
NanoService Example for u-blox Cellular modems
Dependencies: Beep LM75B MMA7660 mbed nsdl_lib
Fork of NSDL_HelloWorld by
main.cpp
00001 #include "mbed.h" 00002 #include "UbloxUSBGSMModem.h" 00003 #include "UbloxUSBCDMAModem.h" 00004 #include "CellularModem.h" 00005 #include "Socket.h" 00006 #include "Endpoint.h" 00007 #include "UDPSocket.h" 00008 #include "C12832_lcd.h" 00009 #include "nsdl_support.h" 00010 // Include various resources 00011 #include "temperature.h" 00012 #include "light.h" 00013 #include "gps.h" 00014 #include "relay.h" 00015 00016 static C12832_LCD lcd; 00017 00018 // **************************************************************************** 00019 // Handlers for debugging crashes 00020 00021 #include <rt_misc.h> 00022 extern char Image$$RW_IRAM1$$ZI$$Limit[]; 00023 00024 extern "C" void HardFault_Handler(void) 00025 { 00026 uint32_t zi_limit = (uint32_t)Image$$RW_IRAM1$$ZI$$Limit; 00027 uint32_t sp_limit = __current_sp(); 00028 00029 zi_limit = (zi_limit + 7) & ~0x7; // ensure zi_limit is 8-byte aligned 00030 00031 struct __initial_stackheap r; 00032 r.heap_base = zi_limit; 00033 r.heap_limit = sp_limit; 00034 r=r; 00035 00036 mbed_die(); 00037 while(1); 00038 } 00039 00040 extern "C" void MemManage_Handler(void) 00041 { 00042 mbed_die(); 00043 while(1); 00044 } 00045 00046 extern "C" void BusFault_Handler(void) 00047 { 00048 mbed_die(); 00049 while(1); 00050 } 00051 00052 extern "C" void UsageFault_Handler(void) 00053 { 00054 mbed_die(); 00055 while(1); 00056 } 00057 00058 // **************************************************************************** 00059 // Configuration section 00060 00061 #define MODEM_UBLOX_CDMA 00062 //#define MODEM_UBLOX_GSM 00063 00064 #if !defined(MODEM_UBLOX_GSM) && !defined(MODEM_UBLOX_CDMA) 00065 #warning No modem defined, using GSM by default 00066 #define MODEM_UBLOX_GSM 00067 #endif 00068 00069 #ifndef MODEM_APN 00070 #warning APN not specified, using "internet" 00071 #define MODEM_APN "internet" 00072 #endif 00073 00074 #ifndef MODEM_USERNAME 00075 #warning username not specified 00076 #define MODEM_USERNAME NULL 00077 #endif 00078 00079 #ifndef MODEM_PASSWORD 00080 #warning password not specified 00081 #define MODEM_PASSWORD NULL 00082 #endif 00083 00084 // Ethernet configuration 00085 /* Define this to enable DHCP, otherwise manual address configuration is used */ 00086 #define DHCP 00087 00088 /* Manual IP configurations, if DHCP not defined */ 00089 #define IP "10.45.0.206" 00090 #define MASK "255.255.255.0" 00091 #define GW "10.45.0.1" 00092 00093 // NSP configuration 00094 /* Change this IP address to that of your NanoService Platform installation */ 00095 static const char* NSP_ADDRESS = "217.140.101.20"; /* demo NSP, web interface at http://nanoservice-demo.mbed.org*/ 00096 static const int NSP_PORT = 5683; 00097 char endpoint_name[16] = "mbed-"; 00098 uint8_t ep_type[] = {"mbed_device"}; 00099 uint8_t lifetime_ptr[] = {"1200"}; 00100 00101 UDPSocket server; 00102 Endpoint nsp; 00103 00104 // **************************************************************************** 00105 // u-blox Cellular initialization 00106 // needed for offline debugging to eliminate the semi-hosting calls 00107 //extern "C" int mbed_interface_uid(char *uid) 00108 //{ 00109 // uid[27] = '1'; 00110 // uid[28] = 'a'; 00111 // uid[29] = '5'; 00112 // uid[30] = 'b'; 00113 // uid[31] = '3'; 00114 // uid[32] = '\0'; 00115 // 00116 // return 1; 00117 //} 00118 00119 00120 static void cellular_init(CellularModem& modem, const char* apn = NULL, const char* username = NULL, const char* password= NULL) 00121 { 00122 char mbed_uid[33]; // for creating unique name for the board 00123 00124 modem.power(true); 00125 Thread::wait(1000); 00126 int ret = modem.connect(MODEM_APN, MODEM_USERNAME, MODEM_PASSWORD); 