TextLCD library for controlling various LCD panels based on the HD44780 4-bit interface
Dependents: STM32_Button_Interrupt_dla_taty
TextLCD.h
00001 /* mbed TextLCD Library, for LCDs based on HD44780 controllers 00002 * Copyright (c) 2007-2010, sford, http://mbed.org 00003 * 2013, v01: WH, Added LCD types, fixed LCD address issues, added Cursor and UDCs 00004 * 2013, v02: WH, Added I2C and SPI bus interfaces 00005 * 2013, v03: WH, Added support for LCD40x4 which uses 2 controllers 00006 * 2013, v04: WH, Added support for Display On/Off, improved 4bit bootprocess 00007 * 2013, v05: WH, Added support for 8x2B, added some UDCs 00008 * 2013, v06: WH, Added support for devices that use internal DC/DC converters 00009 * 2013, v07: WH, Added support for backlight and include portdefinitions for LCD2004 Module from DFROBOT 00010 * 2014, v08: WH, Refactored in Base and Derived Classes to deal with mbed lib change regarding 'NC' defined DigitalOut pins 00011 * 2014, v09: WH/EO, Added Class for Native SPI controllers such as ST7032 00012 * 2014, v10: WH, Added Class for Native I2C controllers such as ST7032i, Added support for MCP23008 I2C portexpander, Added support for Adafruit module 00013 * 2014, v11: WH, Added support for native I2C controllers such as PCF21XX, Improved the _initCtrl() method to deal with differences between all supported controllers 00014 * 2014, v12: WH, Added support for native I2C controller PCF2119 and native I2C/SPI controllers SSD1803, ST7036, added setContrast method (by JH1PJL) for supported devices (eg ST7032i) 00015 * 2014, v13: WH, Added support for controllers US2066/SSD1311 (OLED), added setUDCBlink() method for supported devices (eg SSD1803), fixed issue in setPower() 00016 * 2014, v14: WH, Added support for PT6314 (VFD), added setOrient() method for supported devices (eg SSD1803, US2066), added Double Height lines for supported devices, 00017 * added 16 UDCs for supported devices (eg PCF2103), moved UDC defines to TextLCD_UDC file, added TextLCD_Config.h for feature and footprint settings. 00018 * 2014, v15: WH, Added AC780 support, added I2C expander modules, fixed setBacklight() for inverted logic modules. Fixed bug in LCD_SPI_N define 00019 * 2014, v16: WH, Added ST7070 and KS0073 support, added setIcon(), clrIcon() and setInvert() method for supported devices 00020 * 2015, v17: WH, Clean up low-level _writeCommand() and _writeData(), Added support for alternative fonttables (eg PCF21XX), Added ST7066_ACM controller for ACM1602 module 00021 * 00022 * Permission is hereby granted, free of charge, to any person obtaining a copy 00023 * of this software and associated documentation files (the "Software"), to deal 00024 * in the Software without restriction, including without limitation the rights 00025 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00026 * copies of the Software, and to permit persons to whom the Software is 00027 * furnished to do so, subject to the following conditions: 00028 * 00029 * The above copyright notice and this permission notice shall be included in 00030 * all copies or substantial portions of the Software. 00031 * 00032 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00033 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00034 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00035 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00036 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00037 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00038 * THE SOFTWARE. 00039 */ 00040 00041 #ifndef MBED_TEXTLCD_H 00042 #define MBED_TEXTLCD_H 00043 00044 #include "mbed.h" 00045 #include "TextLCD_Config.h" 00046 #include "TextLCD_UDC.h" 00047 00048 /** A TextLCD interface for driving 4-bit HD44780-based LCDs 00049 * 00050 * Currently supports 8x1, 8x2, 12x3, 12x4, 16x1, 16x2, 16x3, 16x4, 20x2, 20x4, 24x1, 24x2, 24x4, 40x2 and 40x4 panels. 00051 * Interface options include direct mbed pins, I2C portexpander (PCF8474/PCF8574A or MCP23008) or SPI bus shiftregister (74595). 00052 * Supports some controllers with native I2C or SPI interface. Supports some controllers that provide internal DC/DC converters for VLCD or VLED. 00053 * Supports some controllers that feature programmable contrast control, powerdown, blinking UDCs and/or top/down orientation modes. 00054 * 00055 * @code 00056 * #include "mbed.h" 00057 * #include "TextLCD.h" 00058 * 00059 * // I2C Communication 00060 * I2C i2c_lcd(p28,p27); // SDA, SCL 00061 * 00062 * // SPI Communication 00063 * SPI spi_lcd(p5, NC, p7); // MOSI, MISO, SCLK 00064 * 00065 * //TextLCD lcd(p15, p16, p17, p18, p19, p20); // RS, E, D4-D7, LCDType=LCD16x2, BL=NC, E2=NC, LCDTCtrl=HD44780 00066 * //TextLCD_SPI lcd(&spi_lcd, p8, TextLCD::LCD40x4); // SPI bus, 74595 expander, CS pin, LCD Type 00067 * TextLCD_I2C lcd(&i2c_lcd, 0x42, TextLCD::LCD20x4); // I2C bus, PCF8574 Slaveaddress, LCD Type 00068 * //TextLCD_I2C lcd(&i2c_lcd, 0x42, TextLCD::LCD16x2, TextLCD::WS0010); // I2C bus, PCF8574 Slaveaddress, LCD Type, Device Type (OLED) 00069 * //TextLCD_SPI_N lcd(&spi_lcd, p8, p9); // SPI bus, CS pin, RS pin, LCDType=LCD16x2, BL=NC, LCDTCtrl=ST7032_3V3 00070 * //TextLCD_I2C_N lcd(&i2c_lcd, ST7032_SA, TextLCD::LCD16x2, NC, TextLCD::ST7032_3V3); // I2C bus, Slaveaddress, LCD Type, BL=NC, LCDTCtrl=ST7032_3V3 00071 * //TextLCD_SPI_N_3_24 lcd(&spi_lcd, p8, TextLCD::LCD20x4D, NC, TextLCD::SSD1803_3V3); // SPI bus, CS pin, LCDType=LCD20x4D, BL=NC, LCDTCtrl=SSD1803 00072 * //TextLCD_SPI_N_3_24 lcd(&spi_lcd, p8, TextLCD::LCD20x2, NC, TextLCD::US2066_3V3); // SPI bus, CS pin, LCDType=LCD20x2, BL=NC, LCDTCtrl=US2066 (OLED) 00073 * 00074 * int main() { 00075 * lcd.printf("Hello World!\n"); 00076 * } 00077 * @endcode 00078 */ 00079 00080 //The TextLCD_Config.h file selects hardware interface options to reduce memory footprint 00081 //and provides Pin Defines for I2C PCF8574/PCF8574A or MCP23008 and SPI 74595 bus expander interfaces. 00082 //The LCD and serial portexpanders should be wired accordingly. 