
wave cancel
Dependencies: 4DGL-uLCD-SE EthernetInterface HTTPClient NTPClient PinDetect SDFileSystem mbed-rtos mbed wave_player
Fork of 4180_Final_Project by
main.cpp
00001 //WavePlayer_HelloWorld4180 00002 //internet_clock 00003 00004 #include "mbed.h" 00005 #include "SDFileSystem.h" 00006 #include "wave_player.h" 00007 #include "EthernetInterface.h" 00008 #include "NTPClient.h" 00009 #include "uLCD_4DGL.h" 00010 #include "rtos.h" 00011 #include "PinDetect.h" 00012 #include "HTTPClient.h" 00013 00014 //pinouts 00015 // Graphic LCD - TX, RX, and RES pins 00016 uLCD_4DGL uLCD(p28,p27,p29); 00017 SDFileSystem sd(p11, p12, p13, p14, "sd"); //SD card 00018 Serial pc(USBTX, USBRX); 00019 Serial time_weth(p9, p10); 00020 AnalogOut DACout(p18); 00021 wave_player waver(&DACout); 00022 PinDetect snooze(p19); //snooze button 00023 PinDetect off(p20); //turn alarm off 00024 PinDetect settings(p17); //turn alarm off 00025 DigitalOut myled1(LED1); 00026 DigitalOut myled2(LED2); 00027 DigitalOut myled3(LED3); 00028 //Mutex lcd_mutex; 00029 00030 // Parameters 00031 char* time_domain_name = "0.uk.pool.ntp.org"; 00032 //char* weth_domain_name = "http://weather.yahooapis.com/forecastrss?w=2502265"; 00033 //char* weth_domain_name = "http://developer.mbed.org/media/uploads/mbed_official/hello.txt"; 00034 //char* weth_domain_name = "https://query.yahooapis.com/v1/public/yql?q=select%20item.condition%20from%20weather.forecast%20where%20woeid%20in%20%28select%20woeid%20from%20geo.places%281%29%20where%20text%3D%22atlanta%2C%20ga%22%29&format=json&env=store%3A%2F%2Fdatatables.org%2Falltableswithkeys"; 00035 int port_number = 123; 00036 00037 // Networking 00038 EthernetInterface eth; 00039 #define snoozeTime 420 00040 #define locationLength 50 00041 00042 //global variables 00043 time_t ct_time; 00044 // Base alarm time-24 hour clock 00045 int baseAlarmHour = 0; //0-23 00046 int baseAlarmMin = 0; 00047 // Current alarm time 00048 int curAlarmHour = 18; //0-23 00049 int curAlarmMin = 9; 00050 bool play = true; 00051 //traffic 00052 char start_add[locationLength]; // start location address 00053 char dest_add[locationLength]; // destination address 00054 int trip_sec = 47400; 00055 int arrival_hour; 00056 int arrival_min; 00057 int ready_time = 0; 00058 00059 00060 //time thread 00061 void time_thread(void const *args) 00062 { 00063 char time_buffer[80]; 00064 // Loop and update clock 00065 while (1) { 00066 //lcd_mutex.lock(); 00067 uLCD.locate(0, 1); 00068 ct_time = time(NULL); 00069 strftime(time_buffer, 80, " %a %b %d\n %T %p %z\n %Z\n", \ 00070 localtime(&ct_time)); 00071 uLCD.printf(" UTC/GMT:\n%s", time_buffer); 00072 //lcd_mutex.unlock(); 00073 Thread::wait(100); 00074 } 00075 } 00076 00077 void reset_alarm() 00078 { 00079 int trip_hour = trip_sec/(60*60); 00080 int trip_min = (trip_sec - trip_hour*(60*60))/60; 00081 uLCD.printf("trip %d:%d\n", trip_hour, trip_min); 00082 baseAlarmHour = arrival_hour - trip_hour; 00083 baseAlarmMin = arrival_min - trip_min - ready_time; 00084 while(baseAlarmMin<0) { 00085 baseAlarmHour -= 1; 00086 baseAlarmMin += 60; 00087 } 00088 if(baseAlarmHour<0) { 00089 baseAlarmHour +=24; 00090 } 00091 curAlarmHour = baseAlarmHour; 00092 curAlarmMin = baseAlarmMin; 00093 } 00094 00095 void comm_get() 00096 { 00097 int sum = 0; 00098 bool flag = false; 00099 char url[512]; 00100 char buff[4]; 00101 int i = 0; 00102 sprintf(url, "https://www.mapquestapi.com/directions/v2/route?key=ZoiBJSzSoqfdNiLD0Z9kZdw4uAN5QUQW&from=%s&to=%s", start_add, dest_add); 00103 uLCD.printf("%s^\n",url); 00104 time_weth.printf("%s^",url); 00105 uLCD.printf("Reading travel time"); 00106 while(!flag) { 00107 buff[i] = time_weth.getc(); 00108 //pc.putc(buff[i]); 00109 flag = buff[i] == '^'; 00110 i++; 00111 } 00112 for(i = 0; i < 4; i++) { 00113 sum += int(buff[3-i]-'0') * pow(10.0,i); 00114 } 00115 trip_sec = sum; 00116 uLCD.printf("travel secs %d\n", trip_sec); 00117 wait(10); 00118 } 00119 /* 00120 //communication thread 00121 void comm_thread(void const *args) 00122 { 00123 char buff[4]; 00124 int i; 00125 int sum = 0; 00126 bool flag; 00127 while(true) { 00128 for(i = 0; i < locationLength; i++) { 00129 time_weth.putc(start_add[i]); 00130 } 00131 time_weth.putc('@'); 00132 for(i = 0; i < locationLength; i++) { 00133 time_weth.putc(dest_add[i]); 00134 } 00135 flag = false; 00136 i = 0; 00137 uLCD.printf("send fomplete"); 00138 while(!flag) { 00139 buff[i] = pc.getc(); 00140 //pc.putc(buff[i]); 00141 flag = buff[i] == '^'; 00142 i++; 00143 } 00144 for(i = 0; i < 4; i++) { 00145 sum += int(buff[i]-'0') * pow(10.0,i); 00146 } 00147 trip_sec = sum; 00148 reset_alarm(); 00149 Thread::wait(1800000); 00150 } 00151 } 00152 */ 00153 //pushbutton (p19) 00154 void snooze_hit_callback (void) 00155 { 00156 myled1 = !myled1; 00157 play = false; 00158 time_t newtime; 00159 struct tm * timeinfo; 00160 newtime = ct_time + snoozeTime; 00161 //time (&newtime); 00162 timeinfo = localtime (&newtime); 00163 curAlarmMin = timeinfo->tm_min; 00164 curAlarmHour = timeinfo->tm_hour; 00165 } 00166 00167 void off_hit_callback (void) 00168 { 00169 myled2 = !myled2; 00170 play = false; 00171 curAlarmMin = baseAlarmMin; 00172 curAlarmHour = baseAlarmHour; 00173 } 00174 00175 void settings_hit_callback (void) 00176 { 00177 while(1) { 00178 char buff[100]; 00179 int i = 0; 00180 bool flag = false; 00181 while(!flag) { 00182 buff[i] = pc.getc(); 00183 pc.putc(buff[i]); 00184 flag = buff[i] == '^'; 00185 i++; 00186 } 00187 uLCD.printf("%s", buff); 00188 00189 mkdir("/sd/mydir", 0777); 00190 FILE *fp = fopen("/sd/mydir/sdtest.txt", "w"); 00191 if(fp == NULL) { 00192 error("Could not open file for write\n"); 00193 } 00194 fprintf(fp, buff); 00195 fclose(fp); 00196 } 00197 } 00198 00199 void play_file() 00200 { 00201 bool* play_point = &play; 00202 FILE *wave_file; 00203 printf("\n\n\nHello, wave world!\n"); 00204 wave_file=fopen("/sd/bob.wav","r"); 00205 waver.play(wave_file, play_point); 00206 fclose(wave_file); 00207 } 00208 00209 void timeCompare() 00210 { 00211 struct tm * timeinfo; 00212 timeinfo = localtime (&ct_time); 00213 if (timeinfo->tm_min == curAlarmMin && timeinfo->tm_hour == curAlarmHour) { 00214 play = true; 00215 myled3 = true; 00216 play_file(); 00217 } 00218 } 00219 00220 void getSDInfo() 00221 { 00222 char c; 00223 FILE *fp = fopen("/sd/mydir/sdtest.txt", "r"); 00224 if(fp == NULL) { 00225 error("Could not open file for read\n"); 00226 } 