FIAPライブラリ 構造体の内容が変わっています
Dependents: temp_FIAP temp_FIAP_fetch tepco_demand BlueUSB_f_IEEE1888 ... more
fiap.cpp
00001 #include "fiap.h" 00002 #include "mbed.h" 00003 #include "stdio.h" 00004 #include "spdomparser.hpp" 00005 #include "spxmlnode.hpp" 00006 #include "spxmlhandle.hpp" 00007 00008 char outBuffer[2000+1]= {0}; 00009 char _soap_text[2000]; 00010 char uuid[37]; 00011 // HTTPClient http; 00012 // HTTPText InData("text/html", 800); 00013 // HTTPStream stream; 00014 //char str[50]; 00015 //int yy,mo,dd,hh,mm,ss; 00016 //char requestBuffer[50]; 00017 //int i,ii,ll; 00018 //HTTPResult r; 00019 //FIAP::FIAP(string Storage,string PointSetId) { 00020 00021 void FIAP::post_xml_initialize(void) 00022 { 00023 strcpy(_soap_header,"<?xml version=\"1.0\" encoding=\"UTF-8\"?>"); 00024 strcat(_soap_header,"<soapenv:Envelope xmlns:soapenv=\"http://schemas.xmlsoap.org/soap/envelope/\">"); 00025 strcat(_soap_header,"<soapenv:Body>"); 00026 strcat(_soap_header,"<ns2:dataRQ xmlns:ns2=\"http://soap.fiap.org/\">"); 00027 strcat(_soap_header,"<transport xmlns=\"http://gutp.jp/fiap/2009/11/\">"); 00028 strcat(_soap_header,"<body>"); 00029 strcpy(_soap_footer,"</body>"); 00030 strcat(_soap_footer,"</transport>"); 00031 strcat(_soap_footer,"</ns2:dataRQ>"); 00032 strcat(_soap_footer,"</soapenv:Body>"); 00033 strcat(_soap_footer,"</soapenv:Envelope>"); 00034 strcat(_soap_footer,"\r\n\r\n"); 00035 strcpy(_soap_action,"\"http://soap.fiap.org/data\""); 00036 } 00037 void FIAP::fetch_xml_initialize(void) 00038 { 00039 strcpy(_soap_header,"<?xml version=\"1.0\" encoding=\"UTF-8\"?>"); 00040 strcat(_soap_header,"<soapenv:Envelope xmlns:soapenv=\"http://schemas.xmlsoap.org/soap/envelope/\">"); 00041 strcat(_soap_header,"<soapenv:Body>"); 00042 strcat(_soap_header,"<ns2:queryRQ xmlns:ns2=\"http://soap.fiap.org/\">"); 00043 strcat(_soap_header,"<transport xmlns=\"http://gutp.jp/fiap/2009/11/\">"); 00044 strcat(_soap_header,"<header>"); 00045 strcpy(_soap_footer,"</header>"); 00046 strcat(_soap_footer,"</transport>"); 00047 strcat(_soap_footer,"</ns2:queryRQ>"); 00048 strcat(_soap_footer,"</soapenv:Body>"); 00049 strcat(_soap_footer,"</soapenv:Envelope>"); 00050 strcat(_soap_footer,"\r\n\r\n"); 00051 strcpy(_soap_action,"\"http://soap.fiap.org/query\""); 00052 } 00053 00054 FIAP::FIAP(char Storage[]) 00055 { 00056 debug_mode=false; 00057 strcpy(_fiap_storage,Storage); 00058 } 00059 00060 int FIAP::fetch_last_data(struct fiap_element* v,unsigned int esize) 00061 { 00062 HTTPClient http; 00063 int i,ii,ll,k1,k2; 00064 fetch_xml_initialize(); 00065 strcpy( _soap_text, _soap_header); 00066 sprintf(uuid,"%04x%04x-%04x-%04x-%04x-%04x%04x%04x",rand()%0x10000,rand()%0x10000,rand()%0x10000,rand()%1000|0x4000,rand()%0x1000|0x8000,rand()%0x10000,rand()%10000,(rand()+1)%0x10000); 00067 strcat( _soap_text , "<query id=\""); 00068 strcat( _soap_text , uuid); 00069 strcat( _soap_text , "\" type=\"storage\">"); 00070 for (i=0; i<esize; i++) { 00071 strcat(_soap_text , "<key id=\""); 00072 strcat(_soap_text , v[i].cid); 00073 strcat(_soap_text , "\" attrName=\"time\" select=\"maximum\"/>"); 00074 } 00075 strcat(_soap_text , "</query>"); 00076 strcat(_soap_text , _soap_footer); 00077 if (debug_mode) { 00078 printf(_fiap_storage); 00079 printf("\r\n"); 00080 printf(_soap_text); 00081 printf("<<< Request(end)\n"); 00082 } 00083 //HTTPText InData("text/html", 800); 00084 //InData.set(_soap_text); 00085 HTTPText InData(_soap_text); 00086 char rstr[800]; 00087 HTTPText stream(rstr,800); 00088 //stream.readNext((byte*)outBuffer,1500); 00089 if (debug_mode)printf("post.start \n\r"); 00090 HTTPResult r = http.postXML(_fiap_storage,"http://soap.fiap.org/query",InData,&stream); 00091 if (debug_mode) printf("post.end \n\r"); 00092 if (debug_mode) { 00093 printf("****\n\r"); 00094 printf(rstr); 00095 printf("\n\r****\n\r"); 00096 } 00097 if(!r) { 00098 if (debug_mode)printf("Success \n"); 00099 } else { 00100 00101 if (r==HTTP_PROCESSING) { 00102 if (debug_mode)printf("Processing \n"); 00103 return -1; 00104 } 00105 if (r==HTTP_PARSE) { 00106 if (debug_mode) printf("URI Parse error \n"); 00107 return -1; 00108 } 00109 if (r==HTTP_DNS) { 00110 if (debug_mode) printf("Could not resolve name\n"); 00111 return -1; 00112 } 00113 if (r==HTTP_PRTCL) { 00114 if (debug_mode)printf("Protocol error\n"); 00115 return -1; 00116 } 00117 if (r==HTTP_NOTFOUND) { 00118 if (debug_mode)printf("HTTP 404 Error\n"); 00119 return -1; 00120 } 00121 if (r==HTTP_REFUSED) { 00122 if (debug_mode) printf("HTTP 403 Error \n"); 00123 return -1; 00124 } 00125 if (r==HTTP_ERROR) { 00126 if (debug_mode)printf("HTTP xxx error %d \n ",r); 00127 return -1; 00128 } 00129 if (r==HTTP_TIMEOUT) { 00130 if (debug_mode)printf("Connection timeout\n"); 00131 return -1; 00132 } 00133 if (r==HTTP_CONN) { 00134 if (debug_mode)printf("Connection error\n"); 00135 return -1; 00136 } 00137 if (r==HTTP_CLOSED) { 00138 if (debug_mode)printf("Connection error\n"); 00139 return -1; 00140 } 00141 if (debug_mode)printf("Unknown Network Error\n"); 00142 return -1; 00143 } 00144 SP_XmlNodeList * points; 00145 SP_XmlNodeList * trans_point; 00146 if (strlen(rstr)>0) { 00147 if (debug_mode)printf("\n\r data Get Ok\n\r"); 00148 SP_XmlDomParser parser; 00149 parser.append(rstr,strlen(rstr)); 00150 //stream.readNext((byte*)outBuffer,4096); 00151 SP_XmlHandle rootHandle(parser.getDocument()->getRootElement()); 00152 SP_XmlHandle transport = rootHandle.getChild(0).getChild(0).getChild(0); 00153 SP_XmlElementNode * fiapError = transport.getChild("header").getChild("error").toElement(); 00154 int yy,mo,dd,hh,mm,ss; 00155 char str[50]; 00156 if (debug_mode) printf ("%s \n",transport.toElement()->getName()); 00157 if (fiapError==NULL) { 00158 SP_XmlElementNode * trans_element = transport.toElement(); 00159 trans_point=(SP_XmlNodeList *)trans_element->getChildren() ; 00160 k1=trans_point->getLength(); 00161 if(debug_mode)printf("Trans Child has Element of No %d \n\r",k1); 00162 SP_XmlElementNode * values; 00163 for(k2=0; k2<k1; k2++) { 00164 values =transport.getChild(k2).toElement(); 00165 if(debug_mode)printf(values->getName()); 00166 if(debug_mode)printf("\n\r"); 00167 if(strstr((values->getName()),"body") !