CN0397 (Smart Visible Light Detection)
Dependencies: AD7798
CN0397.h
00001 /** 00002 * @file CN0397.cpp 00003 * @brief Header file for the CN0397 00004 * @author Analog Devices Inc. 00005 * 00006 * For support please go to: 00007 * Github: https://github.com/analogdevicesinc/mbed-adi 00008 * Support: https://ez.analog.com/community/linux-device-drivers/microcontroller-no-os-drivers 00009 * Product: www.analog.com/EVAL-CN0397-ARDZ 00010 * More: https://wiki.analog.com/resources/tools-software/mbed-drivers-all 00011 00012 ******************************************************************************** 00013 * Copyright 2016(c) Analog Devices, Inc. 00014 * 00015 * All rights reserved. 00016 * 00017 * Redistribution and use in source and binary forms, with or without 00018 * modification, are permitted provided that the following conditions are met: 00019 * - Redistributions of source code must retain the above copyright 00020 * notice, this list of conditions and the following disclaimer. 00021 * - Redistributions in binary form must reproduce the above copyright 00022 * notice, this list of conditions and the following disclaimer in 00023 * the documentation and/or other materials provided with the 00024 * distribution. 00025 * - Neither the name of Analog Devices, Inc. nor the names of its 00026 * contributors may be used to endorse or promote products derived 00027 * from this software without specific prior written permission. 00028 * - The use of this software may or may not infringe the patent rights 00029 * of one or more patent holders. This license does not release you 00030 * from the requirement that you obtain separate licenses from these 00031 * patent holders to use this software. 00032 * - Use of the software either in source or binary form, must be run 00033 * on or directly connected to an Analog Devices Inc. component. 00034 * 00035 * THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00036 * IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00037 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00038 * IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00039 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00040 * LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00041 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00042 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00043 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00044 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00045 * 00046 ********************************************************************************/ 00047 00048 #ifndef CN0397_H_ 00049 #define CN0397_H_ 00050 00051 #include <stdio.h> 00052 #include <string.h> 00053 #include "AD7798.h" 00054 00055 #define REGISTERS_VALUES 3 00056 #define CONVERSION_DATA 4 00057 00058 #define V_REF 3150.0 // [mV] 00059 #define _2_16 65535.0 // 2^16 00060 00061 00062 /* Available settings: 00063 * AD7798_CH_AIN1P_AIN1M - select channel 1 00064 * AD7798_CH_AIN2P_AIN2M - select channel 2 00065 * AD7798_CH_AIN3P_AIN3M - select channel 3 00066 */ 00067 #define ADC_CHANNEL AD7798_CH_AIN2P_AIN2M 00068 00069 /* Available settings: 00070 * AD7798_GAIN_1, AD7798_GAIN_2, 00071 * AD7798_GAIN_4, AD7798_GAIN_8, 00072 * AD7798_GAIN_16, AD7798_GAIN_32, 00073 * AD7798_GAIN_64, AD7798_GAIN_128 00074 */ 00075 #define ADC_GAIN AD7798_GAIN_1 00076 /* Available settings: 00077 * Check available value from datasheet 00078 */ 00079 #define ADC_SPS 0x05 /*50SPS*/ 00080 00081 00082 /* Available settings: 00083 * How often to display output values on terminal -> msec 00084 */ 00085 #define DISPLAY_REFRESH 1000 /*[msec]*/ 00086 00087 #define CHANNELS 3 00088 00089 00090 #define USE_CALIBRATION /* Select if you want to use system zero-scale calibration before reading the system data*/ 00091 /** 00092 * Helper function used to flush the serial interface 00093 */ 00094 void flush_serial(); 00095 00096 /** 00097 * @brief The CN0397 shield class 00098 */ 00099 class CN0397 00100 { 00101 public: 00102 /** 00103 * @brief The CN0397 class constructor 00104 */ 00105 CN0397(PinName cs); 00106 /** 00107 * @brief Initialization method of the class. Initializes the AD7798 and initiates calibration if needed 00108 */ 00109 void init(void); 00110 00111 /** 00112 * @brief Displays data on the serial interface 00113 */ 00114 void display_data(void); 00115 00116 /** 00117 * @brief Converts ADC counts to voltage 00118 * @param adcValue - ADC counts 00119 * @param voltage - computed voltage 00120 */ 00121 void data_to_voltage(uint16_t adcValue, float *voltage); 00122 00123 /** 00124 * @brief Computes light intensity of the channel 00125 * @param channel - channel to be converted 00126 * @param adcValue - ADC counts 00127 * @param intensity - computed light intensity 00128 */ 00129 void calc_light_intensity(uint8_t channel, uint16_t adcValue, float *intensity); 00130 00131 /** 00132 * @brief Computes light concentration from light intensity 00133 * @param channel - channel to be converted 00134 * @param intensity - light intensity 00135 * @param conc - computed light concentration 00136 */ 00137 void calc_light_concentration(uint8_t channel, float intensity, float *conc); 00138 00139 /** 00140 * @brief Reads the ADC channels and computes intensity and concentration 00141 */ 00142 void set_app_data(void); 00143 00144 /** 00145 * @brief Calibrates the channel 00146 * @param channel - channel to be calibrated 00147 */ 00148 void calibration(uint8_t channel); 00149 00150 /** 00151 * @brief Instance of the AD7798 00152 */ 00153 AD7798 ad7798; 00154 private: 00155 00156 uint8_t statusReg, idReg, ioReg, gainAdc; 00157 uint16_t modeReg, configReg, offsetReg, fullscaleReg, dataReg; 00158 uint16_t adcValue[3]; 00159 float voltageValue[3], intensityValue[3], lightConcentration[3]; 00160 00161 static const uint8_t Channels[3]; 00162 static const char colour[3][6]; 00163 static const uint8_t ColorPrint[3]; 00164 static const uint8_t Gain[8]; 00165 static const float Lux_LSB[3]; 00166 static const float Optimal_Levels[3]; 00167 00168 }; 00169 00170 #endif /* CN0397_H_ */ 00171
Generated on Mon Jul 18 2022 00:52:12 by
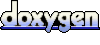