00227 int count = 0; 00228 int i; 00229 char arr_time[8] = ""; 00230 char hour[3], min[3]; 00231 char r_time[8] = ""; 00232 00233 00234 while (!feof(fp)) { // while not end of file 00235 c=fgetc(fp); // get a character/byte from the file 00236 uLCD.printf("%c",c); 00237 if (c == '@') { 00238 count++; // specifies what data (eg. arrival time, ready time, etc) 00239 i = 0; 00240 } else { 00241 //uLCD.printf("%d",count); 00242 switch (count) { 00243 case 0: // arrival time 00244 arr_time[i] = c; 00245 i++; 00246 break; 00247 case 1: // ready time (min) 00248 r_time[i] = c; 00249 i++; 00250 break; 00251 case 2: // start address 00252 start_add[i] = c; 00253 i++; 00254 break; 00255 case 3: // destination address 00256 dest_add[i] = c; 00257 i++; 00258 break; 00259 default: 00260 error("too many & detected\n"); 00261 break; 00262 } 00263 00264 //uLCD.printf("Read from file %02x\n\r",c); // and show it in hex format 00265 } 00266 } 00267 memcpy( hour, &arr_time[0], 2 ); 00268 memcpy( min, &arr_time[3], 2 ); 00269 00270 arrival_hour = atoi(hour); 00271 arrival_min = atoi(min); 00272 uLCD.printf("\narrival hour is %d\n",arrival_hour); 00273 uLCD.printf("arrival min is %d\n",arrival_min); 00274 00275 ready_time = atoi(r_time); 00276 //uLCD.printf("\nString ready time is %s",r_time); 00277 uLCD.printf("Ready Time is %d\n",ready_time); 00278 uLCD.printf("start address is %s\n", start_add); 00279 uLCD.printf("dest address is %s\n", dest_add); 00280 fclose(fp); 00281 } 00282 00283 int main() 00284 { 00285 getSDInfo(); 00286 uLCD.cls(); 00287 comm_get(); 00288 uLCD.cls(); 00289 reset_alarm(); 00290 uLCD.printf("base = %d:%d\ncurr = %d:%d", baseAlarmHour, baseAlarmMin, curAlarmHour, curAlarmMin); 00291 wait(10); 00292 //Thread t2(comm_thread); 00293 snooze.mode(PullUp); 00294 off.mode(PullUp); 00295 settings.mode(PullUp); 00296 wait(0.01); 00297 snooze.attach_deasserted(&snooze_hit_callback); 00298 off.attach_deasserted(&off_hit_callback); 00299 settings.attach_deasserted(&off_hit_callback); 00300 snooze.setSampleFrequency(); 00301 off.setSampleFrequency(); 00302 settings.setSampleFrequency(); 00303 00304 //play_file(); 00305 00306 00307 // Initialize LCD 00308 uLCD.baudrate(115200); 00309 uLCD.background_color(BLACK); 00310 uLCD.cls(); 00311 00312 // Connect to network and wait for DHCP 00313 uLCD.locate(0,0); 00314 uLCD.printf("Getting IP Address\n"); 00315 eth.init(); 00316 if ( eth.connect(60000) == -1 ) { 00317 uLCD.printf("ERROR: Could not\nget IP address"); 00318 return -1; 00319 } 00320 uLCD.printf("IP address is \n%s\n\n",eth.getIPAddress()); 00321 // Read time from server 00322 { 00323 uLCD.printf("Reading time...\n\r"); 00324 NTPClient ntp_client; 00325 ntp_client.setTime(time_domain_name, port_number); 00326 uLCD.printf("Time set\n"); 00327 } 00328 Thread::wait(2000); 00329 eth.disconnect(); 00330 00331 // Reset LCD 00332 uLCD.background_color(WHITE); 00333 uLCD.textbackground_color(WHITE); 00334 uLCD.color(RED); 00335 uLCD.cls(); 00336 uLCD.text_height(2); 00337 00338 Thread t1(time_thread); 00339 while(true) { 00340 timeCompare(); 00341 Thread::wait(100); 00342 } 00343 00344 }
Generated on Fri Jul 15 2022 05:19:22 by
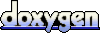