00083 00084 /* LCD Type information on Rows, Columns and Variant. This information is encoded in 00085 * an int and used for the LCDType enumerators in order to simplify code maintenance */ 00086 // Columns encoded in b7..b0 00087 #define LCD_T_COL_MSK 0x000000FF 00088 #define LCD_T_C6 0x00000006 00089 #define LCD_T_C8 0x00000008 00090 #define LCD_T_C10 0x0000000A 00091 #define LCD_T_C12 0x0000000C 00092 #define LCD_T_C16 0x00000010 00093 #define LCD_T_C20 0x00000014 00094 #define LCD_T_C24 0x00000018 00095 #define LCD_T_C32 0x00000020 00096 #define LCD_T_C40 0x00000028 00097 00098 // Rows encoded in b15..b8 00099 #define LCD_T_ROW_MSK 0x0000FF00 00100 #define LCD_T_R1 0x00000100 00101 #define LCD_T_R2 0x00000200 00102 #define LCD_T_R3 0x00000300 00103 #define LCD_T_R4 0x00000400 00104 00105 // Addressing mode encoded in b19..b16 00106 #define LCD_T_ADR_MSK 0x000F0000 00107 #define LCD_T_A 0x00000000 /*Mode A Default 1, 2 or 4 line display */ 00108 #define LCD_T_B 0x00010000 /*Mode B, Alternate 8x2 (actually 16x1 display) */ 00109 #define LCD_T_C 0x00020000 /*Mode C, Alternate 16x1 (actually 8x2 display) */ 00110 #define LCD_T_D 0x00030000 /*Mode D, Alternate 3 or 4 line display (12x4, 20x4, 24x4) */ 00111 #define LCD_T_D1 0x00040000 /*Mode D1, Alternate 3 out of 4 line display (12x3, 20x3, 24x3) */ 00112 #define LCD_T_E 0x00050000 /*Mode E, 40x4 display (actually two 40x2) */ 00113 #define LCD_T_F 0x00060000 /*Mode F, 16x3 display (actually 24x2) */ 00114 #define LCD_T_G 0x00070000 /*Mode G, 16x3 display */ 00115 00116 /* LCD Ctrl information on interface support and features. This information is encoded in 00117 * an int and used for the LCDCtrl enumerators in order to simplify code maintenance */ 00118 // Interface encoded in b31..b24 00119 #define LCD_C_BUS_MSK 0xFF000000 00120 #define LCD_C_PAR 0x01000000 /*Parallel 4 or 8 bit data, E pin, RS pin, RW=GND */ 00121 #define LCD_C_SPI3_8 0x02000000 /*SPI 3 line (MOSI, SCL, CS pins), 8 bits (Count Command initiates Data transfer) */ 00122 #define LCD_C_SPI3_9 0x04000000 /*SPI 3 line (MOSI, SCL, CS pins), 9 bits (RS + 8 Data) */ 00123 #define LCD_C_SPI3_10 0x08000000 /*SPI 3 line (MOSI, SCL, CS pins), 10 bits (RS, RW + 8 Data) */ 00124 #define LCD_C_SPI3_16 0x10000000 /*SPI 3 line (MOSI, SCL, CS pins), 16 bits (RS, RW + 8 Data) */ 00125 #define LCD_C_SPI3_24 0x20000000 /*SPI 3 line (MOSI, SCL, CS pins), 24 bits (RS, RW + 8 Data) */ 00126 #define LCD_C_SPI4 0x40000000 /*SPI 4 line (MOSI, SCL, CS, RS pin), RS pin + 8 Data */ 00127 #define LCD_C_I2C 0x80000000 /*I2C (SDA, SCL pin), 8 control bits (Co, RS, RW) + 8 Data */ 00128 // Features encoded in b23..b16 00129 #define LCD_C_FTR_MSK 0x00FF0000 00130 #define LCD_C_BST 0x00010000 /*Booster */ 00131 #define LCD_C_CTR 0x00020000 /*Contrast Control */ 00132 #define LCD_C_ICN 0x00040000 /*Icons */ 00133 #define LCD_C_PDN 0x00080000 /*Power Down */ 00134 // Fonttable encoded in b15..b12 00135 #define LCD_C_FNT_MSK 0x0000F000 00136 #define LCD_C_FT0 0x00000000 /*Default */ 00137 #define LCD_C_FT1 0x00001000 /*Font1 */ 00138 #define LCD_C_FT2 0x00002000 /*Font2 */ 00139 00140 /** A TextLCD interface for driving 4-bit HD44780-based LCDs 00141 * 00142 * @brief Currently supports 8x1, 8x2, 12x2, 12x3, 12x4, 16x1, 16x2, 16x3, 16x4, 20x2, 20x4, 24x2, 24x4, 40x2 and 40x4 panels 00143 * Interface options include direct mbed pins, I2C portexpander (PCF8474/PCF8574A or MCP23008) or 00144 * SPI bus shiftregister (74595) or native I2C or SPI interfaces for some supported devices. 00145 */ 00146 class TextLCD_Base : public Stream { 00147 //class TextLCD_Base { 00148 00149 //Unfortunately the following #define selection breaks Doxygen !!! 00150 //Add it manually when you want to disable the Stream inheritance 00151 //#if (LCD_PRINTF == 1) 00152 //class TextLCD_Base : public Stream { 00153 //#else 00154 //class TextLCD_Base { 00155 //#endif 00156 00157 public: 00158 00159 /** LCD panel format */ 00160 // The commented out types exist but have not yet been tested with the library 00161 enum LCDType { 00162 // LCD6x1 = (LCD_T_A | LCD_T_C6 | LCD_T_R1), /**< 6x1 LCD panel */ 00163 // LCD6x2 = (LCD_T_A | LCD_T_C6 | LCD_T_R2), /**< 6x2 LCD panel */ 00164 LCD8x1 = (LCD_T_A | LCD_T_C8 | LCD_T_R1), /**< 8x1 LCD panel */ 00165 LCD8x2 = (LCD_T_A | LCD_T_C8 | LCD_T_R2), /**< 8x2 LCD panel */ 00166 LCD8x2B = (LCD_T_D | LCD_T_C8 | LCD_T_R2), /**< 8x2 LCD panel (actually 16x1) */ 00167 LCD12x1 = (LCD_T_A | LCD_T_C12 | LCD_T_R1), /**< 12x1 LCD panel */ 00168 LCD12x2 = (LCD_T_A | LCD_T_C12 | LCD_T_R2), /**< 12x2 LCD panel */ 00169 LCD12x3D = (LCD_T_D | LCD_T_C12 | LCD_T_R3), /**< 12x3 LCD panel, special mode PCF21XX, KS0073 */ 00170 LCD12x3D1 = (LCD_T_D1 | LCD_T_C12 | LCD_T_R3), /**< 12x3 LCD panel, special mode PCF21XX, KS0073 */ 00171 // LCD12x3G = (LCD_T_G | LCD_T_C12 | LCD_T_R3), /**< 12x3 LCD panel, special mode ST7036 */ 00172 LCD12x4 = (LCD_T_A | LCD_T_C12 | LCD_T_R4), /**< 12x4 LCD panel */ 00173 LCD12x4D = (LCD_T_B | LCD_T_C12 | LCD_T_R4), /**< 12x4 LCD panel, special mode PCF21XX, KS0073 */ 00174 LCD16x1 = (LCD_T_A | LCD_T_C16 | LCD_T_R1), /**< 16x1 LCD panel */ 00175 LCD16x1C = (LCD_T_C | LCD_T_C16 | LCD_T_R1), /**< 16x1 LCD panel (actually 8x2) */ 00176 LCD16x2 = (LCD_T_A | LCD_T_C16 | LCD_T_R2), /**< 16x2 LCD panel (default) */ 00177 // LCD16x2B = (LCD_T_B | LCD_T_C16 | LCD_T_R2), /**< 16x2 LCD panel, alternate addressing, wrong.. */ 00178 LCD16x3D = (LCD_T_D | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel, special mode SSD1803 */ 00179 // LCD16x3D1 = (LCD_T_D1 | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel, special mode SSD1803 */ 00180 LCD16x3F = (LCD_T_F | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel (actually 24x2) */ 00181 LCD16x3G = (LCD_T_G | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel, special mode ST7036 */ 00182 LCD16x4 = (LCD_T_A | LCD_T_C16 | LCD_T_R4), /**< 16x4 LCD panel */ 00183 // LCD16x4D = (LCD_T_D | LCD_T_C16 | LCD_T_R4), /**< 16x4 LCD panel, special mode SSD1803 */ 00184 LCD20x1 = (LCD_T_A | LCD_T_C20 | LCD_T_R1), /**< 20x1 LCD panel */ 00185 LCD20x2 = (LCD_T_A | LCD_T_C20 | LCD_T_R2), /**< 20x2 LCD panel */ 00186 // LCD20x3 = (LCD_T_A | LCD_T_C20 | LCD_T_R3), /**< 20x3 LCD panel */ 00187 // LCD20x3D = (LCD_T_D | LCD_T_C20 | LCD_T_R3), /**< 20x3 LCD panel, special mode SSD1803 */ 00188 // LCD20x3D1 = (LCD_T_D1 | LCD_T_C20 | LCD_T_R3), /**< 