=NULL) { 00168 if(debug_mode)printf("find body \n\r"); 00169 points=(SP_XmlNodeList *)values->getChildren() ; 00170 int j=points->getLength(); 00171 if (debug_mode) printf("GetValues of No %d \n\r",j); 00172 SP_XmlElementNode *data_point_node,*data_value_node; 00173 SP_XmlCDataNode *data_value_cnode; 00174 for (i=0; i<j; i++) { 00175 SP_XmlHandle data_handle(points->get(i));//Point 00176 data_point_node=data_handle.toElement(); 00177 //data_value_node=data_handle.getChild("value").toElement(); 00178 //data_value_cnode=data_handle.getChild("value").getChild(0).toCData(); 00179 data_value_node=data_handle.getChild(0).toElement(); 00180 data_value_cnode=data_handle.getChild(0).getChild(0).toCData(); 00181 if (debug_mode) printf("PointID=%s \r\n",data_point_node->getAttrValue("id")); 00182 if (debug_mode) printf("date=%s \r\n",data_value_node->getAttrValue("time")); 00183 if (debug_mode) printf("data=%s \r\n",data_value_cnode->getText()); 00184 for (ii=0; ii<esize; ii++) { 00185 if (strcmp(v[ii].cid,data_point_node->getAttrValue("id"))==0) { 00186 sprintf(str,"%s",data_value_node->getAttrValue("time")); 00187 ll=sscanf(str,"%d-%d-%dT%d:%d:%d.",&yy,&mo,&dd,&hh,&mm,&ss); 00188 if (debug_mode)printf("date convert no %d (%d/%d/%d %d:%d:%d) \r\n",ll,yy,mo,dd,hh,mm,ss); 00189 v[ii].year=yy; 00190 v[ii].month=mo; 00191 v[ii].day=dd; 00192 v[ii].hour=hh; 00193 v[ii].minute=mm; 00194 v[ii].second=ss; 00195 sprintf(v[ii].value,"%s",data_value_cnode->getText()); 00196 } 00197 } 00198 } 00199 00200 } 00201 00202 00203 } 00204 00205 } else { 00206 printf("ERROR\n\r"); 00207 } 00208 } else { 00209 if (debug_mode)printf("error\n\r"); 00210 } 00211 return 0; 00212 } 00213 00214 int FIAP::fetch_last_data(struct fiap_element *v) 00215 { 00216 HTTPClient http; 00217 int ll; 00218 char rstr[800]; 00219 fetch_xml_initialize(); 00220 strcpy(_soap_text, _soap_header); 00221 sprintf(uuid,"%04x%04x-%04x-%04x-%04x-%04x%04x%04x",rand()%0x10000,rand()%0x10000,rand()%0x10000,rand()%1000|0x4000,rand()%0x1000|0x8000,rand()%0x10000,rand()%10000,(rand()+1)%0x10000); 00222 strcat(_soap_text , "<query id=\""); 00223 strcat(_soap_text , uuid); 00224 strcat(_soap_text , "\" type=\"storage\">"); 00225 strcat(_soap_text , "<key id=\""); 00226 strcat(_soap_text , v->cid); 00227 strcat(_soap_text , "\" attrName=\"time\" select=\"maximum\"/>"); 00228 strcat(_soap_text , "</query>"); 00229 strcat(_soap_text , _soap_footer); 00230 if (debug_mode) { 00231 printf("\r\n"); 00232 printf(_fiap_storage); 00233 printf("\r\n"); 00234 printf(_soap_text); 00235 printf("<<< Request(end)\n"); 00236 } 00237 // http.setRequestHeader("Content-Type","text/xml; charset=UTF-8"); 00238 // http.setRequestHeader("SOAPAction","\"http://soap.fiap.org/query\""); 00239 HTTPText InData(_soap_text); 00240 HTTPText stream(rstr,800); 00241 // stream.readNext((byte*)outBuffer,strlen(outBuffer)); 00242 if (debug_mode)printf("post.start \n\r"); 00243 HTTPResult r = http.postXML(_fiap_storage,"http://soap.fiap.org/query",InData,&stream); 00244 if (debug_mode)printf("post.end \n\r"); 00245 if(!r) { 00246 if (debug_mode)printf("Success \n"); 00247 } else { 00248 if (r==HTTP_PROCESSING) { 00249 if (debug_mode)printf("Processing \n"); 00250 return -1; 00251 } 00252 if (r==HTTP_PARSE) { 00253 if (debug_mode) printf("URI Parse error \n"); 00254 return -1; 00255 } 00256 if (r==HTTP_DNS) { 00257 if (debug_mode) printf("Could not resolve name\n"); 00258 return -1; 00259 } 00260 if (r==HTTP_PRTCL) { 00261 if (debug_mode)printf("Protocol error\n"); 00262 return -1; 00263 } 00264 if (r==HTTP_NOTFOUND) { 00265 if (debug_mode)printf("HTTP 404 Error\n"); 00266 return -1; 00267 } 00268 if (r==HTTP_REFUSED) { 00269 if (debug_mode) printf("HTTP 403 Error \n"); 00270 return -1; 00271 } 00272 if (r==HTTP_ERROR) { 00273 if (debug_mode)printf("HTTP xxx error %d \n ",r); 00274 return -1; 00275 } 00276 if (r==HTTP_TIMEOUT) { 00277 if (debug_mode)printf("Connection timeout\n"); 00278 return -1; 00279 } 00280 if (r==HTTP_CONN) { 00281 if (debug_mode)printf("Connection error\n"); 00282 return -1; 00283 } 00284 if (r==HTTP_CLOSED) { 00285 if (debug_mode)printf("Connection error\n"); 00286 return -1; 00287 } 00288 if (debug_mode)printf("Unknown Network Error\n"); 00289 return -1; 00290 } 00291 SP_XmlNodeList * points; 00292 if (strlen(rstr)>0) { 00293 if (debug_mode)printf("\n\r data Get Ok\n\r"); 00294 00295 SP_XmlDomParser parser; 00296 00297 // if (debug_mode)printf("stream readlen = %d \n\r",stream.readLen()); 00298 // outBuffer[stream.readLen()]=0; 00299 if (debug_mode)printf("check0\n\r"); 00300 parser.append(rstr,strlen(rstr)); 00301 if (debug_mode)printf("check1\n\r"); 00302 SP_XmlHandle rootHandle(parser.getDocument()->getRootElement()); 00303 SP_XmlHandle transport = rootHandle.getChild(0).getChild(0).getChild(0); 00304 SP_XmlElementNode * fiapError = transport.getChild("header").getChild("error").toElement(); 00305 int yy,mo,dd,hh,mm,ss; 00306 char str[50]; 00307 if (debug_mode)printf ("%s \n\r",transport.toElement()->getName()); 00308 if (fiapError==NULL) { 00309 SP_XmlElementNode * values =transport.getChild("body").toElement(); 00310 points=(SP_XmlNodeList *)values->getChildren() ; 00311 // points=values->getChildren(); 00312 int j=points->getLength(); 00313 if (debug_mode) printf("GetValues of No %d \n\r",j); 00314 SP_XmlElementNode *data_point_node,*data_value_node; 00315 SP_XmlCDataNode *data_value_cnode; 00316 SP_XmlHandle data_handle(points->get(0));//Point 00317 data_point_node=data_handle.toElement(); 00318 data_value_node=data_handle.getChild("value").toElement(); 00319 data_value_cnode=data_handle.getChild("value").getChild(0).toCData(); 00320 if (debug_mode) printf("PointID=%s \r\n",data_point_node->getAttrValue("id")); 00321 if (debug_mode) printf("date=%s \r\n",data_value_node->getAttrValue("time")); 00322 if (debug_mode) printf("data=%s \r\n",data_value_cnode->getText()); 00323 sprintf(str,"%s",data_value_node->getAttrValue("time")); 00324 ll=sscanf(str,"%d-%d-%dT%d:%d:%d.",&yy,&mo,&dd,&hh,&mm,&ss); 00325 if (debug_mode)printf("date convert no %d (%d/%d/%d %d:%d:%d) \r\n",ll,yy,mo,dd,hh,mm,ss); 