Demo application for using the AT&T IoT Starter Kit Powered by AWS.
Dependencies: SDFileSystem
Fork of ATT_AWS_IoT_demo by
WncControllerK64F.h
00001 /* 00002 Copyright (c) 2016 Fred Kellerman 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 00022 @file WncController.h 00023 @purpose Controls WNC Cellular Modem 00024 @version 1.0 00025 @date July 2016 00026 @author Fred Kellerman 00027 */ 00028 00029 #ifndef __WNCCONTROLLERK64F_H_ 00030 #define __WNCCONTROLLERK64F_H_ 00031 00032 #include <string> 00033 #include <stdint.h> 00034 #include "mbed.h" 00035 #include "MODSERIAL.h" 00036 #include "WncController.h" 00037 00038 namespace WncControllerK64F_fk { 00039 00040 using namespace WncController_fk; 00041 using namespace std; 00042 00043 struct WncGpioPinListK64F { 00044 ///////////////////////////////////////////////////// 00045 // NXP GPIO Pins that are used to initialize the WNC Shield 00046 ///////////////////////////////////////////////////// 00047 DigitalOut * mdm_uart2_rx_boot_mode_sel; // on powerup, 0 = boot mode, 1 = normal boot 00048 DigitalOut * mdm_power_on; // 0 = turn modem on, 1 = turn modem off (should be held high for >5 seconds to cycle modem) 00049 DigitalOut * mdm_wakeup_in; // 0 = let modem sleep, 1 = keep modem awake -- Note: pulled high on shield 00050 DigitalOut * mdm_reset; // active high 00051 DigitalOut * shield_3v3_1v8_sig_trans_ena; // 0 = disabled (all signals high impedence, 1 = translation active 00052 DigitalOut * mdm_uart1_cts; 00053 }; 00054 00055 class WncControllerK64F : public WncController 00056 { 00057 public: 00058 /** 00059 * \brief Constructor for UART controlled WNC 00060 * 00061 * \param [in] wnc_uart - Reference to a SerialBuffered object which will 00062 * be used as the bus to control the WNC. apnStr = a text string for 00063 * the cellular APN name. 00064 * 00065 * \return None. 00066 * 00067 * \details Adding another way to talk to the WNC, like I2C or USB, 00068 * a constructor should be added for each type just like the SerialBuffered 00069 * constructor below. Assumes UART is enabled, setup and ready to go. This 00070 * class will read and write to this UART. 00071 */ 00072 WncControllerK64F(struct WncGpioPinListK64F * pPins, MODSERIAL * wnc_uart, MODSERIAL * debug_uart = NULL); 00073 00074 /** 00075 * \brief Activates a mode where the user can send text to and from the K64F 00076 * debug Serial port directly to the WNC. 00077 * 00078 * \param [in] echoOn - set to true to enable terminal echo 00079 * 00080 * \return true - if terminal mode was successfully entered and exited. 00081 * 00082 * \details Activates a mode where the user can send text to and from the K64F 00083 * debug Serial port directly to the WNC. The mode is entered via this 00084 * call. The mode is exited when the user types CTRL-Q. While in this 00085 * mode all text to and from the WNC is consumed by the debug Serial port. 00086 * No other methods in the class will receive any of the WNC output. 00087 */ 00088 bool enterWncTerminalMode(MODSERIAL *pUart, bool echoOn); 00089 00090 private: 00091 00092 // Disallow copy 00093 // WncControllerK64F operator=(WncControllerK64F lhs); 00094 00095 // Users must define these functionalities: 00096 virtual int putc(char c); 00097 virtual int puts(const char * s); 00098 virtual char getc(void); 00099 virtual int charReady(void); 00100 virtual int dbgWriteChar(char b); 00101 virtual int dbgWriteChars(const char *b); 00102 virtual bool initWncModem(uint8_t powerUpTimeoutSecs); 00103 virtual void waitMs(int t); 00104 virtual void waitUs(int t); 00105 00106 virtual int getLogTimerTicks(void); 00107 virtual void startTimerA(void); 00108 virtual void stopTimerA(void); 00109 virtual int getTimerTicksA_mS(void); 00110 virtual void startTimerB(void); 00111 virtual void stopTimerB(void); 00112 virtual int getTimerTicksB_mS(void); 00113 00114 MODSERIAL * m_pDbgUart; 00115 MODSERIAL * m_pWncUart; 00116 WncGpioPinListK64F m_gpioPinList; 00117 mbed::Timer m_logTimer; 00118 mbed::Timer m_timerA; 00119 mbed::Timer m_timerB; 00120 }; 00121 00122 }; // End namespace WncController_fk 00123 00124 #endif
Generated on Tue Jul 12 2022 22:13:21 by
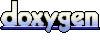