Dyanamixel
Dependents: YOZAKURA_ARM YOZAKURA_ARM_USB YOZAKURA_ARM_USB_Keyboard YOZAKURA_ARM_Keyboard0424 ... more
MX28.h
00001 /* mbed AX-12+ Servo Library 00002 * 00003 * Copyright (c) 2010, cstyles (http://mbed.org) 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #ifndef MBED_MX28_H 00025 #define MBED_MX28_H 00026 00027 #include "mbed.h" 00028 #include "SerialHalfDuplex.h" 00029 00030 #define MX28_WRITE_DEBUG 0 00031 #define MX28_READ_DEBUG 0 00032 #define MX28_TRIGGER_DEBUG 0 00033 #define MX28_DEBUG 0 00034 #define MX28_ERROR_DEBUG 0 00035 00036 #define MX28_REG_ID 0x3 00037 #define MX28_REG_CW_LIMIT 0x06 00038 #define MX28_REG_CCW_LIMIT 0x08 00039 #define MX28_REG_TORQUE_ENABLE 0x18 00040 #define MX28_REG_GOAL_POSITION 0x1E 00041 #define MX28_REG_MOVING_SPEED 0x20 00042 #define MX28_REG_VOLTS 0x2A 00043 #define MX28_REG_TEMP 0x2B 00044 #define MX28_REG_MOVING 0x2E 00045 #define MX28_REG_POSITION 0x24 00046 #define MX28_REG_TORQUE_LIMIT 0x22 00047 #define MX28_REG_CURRENT 0x38 00048 00049 #define MX28_MODE_POSITION 0 00050 #define MX28_MODE_ROTATION 1 00051 00052 #define MX28_CW 1 00053 #define MX28_CCW 0 00054 00055 class MX28 00056 { 00057 00058 public: 00059 00060 /** Create an MX28 servo object connected to the specified serial port, with the specified ID 00061 * 00062 * @param pin tx pin 00063 * @param pin rx pin 00064 * @param int ID, the Bus ID of the servo 1-255 00065 */ 00066 MX28(PinName tx, PinName rx, int ID, int baudrate); 00067 00068 /** Set the mode of the servo 00069 * @param mode 00070 * 0 = Positional, default 00071 * 1 = Continuous rotation 00072 */ 00073 int SetMode(int mode); 00074 00075 /** Set goal angle in integer degrees, in positional mode 00076 * 00077 * @param degrees 0-360 00078 * @param flags, defaults to 0 00079 * flags[0] = blocking, return when goal position reached 00080 * flags[1] = register, activate with a broadcast trigger 00081 * 00082 */ 00083 int SetGoal(int degrees, int flags = 0); 00084 00085 /** Set the speed of the servo in continuous rotation mode 00086 * 00087 * @param speed, -1.0 to 1.0 00088 * -1.0 = full speed counter clock wise 00089 * 1.0 = full speed clock wise 00090 */ 00091 int SetCRSpeed(float speed); 00092 00093 00094 /** Set the clockwise limit of the servo 00095 * 00096 * @param degrees, 0-360 00097 */ 00098 int SetCWLimit(int degrees); 00099 00100 /** Set the counter-clockwise limit of the servo 00101 * 00102 * @param degrees, 0-360 00103 */ 00104 int SetCCWLimit(int degrees); 00105 00106 // Change the ID 00107 00108 /** Change the ID of a servo 00109 * 00110 * @param CurentID 1-255 00111 * @param NewID 1-255 00112 * 00113 * If a servo ID is not know, the broadcast address of 0 can be used for CurrentID. 00114 * In this situation, only one servo should be connected to the bus 00115 */ 00116 int SetID(int CurrentID, int NewID); 00117 00118 00119 /** Poll to see if the servo is moving 00120 * 00121 * @returns true is the servo is moving 00122 */ 00123 int isMoving(void); 00124 00125 /** Send the broadcast "trigger" command, to activate any outstanding registered commands 00126 */ 00127 void trigger(void); 00128 00129 /** Read the current angle of the servo 00130 * 00131 * @returns float in the range 0.0-360.0 00132 */ 00133 float GetPosition(); 00134 00135 /** Read the temperature of the servo 00136 * 00137 * @returns float temperature 00138 */ 00139 float GetTemp(void); 00140 00141 /** Read the supply voltage of the servo 00142 * 00143 * @returns float voltage 00144 */ 00145 float GetVolts(void); 00146 00147 /** Read the supply current of the servo 00148 * 00149 * @returns float current 00150 */ 00151 float GetCurrent(void); 00152 00153 int TorqueEnable(int mode); 00154 00155 int SetTorqueLimit(float torque_limit); 00156 00157 private : 00158 00159 SerialHalfDuplex _mx28; 00160 // Serial _mx28; 00161 int _ID; 00162 00163 int read(int ID, int start, int length, char* data); 00164 int write(int ID, int start, int length, char* data, int flag=0); 00165 00166 }; 00167 00168 #endif
Generated on Tue Jul 19 2022 09:17:42 by
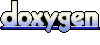