } 00420 mdelay(1); 00421 if ((status = gpio_set_value(hw_ldacb_desc, GPIO_HIGH)) == FAILURE) { 00422 break; 00423 } 00424 } while (0); 00425 00426 if (status == SUCCESS) { 00427 printf(EOL " --- HW LDAC toggled ---" EOL); 00428 } else { 00429 printf(EOL " *** Error toggling HW LDACB ***" EOL); 00430 } 00431 00432 00433 adi_press_any_key_to_continue(); 00434 00435 return(MENU_CONTINUE); 00436 } 00437 00438 /*! 00439 * @brief displays general device configuration state 00440 * 00441 * @details 00442 */ 00443 static void display_gen_config(void) 00444 { 00445 printf("\tRef Resistor: %s\t\tRef Voltage: %d" EOL, 00446 pAd5770r_dev->external_reference == true ? "External" : "Internal", 00447 pAd5770r_dev->reference_selector); 00448 00449 printf("\tAlarms\tBgCRC Msk: %d\tIRef: %d\tneg: %d\tOT: %d " EOL \ 00450 "\t\tT Warn: %d\tBgCRC En: %d\tT Shdn: %d\tOD: %d" EOL, 00451 pAd5770r_dev->alarm_config.background_crc_msk, 00452 pAd5770r_dev->alarm_config.iref_fault_msk, 00453 pAd5770r_dev->alarm_config.neg_ch0_msk, 00454 pAd5770r_dev->alarm_config.over_temp_msk, 00455 pAd5770r_dev->alarm_config.temp_warning_msk, 00456 pAd5770r_dev->alarm_config.background_crc_en, 00457 pAd5770r_dev->alarm_config.thermal_shutdown_en, 00458 pAd5770r_dev->alarm_config.open_drain_en); 00459 } 00460 00461 /*! 00462 * @brief displays general device configuration state 00463 * 00464 * @details 00465 */ 00466 static void print_channel_switches(struct ad5770r_channel_switches *ch_switches, 00467 char * prefix, bool include_sink) 00468 { 00469 if (include_sink) { 00470 printf("\t%s - en0: %d sink0: %d en1: %d en2: %d " \ 00471 "en3: %d en4: %d en5: %d" EOL, prefix, 00472 ch_switches->en0, ch_switches->sink0, ch_switches->en1, 00473 ch_switches->en2, ch_switches->en3, ch_switches->en4, 00474 ch_switches->en5); 00475 } else { 00476 printf("\t%s - ch0: %d ch1: %d ch2: %d " \ 00477 "ch3: %d ch4: %d ch5: %d" EOL, prefix, 00478 ch_switches->en0, ch_switches->en1, ch_switches->en2, 00479 ch_switches->en3, ch_switches->en4, ch_switches->en5); 00480 } 00481 } 00482 00483 /*! 00484 * @brief displays general device configuration state 00485 * 00486 * @details 00487 */ 00488 static void display_dac_channel_configuration_header(void) 00489 { 00490 print_channel_switches(&pAd5770r_dev->channel_config, "Ch Configs", true); 00491 } 00492 00493 /*! 00494 * @brief displays the SW LDAC shadown and other channel output values 00495 * 00496 * @details 00497 */ 00498 static void display_dac_operations_header(void) 00499 { 00500 for (uint8_t i = 0; i < 6 ; i++) { 00501 printf("\tCh %i - Input: 0x%04X \t\tDAC: 0x%04X" EOL, i, 00502 pAd5770r_dev->input_value[i], pAd5770r_dev->dac_value[i]); 00503 } 00504 00505 printf(EOL); 00506 print_channel_switches(&sw_ldac_shadow, "SW LDAC shadow", false); 00507 } 00508 /*! 