00326 v->year=yy; 00327 v->month=mo; 00328 v->day=dd; 00329 v->hour=hh; 00330 v->minute=mm; 00331 v->second=ss; 00332 sprintf(v->value,"%s", data_value_cnode->getText()); 00333 } else { 00334 printf("ERROR\n\r"); 00335 } 00336 } else { 00337 if (debug_mode)printf("error\n\r"); 00338 } 00339 return 0; 00340 } 00341 00342 00343 00344 int FIAP::post(struct fiap_element* v, unsigned int esize) 00345 { 00346 HTTPClient http; 00347 int i; 00348 char rstr[1200]; 00349 char requestBuffer[50]; 00350 post_xml_initialize(); 00351 strcpy(_soap_text,_soap_header); 00352 for (i=0; i<esize; i++) { 00353 sprintf(requestBuffer,"%04d-%02d-%02dT%02d:%02d:%02d.0000000",v[i].year,v[i].month,v[i].day,v[i].hour,v[i].minute,v[i].second); 00354 strcat(_soap_text , "<point id=\""); 00355 strcat(_soap_text , _fiap_id_prefix); 00356 strcat(_soap_text , v[i].cid); 00357 strcat( _soap_text, "\">"); 00358 strcat(_soap_text , "<value time=\""); 00359 strcat(_soap_text , requestBuffer); 00360 strcat(_soap_text , v[i].timezone); 00361 strcat(_soap_text , "\">"); 00362 strcat(_soap_text , v[i].value); 00363 strcat(_soap_text , "</value>"); 00364 strcat(_soap_text , "</point>"); 00365 } 00366 strcat(_soap_text , _soap_footer); 00367 if (debug_mode) { 00368 printf(_soap_text); 00369 printf("<<< Request(end)\n"); 00370 } 00371 // http.setRequestHeader("Content-Type","text/xml; charset=UTF-8"); 00372 // http.setRequestHeader("SOAPAction","\"http://soap.fiap.org/data\""); 00373 // InData=new HTTPText(); 00374 HTTPText InData(_soap_text); 00375 HTTPText OutData(rstr,1200); 00376 HTTPResult r = http.postXML(_fiap_storage,"http://soap.fiap.org/data",InData,&OutData); 00377 if(!r) { 00378 if (debug_mode)printf("Success \n"); 00379 if (debug_mode) { 00380 printf("****\n\r"); 00381 printf(rstr); 00382 printf("\n\r****\n\r"); 00383 } 00384 } else { 00385 if (r==HTTP_PROCESSING) { 00386 if (debug_mode)printf("Processing \n"); 00387 return -1; 00388 } 00389 if (r==HTTP_PARSE) { 00390 if (debug_mode) printf("URI Parse error \n"); 00391 return -1; 00392 } 00393 if (r==HTTP_DNS) { 00394 if (debug_mode) printf("Could not resolve name\n"); 00395 return -1; 00396 } 00397 if (r==HTTP_PRTCL) { 00398 if (debug_mode)printf("Protocol error\n"); 00399 return -1; 00400 } 00401 if (r==HTTP_NOTFOUND) { 00402 if (debug_mode)printf("HTTP 404 Error\n"); 00403 return -1; 00404 } 00405 if (r==HTTP_REFUSED) { 00406 if (debug_mode) printf("HTTP 403 Error \n"); 00407 return -1; 00408 } 00409 if (r==HTTP_ERROR) { 00410 if (debug_mode)printf("HTTP xxx error \n"); 00411 return -1; 00412 } 00413 if (r==HTTP_TIMEOUT) { 00414 if (debug_mode)printf("Connection timeout\n"); 00415 return -1; 00416 } 00417 if (r==HTTP_CONN) { 00418 if (debug_mode)printf("Connection error\n"); 00419 return -1; 00420 } 00421 if (r==HTTP_CLOSED) { 00422 if (debug_mode)printf("Connection error\n"); 00423 return -1; 00424 } 00425 if (debug_mode)printf("Unknown Network Error\n"); 00426 return -1; 00427 } 00428 return 0; 00429 }
Generated on Thu Jul 14 2022 19:12:45 by
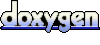