
Example program for EVAL-AD5770R
Dependencies: adi_console_menu platform_drivers
ad5770_user_config.c
00001 /*! 00002 ***************************************************************************** 00003 @file: ad5770_user_config.c 00004 @brief: AD5770R user configuration 00005 @details: 00006 ----------------------------------------------------------------------------- 00007 * 00008 Copyright (c) 2020-2021 Analog Devices, Inc. All Rights Reserved. 00009 00010 This software is proprietary to Analog Devices, Inc. and its licensors. 00011 By using this software you agree to the terms of the associated 00012 Analog Devices Software License Agreement. 00013 ******************************************************************************/ 00014 00015 // #includes 00016 #include <stdint.h> 00017 #include <stdbool.h> 00018 #include <stddef.h> 00019 00020 #include "app_config.h" 00021 00022 #include "spi.h" 00023 #include "spi_extra.h" 00024 00025 #include "ad5770r.h" 00026 00027 00028 // #defines 00029 00030 // type defintions 00031 00032 // constants 00033 struct mbed_spi_init_param spi_init_extra_params = { 00034 .spi_clk_pin = SPI_SCK, 00035 .spi_miso_pin = SPI_MISO, 00036 .spi_mosi_pin = SPI_MOSI 00037 }; 00038 00039 struct ad5770r_init_param ad5770r_user_param = { 00040 /* SPI */ 00041 .spi_init = { 00042 .max_speed_hz = 2500000, 00043 .chip_select = SPI_SS, 00044 .mode = SPI_MODE_0, 00045 .extra = &spi_init_extra_params 00046 }, 00047 00048 /* Device SPI Settings */ 00049 .dev_spi_settings = { 00050 .addr_ascension = false, 00051 .single_instruction = false, 00052 .stream_mode_length = 0 00053 }, 00054 00055 /* Device Settings */ 00056 .channel_config = { 00057 .en0 = true, 00058 .en1 = true, 00059 .en2 = true, 00060 .en3 = true, 00061 .en4 = true, 00062 .en5 = true, 00063 .sink0 = false 00064 }, 00065 00066 .output_mode = { 00067 { 00068 .output_scale = 0x00, 00069 .output_range_mode = 0x00 00070 }, 00071 { 00072 .output_scale = 0x00, 00073 .output_range_mode = 0x00 00074 }, 00075 { 00076 .output_scale = 0x00, 00077 .output_range_mode = 0x00 00078 }, 00079 { 00080 .output_scale = 0x00, 00081 .output_range_mode = 0x00 00082 }, 00083 { 00084 .output_scale = 0x00, 00085 .output_range_mode = 0x00 00086 }, 00087 { 00088 .output_scale = 0x00, 00089 .output_range_mode = 0x00 00090 }, 00091 }, 00092 00093 .external_reference = true, 00094 .reference_selector = AD5770R_EXT_REF_1_25_V, 00095 .alarm_config = { 00096 .open_drain_en = false, 00097 .thermal_shutdown_en = false, 00098 .background_crc_en = false, 00099 .temp_warning_msk = false, 00100 .over_temp_msk = false, 00101 .neg_ch0_msk = false, 00102 .iref_fault_msk = false, 00103 .background_crc_msk = false 00104 }, 00105 .output_filter = { 00106 AD5770R_OUTPUT_FILTER_RESISTOR_60_OHM, 00107 AD5770R_OUTPUT_FILTER_RESISTOR_60_OHM, 00108 AD5770R_OUTPUT_FILTER_RESISTOR_60_OHM, 00109 AD5770R_OUTPUT_FILTER_RESISTOR_60_OHM, 00110 AD5770R_OUTPUT_FILTER_RESISTOR_60_OHM, 00111 AD5770R_OUTPUT_FILTER_RESISTOR_60_OHM 00112 }, 00113 .mon_setup = { 00114 .monitor_function = AD5770R_CURRENT_MONITORING, 00115 .mux_buffer = false, 00116 .ib_ext_en = true, 00117 .monitor_channel = AD5770R_CH3 00118 }, 00119 .mask_hw_ldac = { 00120 .en0 = true, 00121 .en1 = true, 00122 .en2 = true, 00123 .en3 = true, 00124 .en4 = true, 00125 .en5 = true, 00126 }, 00127 .dac_value = {0x0, 0x0, 0x0, 0x0, 0x0, 0x0}, 00128 .page_mask = { 00129 .dac_data_page_mask = 0x0000, 00130 .input_page_mask = 0x0000 00131 }, 00132 .mask_channel_sel = { 00133 .en0 = true, 00134 .en1 = true, 00135 .en2 = true, 00136 .en3 = true, 00137 .en4 = true, 00138 .en5 = true, 00139 }, 00140 .sw_ldac = { 00141 .en0 = true, 00142 .en1 = true, 00143 .en2 = true, 00144 .en3 = true, 00145 .en4 = true, 00146 .en5 = true, 00147 }, 00148 .input_value = {0x1, 0x2, 0x3, 0x4, 0x5, 0x6} 00149 }; 00150 00151 // static variables
Generated on Wed Jul 13 2022 14:14:19 by
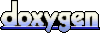