
Prova con controller motore
Dependencies: X-NUCLEO-IHM05A1 mbed
Fork of HelloWorld_IHM05A1 by
Exercise_IHM05A1.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file main.cpp 00004 * @author IPC Rennes 00005 * @version V1.0.0 00006 * @date April 13th, 2016 00007 * @brief mbed simple application for the STMicroelectronics X-NUCLEO-IHM05A1 00008 * Motor Control Expansion Board: control of 1 motor. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 00041 /* mbed specific header files. */ 00042 #include "mbed.h" 00043 00044 /* Component specific header files. */ 00045 #include "L6208.h" 00046 00047 00048 00049 00050 /* Definitions ---------------------------------------------------------------*/ 00051 #ifdef TARGET_NUCLEO_F334R8 00052 #define VREFA_PWM_PIN D11 00053 #define VREFB_PWM_PIN D9 00054 #elif TARGET_NUCLEO_F302R8 00055 #define VREFA_PWM_PIN D11 00056 #define VREFB_PWM_PIN D15 /* HW mandatory patch: bridge manually D9 with D15 */ 00057 #else 00058 #define VREFA_PWM_PIN D3 00059 #define VREFB_PWM_PIN D9 00060 #endif 00061 00062 Serial pc(SERIAL_TX, SERIAL_RX); 00063 00064 /* Variables -----------------------------------------------------------------*/ 00065 00066 /* Initialization parameters of the motor connected to the expansion board. */ 00067 l6208_init_t init = 00068 { 00069 1500, //Acceleration rate in step/s^2 or (1/16)th step/s^2 for microstep modes 00070 40, //Acceleration current torque in % (from 0 to 100) 00071 1500, //Deceleration rate in step/s^2 or (1/16)th step/s^2 for microstep modes 00072 30, //Deceleration current torque in % (from 0 to 100) 00073 1500, //Running speed in step/s or (1/16)th step/s for microstep modes 00074 50, //Running current torque in % (from 0 to 100) 00075 20, //Holding current torque in % (from 0 to 100) 00076 STEP_MODE_1_16, //Step mode via enum motorStepMode_t 00077 FAST_DECAY, //Decay mode via enum motorDecayMode_t 00078 0, //Dwelling time in ms 00079 FALSE, //Automatic HIZ STOP 00080 100000 //VREFA and VREFB PWM frequency (Hz) 00081 }; 00082 00083 /* Motor Control Component. */ 00084 L6208 *motor; 00085 00086 /* Functions -----------------------------------------------------------------*/ 00087 00088 /** 00089 * @brief This is an example of user handler for the flag interrupt. 00090 * @param None 00091 * @retval None 00092 * @note If needed, implement it, and then attach and enable it: 00093 * + motor->attach_flag_irq(&my_flag_irq_handler); 00094 * + motor->enable_flag_irq(); 00095 * To disable it: 00096 * + motor->DisbleFlagIRQ(); 00097 */ 00098 void my_flag_irq_handler(void) 00099 { 00100 pc.printf(" WARNING: \"FLAG\" interrupt triggered:\r\n"); 00101 motor->disable(); 00102 pc.printf(" Motor disabled.\r\n\n"); 00103 } 00104 00105 /** 00106 * @brief This is an example of error handler. 00107 * @param[in] error Number of the error 00108 * @retval None 00109 * @note If needed, implement it, and then attach it: 00110 * + motor->attach_error_handler(&my_error_handler); 00111 */ 00112 void my_error_handler(uint16_t error) 00113 { 00114 /* Printing to the console. */ 00115 pc.printf("Error %d detected\r\n\n", error); 00116 00117 /* Infinite loop */ 00118 while (true) { 00119 } 00120 } 00121 00122 /* Main ----------------------------------------------------------------------*/ 00123 00124 int main() 00125 { 00126 // configura velocità della comunicazione seriale su USB-VirtualCom e invia messaggio di benvenuto 00127 pc.baud(921600); //921600 bps 00128 //pc.baud(9600); //256000 bps 00129 pc.printf("*** Test Motor ***\n\r"); 00130 00131 /* Printing to the console. */ 00132 pc.printf("STARTING MAIN PROGRAM\r\n"); 00133 pc.printf(" Reminder:\r\n"); 00134 pc.printf(" The position unit is in agreement to the step mode.