
led example with 2 timers
Embed:
(wiki syntax)
Show/hide line numbers
demodulator.cpp
00001 #include "main.h" 00002 00003 extern Serial pc; 00004 extern Queue<string, 16> WriterQueue; 00005 extern Mail<message_t, 16> mailBox; 00006 message_t gTrame; 00007 string dataTrame; 00008 00009 00010 bool verifyCRC() 00011 { 00012 bool result = false; 00013 00014 dataTrame = ""; 00015 string crcTrame = ""; 00016 dataTrame = gTrame.trame.substr(32, gTrame.size); 00017 crcTrame = gTrame.trame.substr(32+gTrame.size, 16); 00018 00019 char *a = (char*)dataTrame.c_str(); 00020 uint16_t crc = calculate_crc16(a, sizeof(a)); 00021 string crc_s = bitset<16>(crc).to_string<char,string::traits_type,string::allocator_type>(); 00022 00023 if(crc_s == crcTrame) 00024 { 00025 result = true; 00026 } 00027 00028 return result; 00029 //pc.printf("\n\r%s",dataTrame); 00030 //pc.printf("\n\r%s",crcTrame); 00031 } 00032 00033 string rebuildMessage() 00034 { 00035 string message; 00036 int tab[8] = {128, 64, 32, 16, 8, 4, 2, 1}; 00037 int asciiValue = 0; 00038 00039 for(int i=0; i<gTrame.size; i+=OCTET) 00040 { 00041 for(int y=0; y<OCTET; y++) 00042 { 00043 asciiValue += (dataTrame.c_str()[i+y]-48)* tab[y]; 00044 } 00045 message += asciiValue; 00046 asciiValue = 0; 00047 } 00048 00049 return message; 00050 } 00051 00052 void Demodulator_thread(void const *args) 00053 { 00054 while(1) 00055 { 00056 /* osEvent evt = mailBox.get(); 00057 if (evt.status == osEventMail) 00058 { 00059 message_t *mbTrame = (message_t*)evt.value.p; 00060 gTrame.trame = mbTrame->trame; 00061 gTrame.size = mbTrame->size; 00062 mailBox.free(mbTrame); 00063 00064 pc.printf("get in the mail \n\r", gTrame.trame.c_str()); 00065 00066 pc.printf("\n\r%s", mbTrame->trame); 00067 if(verifyCRC()) 00068 { 00069 pc.printf("\n\r crc ok"); 00070 pc.printf("\n\r%s", rebuildMessage()); 00071 //WriterQueue.put(new string(rebuildMessage())); 00072 //pc.printf("put in the queue %s \n\r", gTrame.trame.c_str()); 00073 } 00074 }*/ 00075 } 00076 }
Generated on Wed Jul 27 2022 13:56:09 by
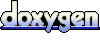