00509 * @brief prints the provided monitor config to the terminal 00510 * 00511 * @details 00512 * @param ad5770R_monitor_config monitor_config - struct for the monitor config to be displayed 00513 * @return SUCCESS in case of success, negative error otherwise 00514 */ 00515 static void print_monitor_setup(const struct ad5770r_monitor_setup * mon_setup) 00516 { 00517 printf("\tMonitor: "); 00518 switch (mon_setup->monitor_function) { 00519 case AD5770R_DISABLE: { 00520 printf("Disabled "); 00521 break; 00522 } 00523 case AD5770R_VOLTAGE_MONITORING: { 00524 printf("Voltage Ch %d", mon_setup->monitor_channel); 00525 break; 00526 } 00527 case AD5770R_CURRENT_MONITORING: { 00528 printf("Current Ch %d", mon_setup->monitor_channel); 00529 break; 00530 } 00531 case AD5770R_TEMPERATURE_MONITORING: { 00532 printf("Temperature"); 00533 break; 00534 } 00535 } 00536 00537 printf("\tBuffer: "); 00538 if (mon_setup->mux_buffer == true) { 00539 printf("On"); 00540 } else { 00541 printf("Off"); 00542 } 00543 00544 printf("\tIB_Ext: "); 00545 if (mon_setup->ib_ext_en == true) { 00546 printf("On"); 00547 } else { 00548 printf("Off"); 00549 } 00550 printf(EOL); 00551 } 00552 00553 /*! 00554 * @brief configure the Mux Monitor setup 00555 * 00556 * @details 00557 */ 00558 static void display_monitor_setup_header(void) 00559 { 00560 print_monitor_setup(&pAd5770r_dev->mon_setup); 00561 } 00562 00563 /*! 00564 * @brief configure the Mux Monitor setup 00565 * 00566 * @details 00567 */ 00568 static int32_t do_monitor_setup(uint32_t id) 00569 { 00570 int32_t status; 00571 struct ad5770r_monitor_setup monitor_setup; 00572 00573 monitor_setup = pAd5770r_dev->mon_setup; 00574 00575 switch(id) { 00576 case AD5770R_DISABLE: 00577 monitor_setup.monitor_function = AD5770R_DISABLE; 00578 break; 00579 case AD5770R_VOLTAGE_MONITORING: 00580 monitor_setup.monitor_function = AD5770R_VOLTAGE_MONITORING; 00581 break; 00582 case AD5770R_CURRENT_MONITORING: 00583 monitor_setup.monitor_function = AD5770R_CURRENT_MONITORING; 00584 break; 00585 case AD5770R_TEMPERATURE_MONITORING: 00586 monitor_setup.monitor_function = AD5770R_TEMPERATURE_MONITORING; 00587 break; 00588 case TOGGLE_MUX_BUFFER: 00589 monitor_setup.mux_buffer = !monitor_setup.mux_buffer; 00590 break; 00591 case TOGGLE_DIODE_EXT_BIAS: 00592 monitor_setup.ib_ext_en = !monitor_setup.ib_ext_en; 00593 break; 00594 default: 00595 // ensure the id is valid. 00596 assert(( id >= AD5770R_CH0 + MENU_CHANNEL_OFFSET ) 00597 && (id <= AD5770R_CH5 + MENU_CHANNEL_OFFSET)); 00598 monitor_setup.monitor_channel = (enum ad5770r_channels)( 00599 id - MENU_CHANNEL_OFFSET); 00600 } 00601 00602 if ((status = ad5770r_set_monitor_setup(pAd5770r_dev, 00603 &monitor_setup)) != SUCCESS) { 00604 printf(EOL " *** Error setting monitor setup: %d" EOL, status); 00605 adi_press_any_key_to_continue(); 00606 } 00607 00608 return(MENU_CONTINUE); 00609 } 00610 00611 /*! 00612 * @brief displays several pieces of status information above main menu 00613 * 00614 * @details 00615 */ 00616 static void display_main_menu_header(void) 00617 { 00618 int32_t ret; 00619 uint8_t device_status = 0, interface_status = 0, scratchpad = 0; 00620 00621 if (pAd5770r_dev == NULL) { 00622 printf(EOL " *** Device Not Initialized ***" EOL); 00623 return; 00624 } 00625 00626 do { 00627 if ((ret = ad5770r_get_status(pAd5770r_dev, &device_status)) != SUCCESS) { 00628 break; 00629 } 00630 if ((ret = ad5770r_get_interface_status(pAd5770r_dev, 00631 &interface_status)) != SUCCESS) { 00632 break; 00633 } 00634 if ((ret = ad5770r_spi_reg_read(pAd5770r_dev, AD5770R_SCRATCH_PAD, 00635 &scratchpad)) != SUCCESS) { 