
Weather casting with Machine Learning (SVM and SRNN).
Dependencies: EthernetInterface GraphicHandler NTPClient SRNN SVM SensorModule mbed-rtos mbed
setup.cpp
00001 #include "setup.hpp" 00002 00003 // mcsvmのセットアップ : サンプル/係数のセット 00004 static void mcsvm_setup(void) 00005 { 00006 FILE* svm_setup_fp; 00007 char buf_str[20]; 00008 int ret, line; 00009 float buf_data[DIM_SIGNAL]; 00010 00011 float* svm_tmp_sample = new float[MCSVM_NUM_SAMPLES * DIM_SIGNAL]; 00012 int* svm_tmp_sample_label = new int[MCSVM_NUM_SAMPLES]; 00013 float* svm_tmp_mc_alpha = new float[MCSVM_NUM_SAMPLES * NUM_WEATHERS * (NUM_WEATHERS - 1) / 2]; 00014 00015 // You can use /dat/SVM_SAMP.txt 00016 svm_setup_fp = fopen( "/local/SVM_SAMP.CSV" , "r" ); 00017 if( svm_setup_fp == NULL ) { 00018 fprintf( stderr, "Error in svm setup : sample file cannot open. \r \n" ); 00019 exit(1); 00020 } 00021 00022 line = 0; 00023 while( ( ret = fscanf( svm_setup_fp, " %[^\n,],%f,%f,%f", buf_str, &(buf_data[0]), &(buf_data[1]), &(buf_data[2])) ) != EOF ) { 00024 00025 if ( !strcmp(buf_str,"shiny") ) { 00026 svm_tmp_sample_label[line] = SHINY; 00027 } else if ( !strcmp(buf_str,"cloudy") ) { 00028 svm_tmp_sample_label[line] = CLOUDY; 00029 } else if ( !strcmp(buf_str,"rainy") ) { 00030 svm_tmp_sample_label[line] = RAINY; 00031 } else if ( !strcmp(buf_str,"snowy") ) { 00032 svm_tmp_sample_label[line] = SNOWY; 00033 } else { 00034 continue; 00035 } 00036 00037 memcpy(&(svm_tmp_sample[line * DIM_SIGNAL]), buf_data, sizeof(float) * DIM_SIGNAL); 00038 // printf("svm sample loading.... ret : %d line : %d %s %f %f %f \r\n", ret, line, buf_str, svm_tmp_sample[line*3], svm_tmp_sample[line*3+1], svm_tmp_sample[line*3+2]); 00039 line++; 00040 } 00041 00042 mcsvm = new MCSVM(NUM_WEATHERS, DIM_SIGNAL, MCSVM_NUM_SAMPLES, svm_tmp_sample, svm_tmp_sample_label); 00043 00044 // Thank you freopen. 00045 // Here, we should not use fclose -> fopen 00046 00047 // You can use /dat/SVM_ALPH.txt 00048 svm_setup_fp = freopen("/local/SVM_ALPH.CSV", "r", svm_setup_fp ); 00049 fflush( svm_setup_fp ); // required. 00050 00051 if ( svm_setup_fp == NULL ) { 00052 fprintf( stderr, "Error in open learned alpha data. \r\n"); 00053 exit(1); 00054 } 00055 00056 // 一列のデータではfscanfフォーマットがだるいので, fgetsを使用 00057 line = 0; 00058 while( fgets( buf_str, 20, svm_setup_fp ) != NULL ) { 00059 svm_tmp_mc_alpha[line] = atof(buf_str); 00060 // printf("%d %f \r\n", line, tmp_mc_alpha[line]); 00061 line++; 00062 } 00063 00064 fclose( svm_setup_fp ); 00065 00066 mcsvm->set_alpha(svm_tmp_mc_alpha, MCSVM_NUM_SAMPLES, NUM_WEATHERS); 00067 00068 delete [] svm_tmp_sample; 00069 delete [] svm_tmp_sample_label; 00070 delete [] svm_tmp_mc_alpha; 00071 00072 } 00073 00074 // SRNNのセットアップ. 初期データのセット. 00075 static void srnn_setup(void) 00076 { 00077 FILE* srnn_setup_fp; 00078 int ret; 00079 float buf_data[DIM_SIGNAL]; 00080 float sample_maxmin[DIM_SIGNAL * 2]; 00081 char buf_str[20]; 00082 00083 // グローバルなサンプルキューを**ここで**アロケート 00084 srnn_sample_queue = new float[DIM_SIGNAL * LEN_DATA_SEQUENCE]; 00085 00086 // 信号の正規化のために, 信号の最大値と最小値を決めてやる必要がある. 00087 sample_maxmin[0] = 50; 00088 sample_maxmin[1] = -20; // 気温の最大/最小値(想定値) 00089 sample_maxmin[2] = 1030; 00090 sample_maxmin[3] = 960; // 気圧 00091 sample_maxmin[4] = 100; 00092 sample_maxmin[5] = 0; // 湿度 00093 00094 srnn_setup_fp = fopen( SEQUENCE_DATA_NAME, "r"); 00095 if( srnn_setup_fp == NULL ) { 00096 fprintf( stderr, "Error in SRNN setup. sample file cannot open. \r\n"); 00097 exit(1); 00098 } 00099 00100 int line = 0; 00101 while( ( ret = fscanf( srnn_setup_fp, " %[^\n,],%f,%f,%f", buf_str, &(buf_data[0]), &(buf_data[1]), &(buf_data[2])) ) != EOF ) { 