
Example program for EVAL-AD4130
Dependencies: tempsensors sdp_k1_sdram
ad4130_data_capture.c File Reference
AD4130 data capture interface for IIO based applications. More...
Go to the source code of this file.
Functions | |
int32_t | ad4130_data_capture_init (void) |
Function to init the data capture for AD4130 device. | |
static int32_t | adc_store_active_chns (uint32_t chn_mask) |
Store the list of all previously enabled channels and enable new channels set in the channel mask argument. | |
static int32_t | adc_start_data_capture (void) |
Trigger a data capture in continuous/burst mode. | |
static int32_t | adc_stop_data_capture (void) |
Stop a data capture from continuous/burst/fifo mode. | |
static int32_t | adc_start_fifo_data_capture (void) |
Trigger a data capture in FIFO mode. | |
static int32_t | adc_read_single_sample (uint32_t *adc_raw) |
Read a single sample of ADC. | |
static int32_t | adc_read_fifo (uint32_t *data, uint32_t samples) |
Read a single sample of ADC. | |
static int32_t | adc_read_converted_sample (uint32_t *adc_data, uint8_t input_chn) |
Read ADC raw data for recently sampled channel. | |
int32_t | read_single_sample (uint8_t input_chn, uint32_t *adc_raw) |
Function to read the single ADC sample (raw data) for input channel. | |
static void | reset_data_capture (void) |
Reset the data capture specific variables. | |
int32_t | prepare_data_transfer (uint32_t ch_mask, uint8_t sample_size) |
Function to prepare the data ADC capture for new READBUFF request from IIO client (for active channels) | |
int32_t | end_data_transfer (void) |
Function to stop ADC data capture. | |
static int32_t | buffer_read_operations (uint32_t nb_of_samples) |
Perform buffer read operations to read requested samples. | |
static void | buffer_write_operations (void) |
Perform buffer write operations such as buffer full or empty check, resetting buffer index and pointers, etc. | |
void | data_capture_callback (void *ctx) |
This is an ISR (Interrupt Service Routine) to monitor end of conversion event. | |
void | fifo_data_capture_callback (void *ctx) |
This is an ISR (Interrupt Service Routine) to monitor FIFO data available event. This function is expected to be called asynchronously when data from internal device FIFO is available to read. | |
static int32_t | read_burst_data (int8_t *pbuf, uint32_t nb_of_samples) |
Capture requested number of ADC samples in burst mode. | |
static int32_t | read_fifo_data (int8_t *pbuf, uint32_t nb_of_samples) |
Capture requested number of ADC samples in FIFO mode. | |
static int32_t | read_continuous_conv_data (int8_t **pbuf, uint32_t nb_of_samples) |
Read requested number of ADC samples in continuous mode. | |
int32_t | read_buffered_data (int8_t **pbuf, uint32_t nb_of_bytes) |
Function to read the ADC buffered raw data requested by IIO client. |
Detailed Description
AD4130 data capture interface for IIO based applications.
This module handles the ADC data capturing for IIO client
Copyright (c) 2021-22 Analog Devices, Inc. All rights reserved.
This software is proprietary to Analog Devices, Inc. and its licensors. By using this software you agree to the terms of the associated Analog Devices Software License Agreement.
Definition in file ad4130_data_capture.c.
Function Documentation
int32_t ad4130_data_capture_init | ( | void | ) |
Function to init the data capture for AD4130 device.
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 119 of file ad4130_data_capture.c.
static int32_t adc_read_converted_sample | ( | uint32_t * | adc_data, |
uint8_t | input_chn | ||
) | [static] |
Read ADC raw data for recently sampled channel.
- Parameters:
-
adc_data[in,out] - Pointer to adc data read variable input_chn[in] - Input channel (optional)
- Returns:
- 0 in case of success, negative error code otherwise
- Note:
- This function is intended to call from the conversion end trigger event. Therefore, this function should just read raw ADC data without further monitoring conversion end event. Continuous conversion mode is used to for this operation.
Definition at line 308 of file ad4130_data_capture.c.
static int32_t adc_read_fifo | ( | uint32_t * | data, |
uint32_t | samples | ||
) | [static] |
Read a single sample of ADC.
- Parameters:
-
data[in] - Pointer to FIFO data array samples[in] - Number of samples to read
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 289 of file ad4130_data_capture.c.
static int32_t adc_read_single_sample | ( | uint32_t * | adc_raw ) | [static] |
Read a single sample of ADC.
- Parameters:
-
adc_raw[in] - Pointer to ADC raw data variable
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 274 of file ad4130_data_capture.c.
static int32_t adc_start_data_capture | ( | void | ) | [static] |
Trigger a data capture in continuous/burst mode.