00127 if(ret) 00128 { 00129 printf("Could not connect\n"); 00130 } 00131 printf("Connection OK\n"); 00132 00133 mbed_interface_uid(mbed_uid); 00134 mbed_uid[32] = '\0'; 00135 strncat(endpoint_name, mbed_uid + 27, 15 - strlen(endpoint_name)); 00136 } 00137 00138 00139 // **************************************************************************** 00140 // NSP initialization 00141 00142 static void nsp_init() 00143 { 00144 server.init(); 00145 server.bind(NSP_PORT); 00146 00147 nsp.set_address(NSP_ADDRESS, NSP_PORT); 00148 00149 printf("name: %s", endpoint_name); 00150 printf("NSP=%s - port %d\n", NSP_ADDRESS, NSP_PORT); 00151 00152 lcd.locate(0,22); 00153 lcd.printf("EP name:%s\n", endpoint_name); 00154 } 00155 00156 // **************************************************************************** 00157 // Resource creation 00158 00159 static int create_resources() 00160 { 00161 sn_nsdl_resource_info_s *resource_ptr = NULL; 00162 sn_nsdl_ep_parameters_s *endpoint_ptr = NULL; 00163 00164 printf("Creating resources"); 00165 00166 /* Create resources */ 00167 resource_ptr = (sn_nsdl_resource_info_s*)nsdl_alloc(sizeof(sn_nsdl_resource_info_s)); 00168 if(!resource_ptr) 00169 return 0; 00170 memset(resource_ptr, 0, sizeof(sn_nsdl_resource_info_s)); 00171 00172 resource_ptr->resource_parameters_ptr = (sn_nsdl_resource_parameters_s*)nsdl_alloc(sizeof(sn_nsdl_resource_parameters_s)); 00173 if(!resource_ptr->resource_parameters_ptr) 00174 { 00175 nsdl_free(resource_ptr); 00176 return 0; 00177 } 00178 memset(resource_ptr->resource_parameters_ptr, 0, sizeof(sn_nsdl_resource_parameters_s)); 00179 00180 // Static resources 00181 nsdl_create_static_resource(resource_ptr, sizeof("dev/mfg")-1, (uint8_t*) "dev/mfg", 0, 0, (uint8_t*) "Sensinode", sizeof("Sensinode")-1); 00182 nsdl_create_static_resource(resource_ptr, sizeof("dev/mdl")-1, (uint8_t*) "dev/mdl", 0, 0, (uint8_t*) "NSDL-C mbed device", sizeof("NSDL-C mbed device")-1); 00183 00184 // Dynamic resources 00185 create_temperature_resource(resource_ptr); 00186 create_light_resource(resource_ptr); 00187 create_gps_resource(resource_ptr); 00188 create_relay_resource(resource_ptr); 00189 00190 /* Register with NSP */ 00191 endpoint_ptr = nsdl_init_register_endpoint(endpoint_ptr, (uint8_t*)endpoint_name, ep_type, lifetime_ptr); 00192 if(sn_nsdl_register_endpoint(endpoint_ptr) != 0) 00193 printf("NSP registering failed\r\n"); 00194 else 00195 printf("NSP registering OK\r\n"); 00196 nsdl_clean_register_endpoint(&endpoint_ptr); 00197 00198 nsdl_free(resource_ptr->resource_parameters_ptr); 00199 nsdl_free(resource_ptr); 00200 return 1; 00201 } 00202 00203 // running led 00204 Ticker flash; 00205 DigitalOut led(LED1); 00206 void flashLED(void){led = !led;} 00207 00208 // **************************************************************************** 00209 // Program entry point 00210 00211 int main() 00212 { 00213 flash.attach(&flashLED, 1.0f); 00214 00215 lcd.cls(); 00216 lcd.locate(0,0); 00217 lcd.printf("mbed NanoService u-blox"); 00218 printf("mbed NanoService u-blox Example App 0.1\n"); 00219 00220 #ifdef MODEM_UBLOX_GSM 00221 UbloxUSBGSMModem modem; 00222 #else 00223 UbloxUSBCDMAModem modem(p18, true, 1); 00224 #endif 00225 00226 // Initialize Cellular interface first 00227 puts("cellular_init"); 00228 cellular_init(modem); 00229 00230 // Initialize NSP node 00231 puts("nsp_init"); 00232 nsp_init(); 00233 00234 // Initialize NSDL stack 00235 puts("nsdl_init"); 00236 nsdl_init(); 00237 00238 // Create NSDL resources 00239 puts("create_resources"); 00240 create_resources(); 00241 00242 // Run the NSDL event loop (never returns) 00243 puts("nsdl_event_loop"); 00244 nsdl_event_loop(); 00245 }
Generated on Thu Jul 14 2022 08:47:03 by
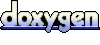