
Example program for EVAL-AD4130
Dependencies: tempsensors sdp_k1_sdram
app_config.c
00001 /***************************************************************************//** 00002 * @file app_config.c 00003 * @brief Application configurations module 00004 * @details This module contains the configurations needed for IIO application 00005 ******************************************************************************** 00006 * Copyright (c) 2020-2022 Analog Devices, Inc. 00007 * 00008 * This software is proprietary to Analog Devices, Inc. and its licensors. 00009 * By using this software you agree to the terms of the associated 00010 * Analog Devices Software License Agreement. 00011 *******************************************************************************/ 00012 00013 /******************************************************************************/ 00014 /***************************** Include Files **********************************/ 00015 /******************************************************************************/ 00016 00017 #include <stdbool.h> 00018 00019 #include "app_config.h" 00020 #include "ad4130_data_capture.h" 00021 #include "no_os_error.h" 00022 #include "no_os_uart.h" 00023 #include "no_os_irq.h" 00024 #include "no_os_gpio.h" 00025 00026 /******************************************************************************/ 00027 /************************ Macros/Constants ************************************/ 00028 /******************************************************************************/ 00029 00030 /******************************************************************************/ 00031 /******************** Variables and User Defined Data Types *******************/ 00032 /******************************************************************************/ 00033 00034 /* UART init parameters */ 00035 struct no_os_uart_init_param uart_init_params = { 00036 .device_id = NULL, 00037 .baud_rate = IIO_UART_BAUD_RATE, 00038 .size = NO_OS_UART_CS_8, 00039 .parity = NO_OS_UART_PAR_NO, 00040 .stop = NO_OS_UART_STOP_1_BIT, 00041 .extra = &uart_extra_init_params 00042 }; 00043 00044 /* SPI initialization parameters */ 00045 struct no_os_spi_init_param spi_init_params = { 00046 .max_speed_hz = 10000000, // Max SPI Speed 00047 .chip_select = SPI_CSB, // Chip Select 00048 .mode = NO_OS_SPI_MODE_3, // CPOL = 1, CPHA = 1 00049 .platform_ops = &spi_ops, 00050 .extra = &spi_extra_init_params // SPI extra configurations 00051 }; 00052 00053 /* External interrupt init parameters */ 00054 static struct no_os_irq_init_param ext_int_init_params = { 00055 .irq_ctrl_id = 0, 00056 .platform_ops = &irq_ops, 00057 .extra = &ext_int_extra_init_params 00058 }; 00059 00060 /* External interrupt callback descriptor */ 00061 static struct no_os_callback_desc ext_int_callback_desc = { 00062 #if (DATA_CAPTURE_MODE == CONTINUOUS_DATA_CAPTURE) 00063 data_capture_callback, 00064 #elif (DATA_CAPTURE_MODE == FIFO_DATA_CAPTURE) 00065 fifo_data_capture_callback, 00066 #else 00067 NULL, 00068 #endif 00069 NULL, 00070 NULL 00071 }; 00072 00073 /* Conversion monitor GPO init parameters */ 00074 static struct no_os_gpio_init_param conv_mon_gpio_init_params = { 00075 .number = CONV_MON, 00076 .platform_ops = &gpio_ops, 00077 .extra = NULL 00078 }; 00079 00080 /* UART descriptor */ 00081 struct no_os_uart_desc *uart_desc; 00082 00083 /* External interrupt descriptor */ 00084 struct no_os_irq_ctrl_desc *external_int_desc; 00085 00086 /* LED GPO descriptor */ 00087 struct no_os_gpio_desc *conv_mon_gpio_desc = NULL; 00088 00089 /******************************************************************************/ 00090 /************************ Functions Prototypes ********************************/ 00091 /******************************************************************************/ 00092 00093 /******************************************************************************/ 00094 /************************ Functions Definitions *******************************/ 00095 /******************************************************************************/ 00096 00097 /** 00098 * @brief Initialize the GPIOs 00099 * @return 0 in case of success, negative error code otherwise 00100 */ 00101 static int32_t init_gpio(void) 00102 { 00103 int32_t ret; 00104 00105 /* Initialize the conversion monitor GPO */ 00106 ret = no_os_gpio_get_optional(&conv_mon_gpio_desc, 00107 &conv_mon_gpio_init_params); 00108 if (ret) { 00109 return ret; 00110 } 00111 00112 ret = no_os_gpio_direction_input(conv_mon_gpio_desc); 00113 if (ret) { 00114 return ret; 00115 } 00116 00117 return 0; 00118 } 00119 00120 /** 00121 * @brief Initialize the UART peripheral 00122 * @return 0 in case of success, negative error code otherwise 00123 */ 00124 static int32_t init_uart(void) 00125 { 00126 return no_os_uart_init(&uart_desc, &uart_init_params); 00127 } 00128 00129 /** 00130 * @brief Initialize the IRQ contoller 00131 * @return 0 in case of success, negative error code otherwise 00132 */ 00133 static int32_t init_interrupt(void) 00134 { 00135 int32_t ret; 00136 00137 #if (DATA_CAPTURE_MODE != BURST_DATA_CAPTURE_MODE) 00138 /* Init interrupt controller for external interrupt (for monitoring 00139 * conversion event on BUSY pin) */ 00140 ext_int_extra_init_params.gpio_irq_pin = CONV_MON; 00141 ret = no_os_irq_ctrl_init(&external_int_desc, &ext_int_init_params); 00142 if (ret) { 00143 return ret; 00144 } 00145 00146 /* Register a callback function for external interrupt */ 00147 ret = no_os_irq_register_callback(external_int_desc, 00148 EXT_INT_ID, 00149 &ext_int_callback_desc); 00150 if (ret) { 00151 return ret; 00152 } 00153 00154 ret = no_os_irq_trigger_level_set(external_int_desc, 00155 EXT_INT_ID, NO_OS_IRQ_EDGE_RISING); 00156 if (ret) { 00157 return ret; 00158 } 00159 00160 /* Enable external interrupt */ 00161 ret = no_os_irq_enable(external_int_desc, EXT_INT_ID); 00162 if (ret) { 00163 return ret; 00164 } 00165 #endif 00166 00167 return 0; 00168 } 00169 00170 /** 00171 * @brief Initialize the system peripherals 00172 * @return 0 in case of success, negative error code otherwise 00173 */ 00174 int32_t init_system(void) 00175 { 00176 int32_t ret; 00177 00178 ret = init_gpio(); 00179 if (ret) { 00180 return ret; 00181 } 00182 00183 ret = init_uart(); 00184 if (ret) { 00185 return ret; 00186 } 00187 00188 ret = init_interrupt(); 00189 if (ret) { 00190 return ret; 00191 } 00192 00193 #if defined(USE_SDRAM_CAPTURE_BUFFER) 00194 ret = sdram_init(); 00195 if (ret) { 00196 return ret; 00197 } 00198 #endif 00199 00200 return 0; 00201 }
Generated on Wed Jul 20 2022 12:42:25 by
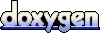