20x3 LCD panel, special mode SSD1803 */ 00189 LCD20x4 = (LCD_T_A | LCD_T_C20 | LCD_T_R4), /**< 20x4 LCD panel */ 00190 LCD20x4D = (LCD_T_D | LCD_T_C20 | LCD_T_R4), /**< 20x4 LCD panel, special mode SSD1803 */ 00191 LCD24x1 = (LCD_T_A | LCD_T_C24 | LCD_T_R1), /**< 24x1 LCD panel */ 00192 LCD24x2 = (LCD_T_A | LCD_T_C24 | LCD_T_R2), /**< 24x2 LCD panel */ 00193 // LCD24x3D = (LCD_T_D | LCD_T_C24 | LCD_T_R3), /**< 24x3 LCD panel */ 00194 // LCD24x3D1 = (LCD_T_D | LCD_T_C24 | LCD_T_R3), /**< 24x3 LCD panel */ 00195 LCD24x4D = (LCD_T_D | LCD_T_C24 | LCD_T_R4), /**< 24x4 LCD panel, special mode KS0078 */ 00196 // LCD32x1 = (LCD_T_A | LCD_T_C32 | LCD_T_R1), /**< 32x1 LCD panel */ 00197 // LCD32x1C = (LCD_T_C | LCD_T_C32 | LCD_T_R1), /**< 32x1 LCD panel (actually 16x2) */ 00198 // LCD32x2 = (LCD_T_A | LCD_T_C32 | LCD_T_R2), /**< 32x2 LCD panel */ 00199 // LCD32x4 = (LCD_T_A | LCD_T_C32 | LCD_T_R4), /**< 32x4 LCD panel */ 00200 // LCD40x1 = (LCD_T_A | LCD_T_C40 | LCD_T_R1), /**< 40x1 LCD panel */ 00201 // LCD40x1C = (LCD_T_C | LCD_T_C40 | LCD_T_R1), /**< 40x1 LCD panel (actually 20x2) */ 00202 LCD40x2 = (LCD_T_A | LCD_T_C40 | LCD_T_R2), /**< 40x2 LCD panel */ 00203 LCD40x4 = (LCD_T_E | LCD_T_C40 | LCD_T_R4) /**< 40x4 LCD panel, Two controller version */ 00204 }; 00205 00206 00207 /** LCD Controller Device */ 00208 enum LCDCtrl { 00209 HD44780 = 0 | LCD_C_PAR, /**< HD44780 or full equivalent (default) */ 00210 AC780 = 1 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_PDN), /**< AC780/KS0066i 4/8 bit, SPI, I2C */ 00211 AIP31068 = 2 | (LCD_C_SPI3_9 | LCD_C_I2C | LCD_C_BST), /**< AIP31068 I2C, SPI3 */ 00212 KS0073 = 3 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_PDN), /**< KS0073 4/8 bit, SPI3 */ 00213 KS0078 = 4 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_PDN), /**< KS0078 24x4 support, 4/8 bit, SPI3 */ 00214 PCF2103_3V3 = 5 | (LCD_C_PAR | LCD_C_I2C), /**< PCF2103 3V3 no Booster, 4/8 bit, I2C */ 00215 PCF2113_3V3 = 6 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< PCF2113 3V3 with Booster, 4/8 bit, I2C */ 00216 PCF2116_3V3 = 7 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST), /**< PCF2116 3V3 with Booster, 4/8 bit, I2C */ 00217 PCF2116_5V = 8 | (LCD_C_PAR | LCD_C_I2C), /**< PCF2116 5V no Booster, 4/8 bit, I2C */ 00218 PCF2116C_5V = 9 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST) | LCD_C_FT1, /**< PCF2116C 3V3 with Booster, 4/8 bit, I2C */ 00219 PCF2119_3V3 = 10 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< PCF2119 3V3 with Booster, 4/8 bit, I2C */ 00220 // PCF2119C_3V3 = 11 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), LCD_C_FT1, /**< PCF2119K 3V3 with Booster, 4/8 bit, I2C */ 00221 // PCF2119_5V = 12 | (LCD_C_PAR | LCD_C_I2C), /**< PCF2119 5V no Booster, 4/8 bit, I2C */ 00222 PT6314 = 13 | (LCD_C_PAR | LCD_C_SPI3_16 | LCD_C_CTR), /**< PT6314 VFD, 4/8 bit, SPI3 */ 00223 SSD1803_3V3 = 14 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR | LCD_C_PDN), /**< SSD1803 3V3 with Booster, 4/8 bit, I2C, SPI3 */ 00224 // SSD1803_5V = 15 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR | LCD_C_PDN), /**< SSD1803 3V3 with Booster, 4/8 bit, I2C, SPI3 */ 00225 ST7032_3V3 = 16 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< ST7032 3V3 with Booster, 4/8 bit, SPI4, I2C */ 00226 ST7032_5V = 17 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_CTR), /**< ST7032 5V no Booster, 4/8 bit, SPI4, I2C */ 00227 ST7036_3V3 = 18 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< ST7036 3V3 with Booster, 4/8 bit, SPI4, I2C */ 00228 ST7036_5V = 19 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< ST7036 5V no Booster, 4/8 bit, SPI4, I2C */ 00229 ST7066_ACM = 20 | (LCD_C_PAR | LCD_C_I2C), /**< ST7066 4/8 bit, I2C on ACM1602 using a PIC */ 00230 ST7070 = 21 | (LCD_C_PAR | LCD_C_SPI3_8 | LCD_C_SPI4), /**< ST7070 4/8 bit, SPI3 */ 00231 US2066_3V3 = 22 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_I2C | LCD_C_CTR | LCD_C_PDN), /**< US2066/SSD1311 3V3, 4/8 bit, I2C, SPI3 */ 00232 WS0010 = 23 | (LCD_C_PAR | LCD_C_SPI3_10 | LCD_C_PDN) /**< WS0010/RS0010 OLED Controller, 4/8 bit, SPI3 */ 00233 // WS0012 = 24 | (LCD_C_PAR | LCD_C_SPI3_10 | LCD_C_I2C | LCD_C_PDN), /**< WS0012 4/8 bit, SPI, I2C */ 00234 00235 }; 00236 00237 00238 /** LCD Cursor control */ 00239 enum LCDCursor { 00240 CurOff_BlkOff = 0x00, /**< Cursor Off, Blinking Char Off */ 00241 CurOn_BlkOff = 0x02, /**< Cursor On, Blinking Char Off */ 00242 CurOff_BlkOn = 0x01, /**< Cursor Off, Blinking Char On */ 00243 CurOn_BlkOn = 0x03 /**< Cursor On, Blinking Char On */ 00244 }; 00245 00246 /** LCD Display control */ 00247 enum LCDMode { 00248 DispOff = 0x00, /**< Display Off */ 00249 DispOn = 0x04 /**< Display On */ 00250 }; 00251 00252 /** LCD Backlight control */ 00253 enum LCDBacklight { 00254 LightOff, /**< Backlight Off */ 00255 LightOn /**< Backlight On */ 00256 }; 00257 00258 /** LCD Blink control (UDC), supported for some Controllers */ 00259 enum LCDBlink { 00260 BlinkOff, /**< Blink Off */ 00261 BlinkOn /**< Blink On */ 00262 }; 00263 00264 /** LCD Orientation control, supported for some Controllers */ 00265 enum LCDOrient { 00266 Top, /**< Top view */ 00267 Bottom /**< Upside down view */ 00268 }; 00269 00270 /** LCD BigFont control, supported for some Controllers */ 00271 enum LCDBigFont { 00272 None, /**< no lines */ 00273 TopLine, /**< 1+2 line */ 00274 CenterLine, /**< 2+3 line */ 00275 BottomLine, /**< 2+3 line or 3+4 line */ 00276 TopBottomLine /**< 1+2 line and 3+4 line */ 00277 }; 00278 00279 00280 /** Convert ASCII character code to the LCD fonttable code 00281 * 00282 * @param c The character to write to the display 00283 * @return The character code for the specific fonttable of the controller 00284 */ 00285 int ASCII_2_LCD (int c); 00286 00287 00288 #if(LCD_PRINTF != 1) 00289 /** Write a character to the LCD 00290 * 00291 * @param c The character to write to the display 00292 */ 00293 int putc(int c); 00294 00295 /** Write a raw string to the LCD 00296 * 00297 * @param string text, may be followed by variables to emulate formatting the string. 00298 * However, printf formatting is NOT supported and variables will be ignored! 