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 196 of file ad4130_data_capture.c.
static int32_t adc_start_fifo_data_capture | ( | void | ) | [static] |
Trigger a data capture in FIFO mode.
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 229 of file ad4130_data_capture.c.
static int32_t adc_stop_data_capture | ( | void | ) | [static] |
Stop a data capture from continuous/burst/fifo mode.
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 219 of file ad4130_data_capture.c.
static int32_t adc_store_active_chns | ( | uint32_t | chn_mask ) | [static] |
Store the list of all previously enabled channels and enable new channels set in the channel mask argument.
- Parameters:
-
chn_mask[in] - Active channels list
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 161 of file ad4130_data_capture.c.
static int32_t buffer_read_operations | ( | uint32_t | nb_of_samples ) | [static] |
Perform buffer read operations to read requested samples.
- Parameters:
-
nb_of_samples[in] - Requested number of samples to read
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 461 of file ad4130_data_capture.c.
static void buffer_write_operations | ( | void | ) | [static] |
Perform buffer write operations such as buffer full or empty check, resetting buffer index and pointers, etc.
- Returns:
- none
Definition at line 494 of file ad4130_data_capture.c.
void data_capture_callback | ( | void * | ctx ) |
This is an ISR (Interrupt Service Routine) to monitor end of conversion event.
- Parameters:
-
ctx[in] - Callback context (unused)
- Returns:
- none
This is an Interrupt callback function/ISR invoked in synchronous/asynchronous manner depending upon the application implementation. The conversion results are read into acquisition buffer and control continue to sample next channel. This continues until conversion is stopped (through IIO client command)
Definition at line 533 of file ad4130_data_capture.c.
int32_t end_data_transfer | ( | void | ) |
Function to stop ADC data capture.
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 439 of file ad4130_data_capture.c.
void fifo_data_capture_callback | ( | void * | ctx ) |
This is an ISR (Interrupt Service Routine) to monitor FIFO data available event. This function is expected to be called asynchronously when data from internal device FIFO is available to read.
- Parameters:
-
ctx[in] - Callback context (unused)
- Returns:
- none
Definition at line 580 of file ad4130_data_capture.c.
int32_t prepare_data_transfer | ( | uint32_t | ch_mask, |
uint8_t | sample_size | ||
) |
Function to prepare the data ADC capture for new READBUFF request from IIO client (for active channels)
- Parameters:
-
ch_mask[in] - Channels to enable for data capturing sample_size[in] - Sample size in bytes
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 406 of file ad4130_data_capture.c.
int32_t read_buffered_data | ( | int8_t ** | pbuf, |
uint32_t | nb_of_bytes | ||
) |
Function to read the ADC buffered raw data requested by IIO client.
- Parameters:
-
pbuf[in] - Pointer to data buffer nb_of_bytes[in] - Number of bytes to read
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 758 of file ad4130_data_capture.c.
static int32_t read_burst_data | ( | int8_t * | pbuf, |
uint32_t | nb_of_samples | ||
) | [static] |
Capture requested number of ADC samples in burst mode.
- Parameters:
-
pbuf[out] - Pointer to ADC data buffer nb_of_samples[in] - Number of samples to be read
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 593 of file ad4130_data_capture.c.
static int32_t read_continuous_conv_data | ( | int8_t ** | pbuf, |
uint32_t | nb_of_samples | ||
) | [static] |
Read requested number of ADC samples in continuous mode.
- Parameters:
-
pbuf[in] - Pointer to data buffer nb_of_samples[in] - Number of samples to read
- Returns:
- 0 in case of success, negative error code otherwise
- Note:
- The actual sample capturing happens through interrupt. This function tracks the buffer read pointer to read block of data
Definition at line 708 of file ad4130_data_capture.c.
static int32_t read_fifo_data | ( | int8_t * | pbuf, |
uint32_t | nb_of_samples | ||
) | [static] |
Capture requested number of ADC samples in FIFO mode.
- Parameters:
-
pbuf[in] - Input buffer nb_of_samples[in] - Number of samples to read
- Returns:
- 0 in case of success, negative error code otherwise
Definition at line 637 of file ad4130_data_capture.c.
int32_t read_single_sample | ( | uint8_t | input_chn, |
uint32_t * | adc_raw | ||
) |
Function to read the single ADC sample (raw data) for input channel.
- Parameters:
-
input_chn[in] - Input channel to be sampled and read data for raw_data[in,out]- ADC raw data
- Returns:
- 0 in case of success, negative error code otherwise
- Note:
- The single conversion mode is used to read a single sample
Definition at line 326 of file ad4130_data_capture.c.
static void reset_data_capture | ( | void | ) | [static] |
Reset the data capture specific variables.
- Returns:
- none
Definition at line 381 of file ad4130_data_capture.c.
Generated on Wed Jul 20 2022 12:42:25 by
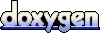