
This is a very basic (hopefully easily understandeable) program to send the data bits of a DHT11 temperature and humidity sensor through Nucleo UART. It is a simple step-by-step guide to build the program.
Dependencies: mbed
Fork of DHT11_with_Nucleo by
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut myled(LED1); // Activate LED 00004 DigitalIn mybutton(USER_BUTTON); // Activate button 00005 DigitalInOut data_pin(A0); // Activate digital in 00006 Serial pc(SERIAL_TX, SERIAL_RX); // Initialize UART connection 00007 // Use a terminal program (eg. TeraTerm). 00008 Timer tmr; //initialize timer 00009 uint64_t adat; // 64 bit variable for temporary data 00010 int i; 00011 00012 // Function to initialize DHT11 00013 void dht_read(void) { 00014 data_pin.output(); // Set A0 as output 00015 // Initialize measurement > 18 ms low 00016 data_pin = 0; 00017 wait_ms(20); 00018 // After high and release the pin switch input mode 00019 data_pin = 1; 00020 data_pin.input(); 00021 // Wait until the end of 80 us low 00022 while(!data_pin.read()) {} 00023 // Wait until end of 80 us high 00024 while(data_pin.read()) {} 00025 // 40 bit, 40 read out cycle 00026 for(i=0; i<40; i++) { 00027 adat = adat << 1; // Shift for new number 00028 tmr.stop(); // Stop timer if runs 00029 tmr.reset(); // Reset timer 00030 // Wait until pin 00031 while(!data_pin.read()) {} 00032 tmr.start(); 00033 while(data_pin.read()) {} 00034 // If DHT11 HIGH longer than 40 micro seconds (hopefully 70 us) 00035 if(tmr.read_us() > 40) { 00036 // bit is 1 00037 adat++; 00038 } 00039 } 00040 } 00041 00042 int main() { 00043 pc.printf("Read the DHT11 temperature and humidity sensor!\n"); //Welcome message 00044 while(1) { 00045 if (mybutton == 0) { // Button is pressed 00046 // Reset adat variable 00047 adat = 0; 00048 myled = 1; // LED is ON 00049 dht_read(); // Call the function 00050 // Send result through UART result 00051 pc.printf("Humidity: "); // Humidity 00052 pc.printf("%d", (adat & 0x000000ff00000000) >> 32); // Humidity 00053 pc.printf("\n\r"); // Send a new line and carriage return. 00054 pc.printf("%d", (adat & 0x0000000000ff0000) >> 16 ); // Temperature 00055 pc.printf("\n\r"); 00056 pc.printf("%d", adat & 0x00000000000000ff); // Checksum. 00057 pc.printf("\n\r"); 00058 wait_ms(200); // Wait 0.2 sec till continue. 00059 } else { 00060 myled = 0; // LED is OFF 00061 } 00062 } 00063 }
Generated on Wed Jul 13 2022 11:53:15 by
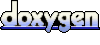