00299 */ 00300 int printf(const char* text, ...); 00301 #else 00302 #if DOXYGEN_ONLY 00303 /** Write a character to the LCD 00304 * 00305 * @param c The character to write to the display 00306 */ 00307 int putc(int c); 00308 00309 /** Write a formatted string to the LCD 00310 * 00311 * @param format A printf-style format string, followed by the 00312 * variables to use in formatting the string. 00313 */ 00314 int printf(const char* format, ...); 00315 #endif 00316 00317 #endif 00318 00319 /** Locate cursor to a screen column and row 00320 * 00321 * @param column The horizontal position from the left, indexed from 0 00322 * @param row The vertical position from the top, indexed from 0 00323 */ 00324 void locate(int column, int row); 00325 00326 /** Return the memoryaddress of screen column and row location 00327 * 00328 * @param column The horizontal position from the left, indexed from 0 00329 * @param row The vertical position from the top, indexed from 0 00330 * @return The memoryaddress of screen column and row location 00331 */ 00332 int getAddress(int column, int row); 00333 00334 /** Set the memoryaddress of screen column and row location 00335 * 00336 * @param column The horizontal position from the left, indexed from 0 00337 * @param row The vertical position from the top, indexed from 0 00338 */ 00339 void setAddress(int column, int row); 00340 00341 /** Clear the screen and locate to 0,0 00342 */ 00343 void cls(); 00344 00345 /** Return the number of rows 00346 * 00347 * @return The number of rows 00348 */ 00349 int rows(); 00350 00351 /** Return the number of columns 00352 * 00353 * @return The number of columns 00354 */ 00355 int columns(); 00356 00357 /** Set the Cursormode 00358 * 00359 * @param cursorMode The Cursor mode (CurOff_BlkOff, CurOn_BlkOff, CurOff_BlkOn, CurOn_BlkOn) 00360 */ 00361 void setCursor(LCDCursor cursorMode); 00362 00363 /** Set the Displaymode 00364 * 00365 * @param displayMode The Display mode (DispOff, DispOn) 00366 */ 00367 void setMode(LCDMode displayMode); 00368 00369 /** Set the Backlight mode 00370 * 00371 * @param backlightMode The Backlight mode (LightOff, LightOn) 00372 */ 00373 void setBacklight(LCDBacklight backlightMode); 00374 00375 /** Set User Defined Characters (UDC) 00376 * 00377 * @param unsigned char c The Index of the UDC (0..7) for HD44780 clones and (0..15) for some more advanced controllers 00378 * @param char *udc_data The bitpatterns for the UDC (8 bytes of 5 significant bits for bitpattern and 3 bits for blinkmode (advanced types)) 00379 */ 00380 void setUDC(unsigned char c, char *udc_data); 00381 00382 /** Set UDC Blink and Icon blink 00383 * setUDCBlink method is supported by some compatible devices (eg SSD1803) 00384 * 00385 * @param blinkMode The Blink mode (BlinkOff, BlinkOn) 00386 */ 00387 void setUDCBlink(LCDBlink blinkMode); 00388 00389 /** Set Contrast 00390 * setContrast method is supported by some compatible devices (eg ST7032i) that have onboard LCD voltage generation 00391 * Code imported from fork by JH1PJL 00392 * 00393 * @param unsigned char c contrast data (6 significant bits, valid range 0..63, Value 0 will disable the Vgen) 00394 * @return none 00395 */ 00396 void setContrast(unsigned char c = LCD_DEF_CONTRAST); 00397 00398 /** Set Power 00399 * setPower method is supported by some compatible devices (eg SSD1803) that have power down modes 00400 * 00401 * @param bool powerOn Power on/off 00402 * @return none 00403 */ 00404 void setPower(bool powerOn = true); 00405 00406 /** Set Orient 00407 * setOrient method is supported by some compatible devices (eg SSD1803, US2066) that have top/bottom view modes 00408 * 00409 * @param LCDOrient orient Orientation 00410 * @return none 00411 */ 00412 void setOrient(LCDOrient orient = Top); 00413 00414 /** Set Big Font 00415 * setBigFont method is supported by some compatible devices (eg SSD1803, US2066) 00416 * 00417 * @param lines The selected Big Font lines (None, TopLine, CenterLine, BottomLine, TopBottomLine) 00418 * Double height characters can be shown on lines 1+2, 2+3, 3+4 or 1+2 and 3+4 00419 * Valid double height lines depend on the LCDs number of rows. 00420 */ 00421 void setBigFont(LCDBigFont lines); 00422 00423 /** Set Icons 00424 * 00425 * @param unsigned char idx The Index of the icon pattern (0..15) for KS0073 and similar controllers 00426 * and Index (0..31) for PCF2103 and similar controllers 00427 * @param unsigned char data The bitpattern for the icons (6 lsb for KS0073 bitpattern (5 lsb for KS0078) and 2 msb for blinkmode) 00428 * The bitpattern for the PCF2103 icons is 5 lsb (UDC 0..2) and 5 lsb for blinkmode (UDC 4..6) 00429 */ 00430 void setIcon(unsigned char idx, unsigned char data); 00431 00432 /** Clear Icons 00433 * 00434 * @param none 00435 * @return none 00436 */ 00437 //@TODO Add support for 40x4 dual controller 00438 void clrIcon(); 00439 00440 /** Set Invert 00441 * setInvert method is supported by some compatible devices (eg KS0073) to swap between black and white 00442 * 00443 * @param bool invertOn Invert on/off 00444 * @return none 00445 */ 00446 //@TODO Add support for 40x4 dual controller 00447 void setInvert(bool invertOn); 00448 00449 protected: 00450 00451 /** LCD controller select, mainly used for LCD40x4 00452 */ 00453 enum _LCDCtrl_Idx { 00454 _LCDCtrl_0, /*< Primary */ 00455 _LCDCtrl_1, /*< Secondary */ 00456 }; 00457 00458 /** LCD Datalength control to select between 4 or 8 bit data/commands, mainly used for native Serial interface */ 00459 enum _LCDDatalength { 00460 _LCD_DL_4 = 0x00, /**< Datalength 4 bit */ 00461 _LCD_DL_8 = 0x10 /**< Datalength 8 bit */ 00462 }; 00463 00464 /** Create a TextLCD_Base interface 00465 * @brief Base class, can not be instantiated 00466 * 00467 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00468 * @param ctrl LCD controller (default = HD44780) 00469 */ 00470 TextLCD_Base(LCDType type = LCD16x2, LCDCtrl ctrl = HD44780); 00471 00472 // Stream implementation functions 00473 virtual int _putc(int value); 00474 virtual int _getc(); 00475 00476 /** Medium level initialisation method for LCD controller 00477 * @param _LCDDatalength dl sets the datalength of data/commands 00478 * @return none 00479 */ 00480 void _init(_LCDDatalength dl = _LCD_DL_4); 00481 00482 /** Low level initialisation method for LCD controller 00483 * Set number of lines, fonttype, no cursor etc 00484 * The controller is accessed in 4-bit parallel mode either directly via mbed pins or through I2C or SPI expander. 