00636 break; 00637 } 00638 00639 } while(0); 00640 00641 if (ret != SUCCESS) { 00642 printf(EOL " *** Error in display state: %d **" EOL, ret); 00643 } 00644 00645 printf(EOL "\tInterface Status = 0x%02X\t\tDevice Status = 0x%02X" 00646 EOL "\tScratchpad = 0x%02X" EOL, 00647 interface_status, device_status, scratchpad); 00648 print_monitor_setup(&pAd5770r_dev->mon_setup); 00649 00650 // Increment the scratchpad by 1 to show a +1 delta in footer 00651 if((ret = ad5770r_spi_reg_write(pAd5770r_dev, AD5770R_SCRATCH_PAD, 00652 scratchpad + 1)) != SUCCESS) { 00653 printf(EOL " *** Error writing scratchpad + 1 : %d **" EOL, ret); 00654 } 00655 } 00656 00657 /*! 00658 * @brief displays several pieces of status information below main menu 00659 * 00660 * @details 00661 */ 00662 static void display_main_menu_footer(void) 00663 { 00664 int32_t ret; 00665 uint8_t scratchpad; 00666 00667 if (pAd5770r_dev == NULL) { 00668 printf(EOL " *** Device Not Initialized ***" EOL); 00669 return; 00670 } 00671 00672 if ((ret = ad5770r_spi_reg_read(pAd5770r_dev, AD5770R_SCRATCH_PAD, 00673 &scratchpad)) != SUCCESS) { 00674 printf(EOL " *** Error reading scratchpad: %d **" EOL, ret); 00675 } 00676 00677 printf(EOL "\tScratchpad = 0x%02X" EOL, scratchpad); 00678 } 00679 00680 /*! 00681 * @brief calls the general configuration menu 00682 */ 00683 static int32_t do_general_configuration_menu(uint32_t id) 00684 { 00685 return adi_do_console_menu(&general_configuration_menu); 00686 } 00687 00688 /*! 00689 * @brief calls the monitor setup menu 00690 */ 00691 static int32_t do_monitor_setup_menu(uint32_t id) 00692 { 00693 return adi_do_console_menu(&monitor_setup_menu); 00694 } 00695 00696 /*! 00697 * @brief calls the DAC channel confguration menu 00698 */ 00699 static int32_t do_dac_channel_configuration_menu(uint32_t id) 00700 { 00701 return adi_do_console_menu(&dac_channel_configuration_menu); 00702 } 00703 00704 /*! 00705 * @brief calls the DAC channel confguration menu 00706 */ 00707 static int32_t do_dac_operations_menu(uint32_t id) 00708 { 00709 return adi_do_console_menu(&dac_operations_menu); 00710 } 00711 00712 /* 00713 * DAC Operations Menu 00714 */ 00715 static console_menu_item dac_operations_menu_items[] = { 00716 {"\tSet Input Channel 0", 'Q', do_input_value, 0}, 00717 {"\tSet Input Channel 1", 'W', do_input_value, 1}, 00718 {"\tSet Input Channel 2", 'E', do_input_value, 2}, 00719 {"\tSet Input Channel 3", 'R', do_input_value, 3}, 00720 {"\tSet Input Channel 4", 'T', do_input_value, 4}, 00721 {"\tSet Input Channel 5", 'Y', do_input_value, 5}, 00722 {""}, 00723 {"\tToggle Channel 0 SW LDAC Shadow", '0', do_sw_ldac, AD5770R_HW_LDAC_MASK_CH(1, 0)}, 00724 {"\tToggle Channel 1 SW LDAC Shadow", '1', do_sw_ldac, AD5770R_HW_LDAC_MASK_CH(1, 1)}, 00725 {"\tToggle Channel 2 SW LDAC Shadow", '2', do_sw_ldac, AD5770R_HW_LDAC_MASK_CH(1, 2)}, 00726 {"\tToggle Channel 3 SW LDAC Shadow", '3', do_sw_ldac, AD5770R_HW_LDAC_MASK_CH(1, 3)}, 00727 {"\tToggle Channel 4 SW LDAC Shadow", '4', do_sw_ldac, AD5770R_HW_LDAC_MASK_CH(1, 4)}, 00728 {"\tToggle Channel 5 SW LDAC Shadow", '5', do_sw_ldac, AD5770R_HW_LDAC_MASK_CH(1, 5)}, 00729 {"\tWrite SW LDAC Shadow ", 'U', do_sw_ldac_write}, 