
Retractable steering wheel
Dependencies: DebounceIn EthernetInterface PinDetect mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "PinDetect.h" 00003 #include "DebounceIn.h" 00004 #include "EthernetInterface.h" 00005 00006 #define ECHO_SERVER_PORT 4547 00007 00008 DigitalOut myled(LED1); 00009 00010 //**********FIRGELLI**********// 00011 DigitalOut extend(PTB9); //extends actuator 00012 DigitalOut retract(PTB2); //retracts actuator 00013 //AnalogIn ain(PTC10); //pot wiper (no longer needed) 00014 Timer extend_timer; 00015 Timer retract_timer; 00016 void extend_actuator(); //extends actuator 00017 void retract_actuator(); //retracts actuator 00018 //**********FIRGELLI**********// 00019 00020 //**********SCREW**********// 00021 DigitalOut dir(PTD2); 00022 DigitalOut step(PTD0); 00023 DigitalOut motor_power(PTB3); 00024 DebounceIn switch_top(PTC3); //top limit switch 00025 DebounceIn switch_bottom(PTC2); //bottom limit switch 00026 void motor_power_function(int on_off); //on=1, off=0 00027 void stop_top(); 00028 void stop_bottom(); 00029 void move_up(); 00030 void move_down(); 00031 //**********SCREW**********// 00032 00033 //**********SEAT**********// 00034 //AnalogIn ain(PTC10); //output from potential divider 00035 //bool sitting = false; 00036 //bool seat_state(); //Check state of seat. Returns boolean value for the state of the seat (sitting=true, not sitting=false) 00037 //void person_sitting(); //function called if person sits down 00038 //**********SEAT**********// 00039 00040 //**********SYSTEM**********// 00041 PinDetect reset_switch(PTC16); 00042 //void reset_system(); //resets system in case of fault 00043 //**********SYSTEM**********// 00044 00045 Serial pc(USBTX, USBRX); // tx, rx 00046 00047 //**********FIRGELLI**********// 00048 void extend_actuator() 00049 { 00050 pc.printf("extend\r\n"); 00051 extend_timer.start(); 00052 while(extend_timer.read() < 6) 00053 { 00054 extend = 1; 00055 retract = 0; 00056 } 00057 extend = 0; 00058 retract = 0; 00059 00060 extend_timer.stop(); 00061 extend_timer.reset(); 00062 } 00063 00064 void retract_actuator() 00065 { 00066 pc.printf("retract\r\n"); 00067 retract_timer.start(); 00068 while(retract_timer.read() < 6) 00069 { 00070 extend = 0; 00071 retract = 1; 00072 } 00073 extend = 0; 00074 retract = 0; 00075 00076 retract_timer.stop(); 00077 retract_timer.reset(); 00078 } 00079 //**********FIRGELLI**********// 00080 00081 //**********SEAT**********// 00082 /* 00083 bool seat_state() 00084 { 00085 double sitting_feedback = ain.read(); 00086 // 0.01 < sitting_feedback < 0.99 = person sitting 00087 // 0.01 > sitting_feedback > 0.99 = person not sitting 00088 if(sitting_feedback > 0.99 || sitting_feedback < 0.01) //(problem with occassionally jumping to 0.00....) 00089 { 00090 sitting = false; //no one is sitting, therefore continue as normal 00091 } 00092 else 00093 { 00094 sitting = false; //person is sitting, therefore stop movement of steering wheel 00095 //change to true 00096 } 00097 00098 return sitting; 00099 } 00100 00101 void person_sitting() 00102 { 00103 //stop movement of both actuators and add a wait 00104 pc.printf("person_sitting\r\n"); 00105 step=0; 00106 motor_power_function(0); 00107 wait(3); //delay before starting to move again 00108 } 00109 */ 00110 //**********SEAT**********// 00111 00112 //**********SCREW**********// 00113 void motor_power_function(int on_off) //on=1, off=0 00114 { 00115 pc.printf("motor_power=%i\r\n", on_off); 00116 motor_power = on_off; 00117 } 00118 00119 void stop_top() 00120 { 00121 pc.printf("stop_top\r\n"); 00122 step=0; 00123 motor_power_function(0); 00124 wait(0.5); 00125 extend_actuator(); //extend actuator once the gantry reaches the top 00126 } 00127 00128 void stop_bottom() 00129 { 00130 pc.printf("stop_bottom\r\n"); 00131 step=0; 00132 wait(0.1); 00133 motor_power_function(0); 00134 } 00135 00136 void move_up() 00137 { 00138 pc.printf("move_up\r\n"); 00139 motor_power_function(1); 00140 wait(0.1); 00141 00142 //if (seat_state()==false) //seat free 00143 //{ 00144 while(switch_top != 1) 00145 { 00146 //if (seat_state()==false) //check initial state before moving 00147 //{ 00148 dir=0; //0 is up 00149 step=1; 00150 wait(0.0009); //0.0009 is min. wait 00151 step=0; 00152 wait(0.0009); 00153 //} 00154 //else 00155 //{ 00156 // person_sitting(); //stop movement 00157 //} 00158 } 00159 //} 00160 stop_top(); 00161 } 00162 00163 void move_down() 00164 { 00165 pc.printf("move_down\r\n"); 00166 retract_actuator(); //retract actuator before gantry moves down 00167 motor_power_function(1); 00168 wait(0.1); 00169 00170 //if (seat_state()==false) //seat free 00171 //{ 00172 while(switch_bottom != 1) 00173 { 00174 //if (seat_state()==false) //check initial state before moving 00175 //{ 00176 dir=1; //1 is down 00177 step=1; 00178 wait(0.0009); 00179 step=0; 00180 wait(0.0009); 00181 //} 00182 //} 00183 } 00184 stop_bottom(); 00185 } 00186 00187 //**********SCREW**********// 00188 00189 int main() { 00190 reset_switch.mode(PullDown); 00191 reset_switch.attach_asserted(&NVIC_SystemReset); 00192 reset_switch.setSampleFrequency(); 00193 00194 switch_top.mode(PullDown); 00195 switch_bottom.mode(PullDown); 00196 00197 wait(0.01);//delay for pullups 00198 00199 motor_power_function(0); //start off with motor off 00200 00201 EthernetInterface eth; 00202 eth.init(); //Use DHCP 00203 eth.connect(); 00204 printf("\nServer IP Address is %s\n", eth.getIPAddress()); 00205 00206 UDPSocket server; 00207 server.bind(ECHO_SERVER_PORT); 00208 00209 Endpoint client; 00210 char buffer[256]; 00211 char state_buffer[] = "{\"name\":\"steering\"}"; 00212 int state_buffer_size = strlen(state_buffer); 00213 char deploy_buffer[] = "{\"name\":\"steering\",\"state\":\"deployed\"}"; 00214 int deploy_buffer_size = strlen(deploy_buffer); 00215 char retract_buffer[] = "{\"name\":\"steering\",\"state\":\"retracted\"}"; 00216 int retract_buffer_size = strlen(retract_buffer); 00217 00218 printf("switch_top = %d\r\n", switch_top.read()); 00219 printf("switch_bottom = %d\r\n", switch_bottom.read()); 00220 00221 //initial check to put steering wheel in deployed position 00222 printf("Checking initial state...\r\n"); 00223 printf("Initial state is "); 00224 if (switch_top == 1) 00225 { 00226 printf("deployed\n"); 00227 extend_actuator(); //Already at the top. Extend firgelli if need be 00228 } 00229 else if (switch_bottom == 1) 00230 { 00231 printf("retracted\n"); 00232 move_up(); //move up to top for the starting position 00233 } 00234 else //neither switch is pressed - midway between states 00235 { 00236 printf("midway. Deploying...\n"); 00237 move_up(); //move up to top (reset) 00238 } 00239 00240 while(1) { 00241 myled = !myled; 00242 00243 printf("\nWaiting for UDP packet...\n"); 00244 int n = server.receiveFrom(client, buffer, sizeof(buffer)); 00245 buffer[n] = '\0'; 00246 00247 printf("Received packet from: %s\n", client.get_address()); 00248 printf("Packet contents : '%s'\n",buffer); 00249 00250 if (strcmp(buffer, state_buffer) == 0) //queries current state 00251 { 00252 //return 'deployed' or 'retracted' depending on the limit switches 00253 if (switch_top == 1) 00254 { 00255 printf("Sending state (deployed) packet back to client\n"); 00256 server.sendTo(client, deploy_buffer, deploy_buffer_size); 00257 } 00258 else if (switch_bottom == 1) 00259 { 00260 printf("Sending state (retracted) packet back to client\n"); 00261 server.sendTo(client, retract_buffer, retract_buffer_size); 00262 } 00263 else //nothing 00264 { 00265 } 00266 } 00267 else if (strcmp(buffer, deploy_buffer) == 0) //deploy request 00268 { 00269 move_up(); 00270 printf("Sending deployed packet back to client\n"); 00271 server.sendTo(client, buffer, n); 00272 } 00273 else if (strcmp(buffer, retract_buffer) == 0) //retract request 00274 { 00275 move_down(); 00276 printf("Sending retracted packet back to client\n"); 00277 server.sendTo(client, buffer, n); 00278 } 00279 else //invalid request 00280 { 00281 printf("Invalid request. Error.\n"); 00282 } 00283 } 00284 }
Generated on Tue Jul 12 2022 23:21:05 by
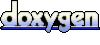