00485 * Some controllers also support native I2C or SPI interfaces. 00486 * 00487 * @param _LCDDatalength dl sets the 4 or 8 bit datalength of data/commands. Required for some native serial modes. 00488 * @return none 00489 */ 00490 void _initCtrl(_LCDDatalength dl = _LCD_DL_4); 00491 00492 /** Low level character address set method 00493 */ 00494 int _address(int column, int row); 00495 00496 /** Low level cursor enable or disable method 00497 */ 00498 void _setCursor(LCDCursor show); 00499 00500 /** Low level method to store user defined characters for current controller 00501 * 00502 * @param unsigned char c The Index of the UDC (0..7) for HD44780 clones and (0..15) for some more advanced controllers 00503 * @param char *udc_data The bitpatterns for the UDC (8 bytes of 5 significant bits) 00504 */ 00505 void _setUDC(unsigned char c, char *udc_data); 00506 00507 /** Low level method to restore the cursortype and display mode for current controller 00508 */ 00509 void _setCursorAndDisplayMode(LCDMode displayMode, LCDCursor cursorType); 00510 00511 /** Low level nibble write operation to LCD controller (serial or parallel) 00512 */ 00513 void _writeNibble(int value); 00514 00515 /** Low level command byte write operation to LCD controller. 00516 * Methods resets the RS bit and provides the required timing for the command. 00517 */ 00518 void _writeCommand(int command); 00519 00520 /** Low level data byte write operation to LCD controller (serial or parallel). 00521 * Methods sets the RS bit and provides the required timing for the data. 00522 */ 00523 void _writeData(int data); 00524 00525 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00526 * Set the Enable pin. 00527 */ 00528 virtual void _setEnable(bool value) = 0; 00529 00530 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00531 * Set the RS pin ( 0 = Command, 1 = Data). 00532 */ 00533 virtual void _setRS(bool value) = 0; 00534 00535 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00536 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00537 */ 00538 virtual void _setBL(bool value) = 0; 00539 00540 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00541 * Set the databus value (4 bit). 00542 */ 00543 virtual void _setData(int value) = 0; 00544 00545 /** Low level byte write operation to LCD controller (serial or parallel) 00546 * Depending on the RS pin this byte will be interpreted as data or command 00547 */ 00548 virtual void _writeByte(int value); 00549 00550 //Display type 00551 LCDType _type; // Display type 00552 int _nr_cols; 00553 int _nr_rows; 00554 int _addr_mode; // Addressing mode of LCDType, defines relation between display row,col and controller memory address 00555 00556 //Display mode 00557 LCDMode _currentMode; 00558 00559 //Controller type 00560 LCDCtrl _ctrl; // Controller type 00561 int _font; // ASCII character fonttable 00562 00563 //Controller select, mainly used for LCD40x4 00564 _LCDCtrl_Idx _ctrl_idx; 00565 00566 // Cursor 00567 int _column; 00568 int _row; 00569 LCDCursor _currentCursor; 00570 00571 // Function modes saved to allow switch between Instruction sets after initialisation time 00572 int _function, _function_1, _function_x; 00573 00574 // Icon, Booster mode and contrast saved to allow contrast change at later time 00575 // Only available for controllers with added features 00576 int _icon_power, _contrast; 00577 }; 00578 00579 //--------- End TextLCD_Base ----------- 00580 00581 00582 //--------- Start TextLCD Bus ----------- 00583 00584 /** Create a TextLCD interface for using regular mbed pins 00585 * 00586 */ 00587 class TextLCD : public TextLCD_Base { 00588 public: 00589 /** Create a TextLCD interface for using regular mbed pins 00590 * 00591 * @param rs Instruction/data control line 00592 * @param e Enable line (clock) 00593 * @param d4-d7 Data lines for using as a 4-bit interface 00594 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00595 * @param bl Backlight control line (optional, default = NC) 00596 * @param e2 Enable2 line (clock for second controller, LCD40x4 only) 00597 * @param ctrl LCD controller (default = HD44780) 00598 */ 00599 TextLCD(PinName rs, PinName e, PinName d4, PinName d5, PinName d6, PinName d7, LCDType type = LCD16x2, PinName bl = NC, PinName e2 = NC, LCDCtrl ctrl = HD44780); 00600 00601 /** Destruct a TextLCD interface for using regular mbed pins 00602 * 00603 * @param none 00604 * @return none 00605 */ 00606 virtual ~TextLCD(); 00607 00608 private: 00609 00610 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00611 * Set the Enable pin. 00612 */ 00613 virtual void _setEnable(bool value); 00614 00615 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00616 * Set the RS pin (0 = Command, 1 = Data). 00617 */ 00618 virtual void _setRS(bool value); 00619 00620 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00621 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00622 */ 00623 virtual void _setBL(bool value); 00624 00625 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00626 * Set the databus value (4 bit). 