\r\n"); 00135 pc.printf(" The speed, acceleration or deceleration unit depend on the step mode:\r\n"); 00136 pc.printf(" - For normal mode and half step mode, the unit is steps/s or /s^2.\r\n"); 00137 pc.printf(" - For microstep modes, the unit is (1/16)steps/s or /s^2.\r\n"); 00138 00139 //----- Initialization 00140 /* Initializing Motor Control Component. */ 00141 motor = new L6208(D2, D8, D7, D4, D5, D6, VREFA_PWM_PIN, VREFB_PWM_PIN); 00142 if (motor->init(&init) != COMPONENT_OK) { 00143 exit(EXIT_FAILURE); 00144 } 00145 00146 /* Attaching and enabling an interrupt handler. */ 00147 motor->attach_flag_irq(&my_flag_irq_handler); 00148 motor->enable_flag_irq(); 00149 00150 /* Attaching an error handler */ 00151 motor->attach_error_handler(&my_error_handler); 00152 00153 /* Printing to the console. */ 00154 pc.printf("Motor Control Application Example for 1 Motor\r\n"); 00155 00156 //----- run the motor BACKWARD 00157 pc.printf("--> Running the motor backward.\r\n"); 00158 motor->run(StepperMotor::BWD); 00159 00160 while (motor->get_status()!=STEADY) { 00161 /* Print reached speed to the console in step/s or microsteps/s */ 00162 pc.printf(" Reached Speed: %d microstep/s.\r\n", motor->get_speed()); 00163 wait_ms(50); 00164 } 00165 pc.printf(" Reached Speed: %d microstep/s.\r\n", motor->get_speed()); 00166 00167 /* Wait for 1 second */ 00168 wait_ms(1000); 00169 00170 //----- Decrease speed while running to one quarter of the previous speed 00171 motor->set_max_speed(motor->get_speed()>>2); 00172 00173 /* Wait until the motor starts decelerating */ 00174 while (motor->get_status()==STEADY); 00175 /* Wait and print speed while the motor is not steady running */ 00176 while (motor->get_status()!=STEADY) { 00177 /* Print reached speed to the console in step/s or microsteps/s */ 00178 pc.printf(" Reached Speed: %d microstep/s.\r\n", motor->get_speed()); 00179 wait_ms(50); 00180 } 00181 pc.printf(" Reached Speed: %d microstep/s.\r\n", motor->get_speed()); 00182 00183 /* Wait for 5 seconds */ 00184 wait_ms(5000); 00185 00186 //----- Soft stop required while running 00187 pc.printf("--> Soft stop requested.\r\n"); 00188 motor->soft_stop(); 00189 00190 /* Wait for the motor of device ends moving */ 00191 motor->wait_while_active(); 00192 00193 /* Wait for 2 seconds */ 00194 wait_ms(2000); 00195 00196 //----- Change step mode to full step mode 00197 motor->set_step_mode(StepperMotor::STEP_MODE_FULL); 00198 pc.printf(" Motor step mode: %d (0:FS, 1:1/2, 2:1/4, 3:1/8, 4:1/16).\r\n", motor->get_step_mode()); 00199 00200 /* Get current position of device and print to the console */ 00201 pc.printf(" Position: %d.\r\n", motor->get_position()); 00202 00203 /* Set speed, acceleration and deceleration to scale with normal mode */ 00204 motor->set_max_speed(init.maxSpeedSps>>4); 00205 motor->set_acceleration(motor->get_acceleration()>>4); 00206 motor->set_deceleration(motor->get_deceleration()>>4); 00207 /* Print parameters to the console */ 00208 pc.printf(" Motor Max Speed: %d step/s.\r\n", motor->get_max_speed()); 00209 pc.printf(" Motor Min Speed: %d step/s.\r\n", motor->get_min_speed()); 00210 pc.printf(" Motor Acceleration: %d step/s.\r\n", motor->get_acceleration()); 00211 pc.printf(" Motor Deceleration: %d step/s.\r\n", motor->get_deceleration()); 00212 00213 //----- move of 200 steps in the FW direction 00214 pc.printf("--> Moving forward 200 steps.\r\n"); 00215 motor->move(StepperMotor::FWD, 200); 00216 00217 /* Waiting while the motor is active. */ 00218 motor->wait_while_active(); 00219 00220 /* Get current position of device and print to the console */ 00221 pc.printf(" Position: %d.