00102 if ( line == LEN_DATA_SEQUENCE ) break; 00103 memcpy(&(srnn_sample_queue[line * DIM_SIGNAL]), buf_data, sizeof(float) * DIM_SIGNAL); 00104 // printf("sample %d : %f %f %f \r\n", line, MATRIX_AT(srnn_sample_queue,DIM_SIGNAL,line,0),MATRIX_AT(srnn_sample_queue,DIM_SIGNAL,line,1), MATRIX_AT(srnn_sample_queue,DIM_SIGNAL,line,2)); 00105 line++; 00106 } 00107 00108 fclose( srnn_setup_fp ); 00109 00110 /* アドバイス:RNNにおいては,ダイナミクス(中間層のニューロン数)は多いほど良い */ 00111 srnn = new SRNN(DIM_SIGNAL, 20, LEN_DATA_SEQUENCE, PREDICT_LENGTH, srnn_sample_queue, sample_maxmin); 00112 00113 } 00114 00115 // センサーのセットアップ. 00116 static void sensor_setup(void) 00117 { 00118 sensor_module = new SensorModule(5); 00119 } 00120 00121 // ネットワークのセットアップ 00122 static void network_setup(void) 00123 { 00124 // セットアップ, 最初の時間取得 00125 const char prefix_net_str[] = "[Network Status]"; 00126 00127 //setup ethernet interface 00128 printf("%s Ethernet initializing....", prefix_net_str); 00129 if ( eth_if.init() < 0 ) {// Use DHCP 00130 fprintf( stderr, "%s Ethernet init failed. \r\n", prefix_net_str); 00131 exit(1); 00132 } 00133 00134 if ( eth_if.connect() < 0 ) { 00135 // (offlineが確定する -> offline modeへ). 00136 fprintf( stderr, "%s Ethernet connect failed. Go To offline \r\n", prefix_net_str); 00137 return; 00138 } 00139 00140 // init time 00141 printf("%s Trying to update time...\r\n", prefix_net_str); 00142 00143 // Please specify near ntp server. ex) Japan -> ntp.nict.jp:123 00144 if (ntp_client.setTime("ntp.nict.jp") == 0) { 00145 printf("%s Set time successfully! \r\n", prefix_net_str); 00146 } else { 00147 fprintf( stderr, "%s Error in setup time \r\n", prefix_net_str); 00148 } 00149 00150 // setup http server 00151 // http_server = new HTTPServer(80, "/local/"); 00152 00153 printf("%s IP Address : %s \r\n", prefix_net_str, eth_if.getIPAddress()); 00154 printf("%s Network setup finished! \r\n", prefix_net_str); 00155 } 00156 00157 // グラフィックハンドラの初期化 00158 static void graphic_handler_setup(void) 00159 { 00160 graphic_handler = new GraphicHandler(DIM_SIGNAL, PREDICT_INTERVAL_TIME, PREDICT_LENGTH, time(NULL)); 00161 } 00162 00163 // データの初期化(アロケート) 00164 static void data_setup(void) 00165 { 00166 new_seqence_data = new float[DIM_SIGNAL]; // 現在の(一番新しい)系列データ 00167 new_predict_data = new float[DIM_SIGNAL * PREDICT_LENGTH]; // 現在の予測結果 00168 new_predict_weather = new int[PREDICT_LENGTH]; // 現在の予測天気 00169 new_predict_probability = new float[PREDICT_LENGTH]; // 現在の予測天気の確率 00170 } 00171 00172 // 時刻の設定 00173 static void jst_setup(int year, int month, int day, int hour, int min) 00174 { 00175 // setup time structure 00176 struct tm t; 00177 t.tm_sec = 0; // 0-59 00178 t.tm_min = min; // 0-59 00179 t.tm_hour = hour; // 0-23 00180 t.tm_mday = day; // 1-31 00181 t.tm_mon = (month-1); // 0-11 00182 t.tm_year = (year-1900); // year since 1900 00183 00184 // convert to timestamp and set 00185 time_t seconds = mktime(&t); 00186 set_time(seconds); 00187 } 00188 00189 // セットアップ. 00190 void setup(void) 00191 { 00192 printf("SETUP START "); 00193 printf("-------------------------- \r\n"); 00194 mcsvm_setup(); 00195 printf("SVM ...OK \r\n"); 00196 srnn_setup(); 00197 printf("SRNN ...OK \r\n"); 00198 //sensor_setup(); 00199 printf("SENSOR ...OK \r\n"); 00200 // network_setup(); 00201 jst_setup(2015, 2, 20, 4, 0); 00202 printf("NETWORK ...NO(offline) \r\n"); 00203 graphic_handler_setup(); 00204 printf("GRAPHIC ...OK \r\n"); 00205 data_setup(); 00206 printf("SHARED DATA ...OK \r\n"); 00207 printf("SETUP SUCCESS "); 00208 printf("-------------------------- \r\n"); 00209 }
Generated on Wed Jul 13 2022 17:43:05 by
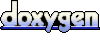