00730 {""}, 00731 {"\tToggle HW LDAC digital input", 'J', do_hw_ldac_toggle}, 00732 {""}, 00733 {"\tSet DAC Channel 0", 'A', do_dac_value, 0}, 00734 {"\tSet DAC Channel 1", 'S', do_dac_value, 1}, 00735 {"\tSet DAC Channel 2", 'D', do_dac_value, 2}, 00736 {"\tSet DAC Channel 3", 'F', do_dac_value, 3}, 00737 {"\tSet DAC Channel 4", 'G', do_dac_value, 4}, 00738 {"\tSet DAC Channel 5", 'H', do_dac_value, 5}, 00739 }; 00740 00741 static console_menu dac_operations_menu = { 00742 .title = "DAC Operations", 00743 .items = dac_operations_menu_items, 00744 .itemCount = ARRAY_SIZE(dac_operations_menu_items), 00745 .headerItem = display_dac_operations_header, 00746 .footerItem = NULL, 00747 .enableEscapeKey = true 00748 }; 00749 00750 /* 00751 * Monitor Setup Menu 00752 */ 00753 static console_menu_item dac_channel_configuration_menu_items[] = { 00754 {"\tToggle Channel 0 Enable", '0', do_channel_config, AD5770R_CHANNEL_CONFIG_CH0_SHUTDOWN_B(1)}, 00755 {"\tToggle Channel 0 Sink Enable", 'S', do_channel_config, AD5770R_CHANNEL_CONFIG_CH0_SINK_EN(1)}, 00756 {"\tToggle Channel 1 Enable", '1', do_channel_config, AD5770R_CHANNEL_CONFIG_CH1_SHUTDOWN_B(1)}, 00757 {"\tToggle Channel 2 Enable", '2', do_channel_config, AD5770R_CHANNEL_CONFIG_CH2_SHUTDOWN_B(1)}, 00758 {"\tToggle Channel 3 Enable", '3', do_channel_config, AD5770R_CHANNEL_CONFIG_CH3_SHUTDOWN_B(1)}, 00759 {"\tToggle Channel 4 Enable", '4', do_channel_config, AD5770R_CHANNEL_CONFIG_CH4_SHUTDOWN_B(1)}, 00760 {"\tToggle Channel 5 Enable", '5', do_channel_config, AD5770R_CHANNEL_CONFIG_CH5_SHUTDOWN_B(1)} 00761 }; 00762 00763 static console_menu dac_channel_configuration_menu = { 00764 .title = "DAC Channel Configuration", 00765 .items = dac_channel_configuration_menu_items, 00766 .itemCount = ARRAY_SIZE(dac_channel_configuration_menu_items), 00767 .headerItem = display_dac_channel_configuration_header, 00768 .footerItem = NULL, 00769 .enableEscapeKey = true 00770 }; 00771 00772 /* 00773 * Monitor Setup Menu 00774 */ 00775 static console_menu_item monitor_setup_menu_items[] = { 00776 {"Disable Monitoring", 'Q', do_monitor_setup, AD5770R_DISABLE}, 00777 {"Enable Voltage Monitoring", 'W', do_monitor_setup, AD5770R_VOLTAGE_MONITORING}, 00778 {"Enable Current Monitoring", 'E', do_monitor_setup, AD5770R_CURRENT_MONITORING}, 00779 {"Enable Temperature Monitoring", 'R', do_monitor_setup, AD5770R_TEMPERATURE_MONITORING}, 00780 {"", '\00', NULL}, 00781 {"Toggle Mux Buffer", 'M', do_monitor_setup, TOGGLE_MUX_BUFFER}, 00782 {"Toggle Diode External Bias", 'X', do_monitor_setup, TOGGLE_DIODE_EXT_BIAS}, 00783 {"", '\00', NULL}, 00784 {"\tSelect Channel 0", '0', do_monitor_setup, AD5770R_CH0 + MENU_CHANNEL_OFFSET}, 00785 {"\tSelect Channel 1", '1', do_monitor_setup, AD5770R_CH1 + MENU_CHANNEL_OFFSET}, 00786 {"\tSelect Channel 2", '2', do_monitor_setup, AD5770R_CH2 + MENU_CHANNEL_OFFSET}, 00787 {"\tSelect Channel 3", '3', do_monitor_setup, AD5770R_CH3 + MENU_CHANNEL_OFFSET}, 00788 {"\tSelect Channel 4", '4', do_monitor_setup, AD5770R_CH4 + MENU_CHANNEL_OFFSET}, 00789 {"\tSelect Channel 5", '5', do_monitor_setup, AD5770R_CH5 + MENU_CHANNEL_OFFSET}, 00790 }; 00791 00792 static console_menu monitor_setup_menu = { 00793 .title = "Monitor Setup", 00794 .items = monitor_setup_menu_items, 00795 .itemCount = ARRAY_SIZE(monitor_setup_menu_items), 00796 .headerItem = display_monitor_setup_header, 00797 .footerItem = NULL, 00798 .enableEscapeKey = true 00799 }; 00800 00801 /* 00802 * General Configuration Menu 00803 */ 00804 static console_menu_item general_configuration_menu_items[] = { 00805 {"Select Int/External Reference Resistor", 'R', do_toggle_ref_resistor}, 00806 {""}, 00807 {"Set Ext 2.50V Reference", 'A', do_set_reference, AD5770R_EXT_REF_2_5_V}, 00808 {"Set Int 1.25V Reference, Vout: ON", 'S', do_set_reference, AD5770R_INT_REF_1_25_V_OUT_ON}, 00809 {"Set Ext 1.25V Reference", 'D', do_set_reference, AD5770R_EXT_REF_1_25_V}, 00810 {"Set Int 1.25V Reference, Vout: OFF", 'F', do_set_reference, AD5770R_INT_REF_1_25_V_OUT_OFF}, 00811 {""}, 00812 {" --Toggle Alarm Configuration bits --"}, 00813 {"\tOpen Drain Enable", '0', do_set_alarm, AD5770R_ALARM_CONFIG_OPEN_DRAIN_EN(1)}, 00814 {"\tThermal Shutdown Enable", '1', do_set_alarm, AD5770R_ALARM_CONFIG_THERMAL_SHUTDOWN_EN(1)}, 00815 {"\tBackground CRC Enable", '2', do_set_alarm, AD5770R_ALARM_CONFIG_BACKGROUND_CRC_EN(1)}, 00816 {"\tTemperature Warning Alarm Mask", '3', do_set_alarm, AD5770R_ALARM_CONFIG_TEMP_WARNING_ALARM_MASK(1)}, 00817 {"\tOver Temperature Alarm Mask", '4', do_set_alarm, AD5770R_ALARM_CONFIG_OVER_TEMP_ALARM_MASK(1)}, 00818 {"\tNegative Channel 0 Mask", '5', do_set_alarm, AD5770R_ALARM_CONFIG_NEGATIVE_CHANNEL0_ALARM_MASK(1)}, 00819 {"\tIREF Fault Alarm Mask", '6', do_set_alarm, AD5770R_ALARM_CONFIG_IREF_FAULT_ALARM_MASK(1)}, 00820 {"\tBackground CRC Alarm Mask", '7', do_set_alarm, AD5770R_ALARM_CONFIG_BACKGROUND_CRC_ALARM_MASK(1)}, 00821 }; 00822 00823 static console_menu general_configuration_menu = { 00824 .title = "General Configuration", 00825 .items = general_configuration_menu_items, 00826 .itemCount = ARRAY_SIZE(general_configuration_menu_items), 00827 .headerItem = display_gen_config, 00828 .footerItem = NULL, 00829 .enableEscapeKey = true 00830 }; 00831 00832 /* 00833 * Definition of the Main Menu Items and menu itself 00834 */ 00835 static console_menu_item main_menu_items[] = { 00836 {"Initialize Device to User Configuration", 'I', do_device_init}, 00837 {"Remove Device", 'X', do_device_remove}, 00838 {""}, 00839 {"Do Software Reset", 'R', do_software_reset}, 00840 {""}, 00841 {"General Configuration...", 'G', do_general_configuration_menu}, 00842 {"Monitor Setup...", 'M', do_monitor_setup_menu}, 00843 {""}, 00844 {"DAC Channel Configuration...", 'C', do_dac_channel_configuration_menu}, 00845 {"DAC Operations...", 'D', do_dac_operations_menu}, 00846 }; 00847 00848 console_menu ad5770r_main_menu = { 00849 #if ACTIVE_DEVICE==GENERIC_AD5770R 00850 .title = "AD5770R Console App", 00851 #elif ACTIVE_DEVICE==GENERIC_AD5772R 00852 .title = "AD5772R Terminal App", 00853 #else 00854 #error "Unsupported device" 00855 #endif 00856 .items = main_menu_items, 00857 .itemCount = ARRAY_SIZE(main_menu_items), 00858 .headerItem = display_main_menu_header, 00859 .footerItem = display_main_menu_footer, 00860 .enableEscapeKey = false 00861 };
Generated on Wed Jul 13 2022 14:14:19 by
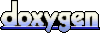