\r\n", motor->get_position()); 00222 00223 /* Disable the power bridges */ 00224 motor->disable(); 00225 00226 /* Check that the power bridges are actually disabled */ 00227 if (motor->check_status_hw()!=0) { 00228 pc.printf(" Motor driver disabled.\r\n"); 00229 } else { 00230 pc.printf(" Failed to disable the motor driver.\r\n"); 00231 } 00232 00233 /* Wait for 2 seconds */ 00234 wait_ms(2000); 00235 00236 //----- Change step mode to 1/4 microstepping mode 00237 motor->set_step_mode(StepperMotor::STEP_MODE_1_4); 00238 pc.printf(" Motor step mode: %d (0:FS, 1:1/2, 2:1/4, 3:1/8, 4:1/16).\r\n", motor->get_step_mode()); 00239 00240 /* Get current position of device and print to the console */ 00241 pc.printf(" Position: %d.\r\n", motor->get_position()); 00242 00243 /* Set speed, acceleration and deceleration to scale with microstep mode */ 00244 motor->set_max_speed(motor->get_max_speed()<<4); 00245 motor->set_acceleration(motor->get_acceleration()<<4); 00246 motor->set_deceleration(motor->get_deceleration()<<4); 00247 /* Print parameters to the console */ 00248 pc.printf(" Motor Max Speed: %d step/s.\r\n", motor->get_max_speed()); 00249 pc.printf(" Motor Min Speed: %d step/s.\r\n", motor->get_min_speed()); 00250 pc.printf(" Motor Acceleration: %d step/s.\r\n", motor->get_acceleration()); 00251 pc.printf(" Motor Deceleration: %d step/s.\r\n", motor->get_deceleration()); 00252 00253 /* Request to go position 800 (quarter steps) */ 00254 motor->go_to(800); 00255 00256 /* Wait for the motor ends moving */ 00257 motor->wait_while_active(); 00258 00259 /* Get current position of device and print to the console */ 00260 pc.printf(" Position: %d.\r\n", motor->get_position()); 00261 00262 /* Wait for 2 seconds */ 00263 wait_ms(2000); 00264 00265 //----- Restore step mode to its initialization value 00266 motor->set_step_mode((StepperMotor::step_mode_t)init.stepMode); 00267 pc.printf(" Motor step mode: %d (0:FS, 1:1/2, 2:1/4, 3:1/8, 4:1/16).\r\n", motor->get_step_mode()); 00268 00269 /* Get current position of device and print to the console */ 00270 pc.printf(" Position: %d.\r\n", motor->get_position()); 00271 00272 //----- Change decay mode 00273 motor->set_decay_mode(SLOW_DECAY); 00274 pc.printf(" Motor decay mode: %d (0:slow decay, 1:fast decay).\r\n", motor->get_decay_mode()); 00275 00276 //----- Go to position -6400 00277 pc.printf("--> Go to position -6400 steps.\r\n"); 00278 motor->go_to(-6400); 00279 00280 /* Wait for the motor ends moving */ 00281 motor->wait_while_active(); 00282 00283 /* Get current position of device and print to the console */ 00284 pc.printf(" Position: %d.\r\n", motor->get_position()); 00285 00286 /* Wait for 2 seconds */ 00287 wait_ms(2000); 00288 00289 //----- Restore decay mode to its initialization value 00290 motor->set_decay_mode(init.decayMode); 00291 pc.printf(" Motor decay mode: %d (0:slow decay, 1:fast decay).\r\n", motor->get_decay_mode()); 00292 00293 //----- Go Home 00294 pc.printf("--> Go to home position.\r\n"); 00295 motor->go_home(); 00296 00297 /* Wait for the motor ends moving */ 00298 motor->wait_while_active(); 00299 00300 /* Wait for 1 second */ 00301 wait_ms(1000); 00302 00303 /* Infinite Loop. */ 00304 pc.printf("--> Infinite Loop...\r\n"); 00305 //while (true) { 00306 int i; 00307 for (i = 0; i < 2; i++) { 00308 /* Request device to go position -3200 */ 00309 motor->go_to(-3200); 00310 00311 /* Waiting while the motor is active. */ 00312 motor->wait_while_active(); 00313 00314 /* Request device to go position 3200 */ 00315 motor->go_to(3200); 00316 00317 /* Waiting while the motor is active. */ 00318 motor->wait_while_active(); 00319 } 00320 } 00321 00322 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 18:33:29 by
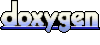