
Debounce and latch a button input
Dependencies: mbed
Debounce.cpp
00001 #include "mbed.h" 00002 00003 Serial pc(USBTX, USBRX); 00004 DigitalIn btn(USER_BUTTON); 00005 DigitalOut led(LED1); 00006 Timer timer; 00007 Timer debounce; 00008 00009 bool state = false; 00010 bool prevState = false; 00011 bool counting = false; 00012 00013 int main() { 00014 while(true) { 00015 state = btn; 00016 if(state != prevState) { 00017 debounce.start(); 00018 } 00019 if(debounce.read_ms() > 20) { 00020 debounce.stop(); 00021 debounce.reset(); 00022 if(!state) { 00023 if(!counting) { 00024 counting = true; 00025 led = true; 00026 timer.start(); 00027 } else { 00028 counting = false; 00029 led = false; 00030 timer.stop(); 00031 pc.printf("%i ms\n", timer.read_ms()); 00032 timer.reset(); 00033 } 00034 } 00035 } 00036 prevState = state; 00037 } 00038 }
Generated on Wed Jul 13 2022 17:21:04 by
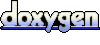