
rev1
Dependencies: 4DGL-uLCD-SE EthernetInterface NTPClient SDFileSystem mbed-rtos mbed wave_player PinDetect
Fork of WavePlayer_HelloWorld by
main.cpp
00001 //WavePlayer_HelloWorld4180 00002 //internet_clock 00003 00004 #include "mbed.h" 00005 #include "SDFileSystem.h" 00006 #include "wave_player.h" 00007 #include "EthernetInterface.h" 00008 #include "NTPClient.h" 00009 #include "uLCD_4DGL.h" 00010 #include "rtos.h" 00011 #include "PinDetect.h" 00012 00013 //pinouts 00014 SDFileSystem sd(p11, p12, p13, p14, "sd"); //SD card 00015 AnalogOut DACout(p18); 00016 wave_player waver(&DACout); 00017 PinDetect snooze(p19); //snooze button 00018 PinDetect off(p20); //turn alarm off 00019 DigitalOut myled1(LED1); 00020 DigitalOut myled2(LED2); 00021 DigitalOut myled3(LED3); 00022 00023 // Parameters 00024 char* domain_name = "0.uk.pool.ntp.org"; 00025 int port_number = 123; 00026 00027 // Networking 00028 EthernetInterface eth; 00029 NTPClient ntp_client; 00030 00031 // Graphic LCD - TX, RX, and RES pins 00032 uLCD_4DGL uLCD(p28,p27,p29); 00033 00034 #define snoozeTime 10 00035 00036 //global variables 00037 time_t ct_time; 00038 // Base alarm time-24 hour clock 00039 int baseAlarmHour = 0; //0-23 00040 int baseAlarmMin = 0; 00041 // Current alarm time 00042 int curAlarmHour = 18; //0-23 00043 int curAlarmMin = 9; 00044 00045 //time thread 00046 void time_thread(void const *args) 00047 { 00048 char time_buffer[80]; 00049 // Loop and update clock 00050 while (1) { 00051 uLCD.locate(0, 1); 00052 ct_time = time(NULL); 00053 strftime(time_buffer, 80, " %a %b %d\n %T %p %z\n %Z\n", \ 00054 localtime(&ct_time)); 00055 uLCD.printf(" UTC/GMT:\n%s", time_buffer); 00056 Thread::wait(100); 00057 } 00058 } 00059 00060 //pushbutton (p19) 00061 void snooze_hit_callback (void) 00062 { 00063 myled1 = !myled1; 00064 time_t newtime; 00065 struct tm * timeinfo; 00066 newtime = ct_time + snoozeTime; 00067 //time (&newtime); 00068 timeinfo = localtime (&newtime); 00069 curAlarmMin = timeinfo->tm_min; 00070 curAlarmHour = timeinfo->tm_hour; 00071 } 00072 00073 void off_hit_callback (void) 00074 { 00075 myled2 = !myled2; 00076 } 00077 00078 void play_file() 00079 { 00080 FILE *wave_file; 00081 printf("\n\n\nHello, wave world!\n"); 00082 wave_file=fopen("/sd/bob.wav","r"); 00083 waver.play(wave_file); 00084 fclose(wave_file); 00085 } 00086 00087 void timeCompare() 00088 { 00089 struct tm * timeinfo; 00090 timeinfo = localtime (&ct_time); 00091 if (timeinfo->tm_min == curAlarmMin && timeinfo->tm_hour == curAlarmHour) { 00092 //playing = true; 00093 play_file(); 00094 myled3 = true; 00095 } 00096 } 00097 00098 int main() 00099 { 00100 snooze.mode(PullUp); 00101 off.mode(PullUp); 00102 wait(0.01); 00103 snooze.attach_deasserted(&snooze_hit_callback); 00104 off.attach_deasserted(&off_hit_callback); 00105 snooze.setSampleFrequency(); 00106 off.setSampleFrequency(); 00107 00108 00109 00110 // Initialize LCD 00111 uLCD.baudrate(115200); 00112 uLCD.background_color(BLACK); 00113 uLCD.cls(); 00114 00115 // Connect to network and wait for DHCP 00116 uLCD.locate(0,0); 00117 uLCD.printf("Getting IP Address\n"); 00118 eth.init(); 00119 if ( eth.connect(60000) == -1 ) { 00120 uLCD.printf("ERROR: Could not\nget IP address"); 00121 return -1; 00122 } 00123 uLCD.printf("IP address is \n%s\n\n",eth.getIPAddress()); 00124 Thread::wait(1000); 00125 00126 // Read time from server 00127 uLCD.printf("Reading time...\n\r"); 00128 ntp_client.setTime(domain_name, port_number); 00129 uLCD.printf("Time set\n"); 00130 Thread::wait(2000); 00131 eth.disconnect(); 00132 00133 // Reset LCD 00134 uLCD.background_color(WHITE); 00135 uLCD.textbackground_color(WHITE); 00136 uLCD.color(RED); 00137 uLCD.cls(); 00138 uLCD.text_height(2); 00139 00140 Thread thread_time(time_thread); 00141 while(!myled3) { 00142 timeCompare(); 00143 Thread::wait(100); 00144 } 00145 00146 }
Generated on Tue Jul 12 2022 23:56:07 by
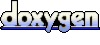