00627 */ 00628 virtual void _setData(int value); 00629 00630 /** Regular mbed pins bus 00631 */ 00632 DigitalOut _rs, _e; 00633 BusOut _d; 00634 00635 /** Optional Hardware pins for the Backlight and LCD40x4 device 00636 * Default PinName value is NC, must be used as pointer to avoid issues with mbed lib and DigitalOut pins 00637 */ 00638 DigitalOut *_bl, *_e2; 00639 }; 00640 00641 //----------- End TextLCD --------------- 00642 00643 00644 //--------- Start TextLCD_I2C ----------- 00645 #if(LCD_I2C == 1) /* I2C Expander PCF8574/MCP23008 */ 00646 00647 /** Create a TextLCD interface using an I2C PCF8574 (or PCF8574A) or MCP23008 portexpander 00648 * 00649 */ 00650 class TextLCD_I2C : public TextLCD_Base { 00651 public: 00652 /** Create a TextLCD interface using an I2C PCF8574 (or PCF8574A) or MCP23008 portexpander 00653 * 00654 * @param i2c I2C Bus 00655 * @param deviceAddress I2C slave address (PCF8574 (or PCF8574A) or MCP23008 portexpander, default = PCF8574_SA0 = 0x40) 00656 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00657 * @param ctrl LCD controller (default = HD44780) 00658 */ 00659 TextLCD_I2C(I2C *i2c, char deviceAddress = PCF8574_SA0, LCDType type = LCD16x2, LCDCtrl ctrl = HD44780); 00660 00661 private: 00662 00663 /** Place the Enable bit in the databus shadowvalue 00664 * Used for mbed I2C portexpander 00665 * @param value data to write 00666 * @return none 00667 */ 00668 void _setEnableBit(bool value); 00669 00670 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00671 * Set the Enable pin. 00672 */ 00673 virtual void _setEnable(bool value); 00674 00675 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00676 * Set the RS pin (0 = Command, 1 = Data). 00677 */ 00678 virtual void _setRS(bool value); 00679 00680 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00681 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00682 */ 00683 virtual void _setBL(bool value); 00684 00685 /** Place the 4bit data in the databus shadowvalue 00686 * Used for mbed I2C portexpander 00687 * @param value data to write 00688 * @return none 00689 */ 00690 void _setDataBits(int value); 00691 00692 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00693 * Set the databus value (4 bit). 00694 */ 00695 virtual void _setData(int value); 00696 00697 //New optimized 00698 //Test faster _writeByte 0.11s vs 0.27s for a 20x4 fillscreen (PCF8574) 00699 //Test faster _writeByte 0.14s vs 0.34s for a 20x4 fillscreen (MCP23008) 00700 00701 /** Low level writes to LCD serial bus expander 00702 */ 00703 virtual void _writeByte(int value); 00704 00705 /** Write data to MCP23008 I2C portexpander 00706 * @param reg register to write 00707 * @param value data to write 00708 * @return none 00709 */ 00710 void _writeRegister (int reg, int value); 00711 00712 //I2C bus 00713 I2C *_i2c; 00714 char _slaveAddress; 00715 00716 // Internal bus shadow value for serial bus only 00717 char _lcd_bus; 00718 }; 00719 #endif /* I2C Expander PCF8574/MCP23008 */ 00720 00721 //---------- End TextLCD_I2C ------------ 00722 00723 00724 //--------- Start TextLCD_SPI ----------- 00725 #if(LCD_SPI == 1) /* SPI Expander SN74595 */ 00726 00727 /** Create a TextLCD interface using an SPI 74595 portexpander 00728 * 00729 */ 00730 class TextLCD_SPI : public TextLCD_Base { 00731 public: 00732 /** Create a TextLCD interface using an SPI 74595 portexpander 00733 * 00734 * @param spi SPI Bus 00735 * @param cs chip select pin (active low) 00736 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00737 * @param ctrl LCD controller (default = HD44780) 00738 */ 00739 TextLCD_SPI(SPI *spi, PinName cs, LCDType type = LCD16x2, LCDCtrl ctrl = HD44780); 00740 00741 private: 00742 00743 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00744 * Set the Enable pin. 00745 */ 00746 virtual void _setEnable(bool value); 00747 00748 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00749 * Set the RS pin (0 = Command, 1 = Data). 00750 */ 00751 virtual void _setRS(bool value); 00752 00753 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00754 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00755 */ 00756 virtual void _setBL(bool value); 00757 00758 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00759 * Set the databus value (4 bit). 00760 */ 00761 virtual void _setData(int value); 00762 00763 // SPI bus 00764 SPI *_spi; 00765 DigitalOut _cs; 00766 00767 // Internal bus shadow value for serial bus only 00768 char _lcd_bus; 00769 }; 00770 #endif /* SPI Expander SN74595 */ 00771 //---------- End TextLCD_SPI ------------ 00772 00773 00774 //--------- Start TextLCD_I2C_N ----------- 00775 #if(LCD_I2C_N == 1) /* Native I2C */ 00776 00777 /** Create a TextLCD interface using a controller with native I2C interface 00778 * 00779 */ 00780 class TextLCD_I2C_N : public TextLCD_Base { 00781 public: 00782 /** Create a TextLCD interface using a controller with native I2C interface 00783 * 00784 * @param i2c I2C Bus 00785 * @param deviceAddress I2C slave address (default = ST7032_SA = 0x7C) 00786 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00787 * @param bl Backlight control line (optional, default = NC) 00788 * @param ctrl LCD controller (default = ST7032_3V3) 00789 */ 00790 TextLCD_I2C_N(I2C *i2c, char deviceAddress = ST7032_SA, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = ST7032_3V3); 00791 00792 /** Destruct a TextLCD interface using a controller with native I2C interface 00793 */ 00794 virtual ~TextLCD_I2C_N(void); 00795 00796 private: 00797 00798 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00799 * Set the Enable pin. 00800 */ 00801 virtual void _setEnable(bool value); 00802 00803 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00804 * Set the RS pin ( 0 = Command, 1 = Data). 00805 */ 00806 virtual void _setRS(bool value); 00807 00808 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00809 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00810 */ 00811 virtual void _setBL(bool value); 00812 00813 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00814 * Set the databus value (4 bit). 00815 */ 00816 virtual void _setData(int value); 00817 00818 /** Low level writes to LCD serial bus only (serial native) 00819 */ 00820 virtual void _writeByte(int value); 00821 00822 //I2C bus 00823 I2C *_i2c; 00824 char _slaveAddress; 00825 00826 // controlbyte to select between data and command. Internal shadow value for serial bus only 00827 char _controlbyte; 00828 00829 //Backlight 00830 DigitalOut *_bl; 00831 00832 }; 00833 #endif /* Native I2C */ 00834 //---------- End TextLCD_I2C_N ------------ 00835 00836 00837 //--------- Start TextLCD_SPI_N ----------- 00838 #if(LCD_SPI_N == 1) /* Native SPI bus */ 00839 00840 /** Create a TextLCD interface using a controller with native SPI4 interface 00841 * 00842 */ 00843 class TextLCD_SPI_N : public TextLCD_Base { 00844 public: 00845 /** Create a TextLCD interface using a controller with native SPI4 interface 00846 * 00847 * @param spi SPI Bus 00848 * @param cs chip select pin (active low) 00849 * @param rs Instruction/data control line 00850 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00851 * @param bl Backlight control line (optional, default = NC) 00852 * @param ctrl LCD controller (default = ST7032_3V3) 00853 */ 00854 TextLCD_SPI_N(SPI *spi, PinName cs, PinName rs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = ST7032_3V3); 00855 00856 /** Destruct a TextLCD interface using a controller with native SPI4 interface 00857 */ 00858 virtual ~TextLCD_SPI_N(void); 00859 00860 private: 00861 00862 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00863 * Set the Enable pin. 00864 */ 00865 virtual void _setEnable(bool value); 00866 00867 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00868 * Set the RS pin (0 = Command, 1 = Data). 