
led example with 2 timers
Embed:
(wiki syntax)
Show/hide line numbers
sender.cpp
00001 #include "main.h" 00002 00003 extern Serial pc; 00004 extern Mail<message_t, 16> mailBox; 00005 DigitalOut myled(LED1); 00006 unsigned int trameSize; 00007 unsigned int trameCounter; 00008 char trameArray[696]; 00009 bool trameReady; 00010 00011 void Sender_init(void) 00012 { 00013 myled = 0; 00014 trameCounter = 1; 00015 trameSize = 0; 00016 trameReady = false; 00017 00018 // Enable the pins on the device to use TIMER MAT3.0 00019 LPC_PINCON->PINSEL0 |= 3<<20; 00020 00021 NVIC_SetVector(TIMER3_IRQn, uint32_t(TIMER3_IRQHandler)); 00022 00023 LPC_SC->PCONP |=1<<23; //timer3 power on 00024 LPC_TIM3->MR0 = TIME; //100 msec ?? 00025 LPC_TIM3->MCR |= 3; //interrupt and reset control 00026 //3 = Interrupt & reset timer3 on match 00027 //1 = Interrupt only, no reset of timer3 00028 LPC_TIM3->TC = 0; // clear timer counter 00029 LPC_TIM3->PC = 0; // clear prescale counter 00030 LPC_TIM3->PR = 0; // clear prescale register 00031 LPC_TIM3->TCR = (1 << 1); //reset Timer3 00032 LPC_TIM3->IR |= 1 << 0; // Clear MR0 interrupt flag 00033 LPC_TIM3->EMR |= (3 << 4); // enable MAT 3.0 00034 00035 00036 NVIC_EnableIRQ(TIMER3_IRQn); //enable timer3 interrupt 00037 00038 } 00039 00040 extern "C" void TIMER3_IRQHandler (void) 00041 { 00042 char current = 0; 00043 char previous = 0; 00044 00045 if((LPC_TIM3->IR & 0x01) == 0x01) // if MR0 interrupt, proceed 00046 { 00047 LPC_TIM3->IR |= 1 << 0; // Clear MR0 interrupt flag 00048 00049 myled =!myled; 00050 00051 if(trameReady == true) 00052 { 00053 00054 if(trameCounter <= trameSize) 00055 { 00056 current = trameArray[trameCounter]; 00057 previous = trameArray[trameCounter-1]; 00058 00059 if(current == previous) 00060 { 00061 LPC_TIM3->MR0 = (TIME/2); 00062 } 00063 else 00064 { 00065 LPC_TIM3->MR0 = (TIME); 00066 } 00067 00068 // next caracter 00069 trameCounter++; 00070 00071 // Activity light 00072 myled =!myled; 00073 } 00074 else 00075 { 00076 // Trame send, wait until a new trame is ready to send 00077 trameReady = false; 00078 LPC_TIM3->TCR = 0; 00079 trameCounter = 1; 00080 myled= 0; 00081 } 00082 } 00083 } 00084 } 00085 00086 void Sender_thread(void const *args) 00087 { 00088 Sender_init(); 00089 00090 while(1) 00091 { 00092 if(trameReady == false) 00093 { 00094 osEvent evt = mailBox.get(); 00095 if (evt.status == osEventMail) 00096 { 00097 message_t *mbTrame = (message_t*)evt.value.p; 00098 trameSize = mbTrame->size; 00099 00100 strcpy(trameArray, mbTrame->trame.c_str()); 00101 mailBox.free(mbTrame); 00102 00103 pc.printf("\n\r%s", trameArray); 00104 pc.printf("\n\r%d", trameSize); 00105 00106 trameReady = true; 00107 LPC_TIM3->TCR = 1; 00108 } 00109 } 00110 } 00111 }
Generated on Wed Jul 27 2022 13:56:09 by
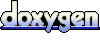