00869 */ 00870 virtual void _setRS(bool value); 00871 00872 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00873 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00874 */ 00875 virtual void _setBL(bool value); 00876 00877 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00878 * Set the databus value (4 bit). 00879 */ 00880 virtual void _setData(int value); 00881 00882 /** Low level writes to LCD serial bus only (serial native) 00883 */ 00884 virtual void _writeByte(int value); 00885 00886 // SPI bus 00887 SPI *_spi; 00888 DigitalOut _cs; 00889 DigitalOut _rs; 00890 00891 //Backlight 00892 DigitalOut *_bl; 00893 }; 00894 #endif /* Native SPI bus */ 00895 //---------- End TextLCD_SPI_N ------------ 00896 00897 00898 //-------- Start TextLCD_SPI_N_3_8 -------- 00899 #if(LCD_SPI_N_3_8 == 1) /* Native SPI bus */ 00900 /** Create a TextLCD interface using a controller with native SPI3 8 bits interface 00901 * This mode is supported by ST7070. 00902 * 00903 */ 00904 class TextLCD_SPI_N_3_8 : public TextLCD_Base { 00905 public: 00906 /** Create a TextLCD interface using a controller with a native SPI3 8 bits interface 00907 * This mode is supported by ST7070. Note that implementation in TexTLCD is not very efficient due to 00908 * structure of the TextLCD library: each databyte is written separately and requires a separate 'count command' set to 1 byte. 00909 * 00910 * @param spi SPI Bus 00911 * @param cs chip select pin (active low) 00912 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00913 * @param bl Backlight control line (optional, default = NC) 00914 * @param ctrl LCD controller (default = ST7070) 00915 */ 00916 TextLCD_SPI_N_3_8(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = ST7070); 00917 00918 /** Destruct a TextLCD interface using a controller with native SPI3_8 interface 00919 */ 00920 virtual ~TextLCD_SPI_N_3_8(void); 00921 00922 private: 00923 00924 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00925 * Set the Enable pin. 00926 */ 00927 virtual void _setEnable(bool value); 00928 00929 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00930 * Set the RS pin (0 = Command, 1 = Data). 00931 */ 00932 virtual void _setRS(bool value); 00933 00934 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00935 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00936 */ 00937 virtual void _setBL(bool value); 00938 00939 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00940 * Set the databus value (4 bit). 00941 */ 00942 virtual void _setData(int value); 00943 00944 /** Low level writes to LCD serial bus only (serial native) 00945 */ 00946 virtual void _writeByte(int value); 00947 00948 // SPI bus 00949 SPI *_spi; 00950 DigitalOut _cs; 00951 00952 // controlbyte to select between data and command. Internal shadow value for serial bus only 00953 char _controlbyte; 00954 00955 //Backlight 00956 DigitalOut *_bl; 00957 }; 00958 00959 #endif /* Native SPI bus */ 00960 //------- End TextLCD_SPI_N_3_8 ----------- 00961 00962 00963 //------- Start TextLCD_SPI_N_3_9 --------- 00964 #if(LCD_SPI_N_3_9 == 1) /* Native SPI bus */ 00965 //Code checked out on logic analyser. Not yet tested on hardware.. 00966 00967 /** Create a TextLCD interface using a controller with native SPI3 9 bits interface 00968 * Note: current mbed libs only support SPI 9 bit mode for NXP platforms 00969 * 00970 */ 00971 class TextLCD_SPI_N_3_9 : public TextLCD_Base { 00972 public: 00973 /** Create a TextLCD interface using a controller with native SPI3 9 bits interface 00974 * Note: current mbed libs only support SPI 9 bit mode for NXP platforms 00975 * 00976 * @param spi SPI Bus 00977 * @param cs chip select pin (active low) 00978 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00979 * @param bl Backlight control line (optional, default = NC) 00980 * @param ctrl LCD controller (default = AIP31068) 00981 */ 00982 TextLCD_SPI_N_3_9(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = AIP31068); 00983 00984 /** Destruct a TextLCD interface using a controller with native SPI3_9 interface 00985 */ 00986 virtual ~TextLCD_SPI_N_3_9(void); 00987 00988 private: 00989 00990 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00991 * Set the Enable pin. 00992 */ 00993 virtual void _setEnable(bool value); 00994 00995 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00996 * Set the RS pin (0 = Command, 1 = Data). 00997 */ 00998 virtual void _setRS(bool value); 00999 01000 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01001 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 01002 */ 01003 virtual void _setBL(bool value); 01004 01005 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01006 * Set the databus value (4 bit). 01007 */ 01008 virtual void _setData(int value); 01009 01010 /** Low level writes to LCD serial bus only (serial native) 01011 */ 01012 virtual void _writeByte(int value); 01013 01014 // SPI bus 01015 SPI *_spi; 01016 DigitalOut _cs; 01017 01018 // controlbyte to select between data and command. Internal shadow value for serial bus only 01019 char _controlbyte; 01020 01021 //Backlight 01022 DigitalOut *_bl; 01023 }; 01024 #endif /* Native SPI bus */ 01025 //-------- End TextLCD_SPI_N_3_9 ---------- 01026 01027 01028 //------- Start TextLCD_SPI_N_3_10 --------- 01029 #if(LCD_SPI_N_3_10 == 1) /* Native SPI bus */ 01030 01031 /** Create a TextLCD interface using a controller with native SPI3 10 bits interface 01032 * Note: current mbed libs only support SPI 10 bit mode for NXP platforms 01033 * 01034 */ 01035 class TextLCD_SPI_N_3_10 : public TextLCD_Base { 01036 public: 01037 /** Create a TextLCD interface using a controller with native SPI3 10 bits interface 01038 * Note: current mbed libs only support SPI 10 bit mode for NXP platforms 01039 * 01040 * @param spi SPI Bus 01041 * @param cs chip select pin (active low) 01042 * @param type Sets the panel size/addressing mode (default = LCD16x2) 01043 * @param bl Backlight control line (optional, default = NC) 01044 * @param ctrl LCD controller (default = AIP31068) 01045 */ 01046 TextLCD_SPI_N_3_10(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = AIP31068); 01047 01048 /** Destruct a TextLCD interface using a controller with native SPI3_10 interface 01049 */ 01050 virtual ~TextLCD_SPI_N_3_10(void); 01051 01052 private: 01053 01054 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01055 * Set the Enable pin. 01056 */ 01057 virtual void _setEnable(bool value); 01058 01059 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01060 * Set the RS pin (0 = Command, 1 = Data). 01061 */ 01062 virtual void _setRS(bool value); 01063 01064 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01065 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 01066 */ 01067 virtual void _setBL(bool value); 01068 01069 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01070 * Set the databus value (4 bit). 01071 */ 01072 virtual void _setData(int value); 01073 01074 /** Low level writes to LCD serial bus only (serial native) 01075 */ 01076 virtual void _writeByte(int value); 01077 01078 // SPI bus 01079 SPI *_spi; 01080 DigitalOut _cs; 01081 01082 // controlbyte to select between data and command. Internal shadow value for serial bus only 01083 char _controlbyte; 01084 01085 //Backlight 01086 DigitalOut *_bl; 01087 }; 01088 01089 #endif /* Native SPI bus */ 01090 //-------- End TextLCD_SPI_N_3_10 ---------- 01091 01092 01093 //------- Start TextLCD_SPI_N_3_16 --------- 01094 #if(LCD_SPI_N_3_16 == 1) /* Native SPI bus */ 01095 01096 /** Create a TextLCD interface using a controller with native SPI3 16 bits interface 01097 * 01098 */ 01099 class TextLCD_SPI_N_3_16 : public TextLCD_Base { 01100 public: 01101 /** Create a TextLCD interface using a controller with native SPI3 16 bits interface 01102 * 01103 * @param spi SPI Bus 01104 * @param cs chip select pin (active low) 01105 * @param type Sets the panel size/addressing mode (default = LCD16x2) 01106 * @param bl Backlight control line (optional, default = NC) 01107 * @param ctrl LCD controller (default = PT6314) 01108 */ 01109 TextLCD_SPI_N_3_16(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = PT6314); 01110 01111 /** Destruct a TextLCD interface using a controller with native SPI3_16 interface 01112 */ 01113 virtual ~TextLCD_SPI_N_3_16(void); 01114 01115 private: 01116 01117 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01118 * Set the Enable pin. 01119 */ 01120 virtual void _setEnable(bool value); 01121 01122 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01123 * Set the RS pin (0 = Command, 1 = Data). 01124 */ 01125 virtual void _setRS(bool value); 01126 01127 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01128 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 01129 */ 01130 virtual void _setBL(bool value); 01131 01132 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01133 * Set the databus value (4 bit). 01134 */ 01135 virtual void _setData(int value); 01136 01137 /** Low level writes to LCD serial bus only (serial native) 01138 */ 01139 virtual void _writeByte(int value); 01140 01141 // SPI bus 01142 SPI *_spi; 01143 DigitalOut _cs; 01144 01145 // controlbyte to select between data and command. Internal shadow value for serial bus only 01146 char _controlbyte; 01147 01148 //Backlight 01149 DigitalOut *_bl; 01150 }; 01151 #endif /* Native SPI bus */ 01152 //-------- End TextLCD_SPI_N_3_16 ---------- 01153 01154 01155 //------- Start TextLCD_SPI_N_3_24 --------- 01156 #if(LCD_SPI_N_3_24 == 1) /* Native SPI bus */ 01157 01158 /** Create a TextLCD interface using a controller with native SPI3 24 bits interface 01159 * Note: lib uses SPI 8 bit mode 01160 * 01161 */ 01162 class TextLCD_SPI_N_3_24 : public TextLCD_Base { 01163 public: 01164 /** Create a TextLCD interface using a controller with native SPI3 24 bits interface 01165 * Note: lib uses SPI 8 bit mode 01166 * 01167 * @param spi SPI Bus 01168 * @param cs chip select pin (active low) 01169 * @param type Sets the panel size/addressing mode (default = LCD16x2) 01170 * @param bl Backlight control line (optional, default = NC) 01171 * @param ctrl LCD controller (default = SSD1803) 01172 */ 01173 TextLCD_SPI_N_3_24(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = SSD1803_3V3); 01174 01175 /** Destruct a TextLCD interface using a controller with native SPI3_24 interface 01176 */ 01177 virtual ~TextLCD_SPI_N_3_24(void); 01178 01179 private: 01180 01181 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01182 * Set the Enable pin. 01183 */ 01184 virtual void _setEnable(bool value); 01185 01186 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01187 * Set the RS pin (0 = Command, 1 = Data). 01188 */ 01189 virtual void _setRS(bool value); 01190 01191 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01192 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 01193 */ 01194 virtual void _setBL(bool value); 01195 01196 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01197 * Set the databus value (4 bit). 01198 */ 01199 virtual void _setData(int value); 01200 01201 /** Low level writes to LCD serial bus only (serial native) 01202 */ 01203 virtual void _writeByte(int value); 01204 01205 // SPI bus 01206 SPI *_spi; 01207 DigitalOut _cs; 01208 01209 // controlbyte to select between data and command. Internal value for serial bus only 01210 char _controlbyte; 01211 01212 //Backlight 01213 DigitalOut *_bl; 01214 }; 01215 #endif /* Native SPI bus */ 01216 //-------- End TextLCD_SPI_N_3_24 ---------- 01217 01218 #endif
Generated on Thu Jul 14 2022 17:12:56 by
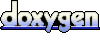