
1
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut datapin(D8); 00004 DigitalOut latchpin(D9); 00005 DigitalOut clockpin(D10); 00006 DigitalOut ww(D11); 00007 DigitalOut xx(D12); 00008 DigitalOut yy(D13); 00009 DigitalOut zz(D14); 00010 00011 const int state[10][8]= { 00012 { 0, 1, 1, 1, 1, 1, 1}, // 0 00013 { 0, 0, 0, 0, 1, 1, 0}, // 1 00014 { 1, 0, 1, 1, 0, 1, 1}, // 2 00015 { 1, 0, 0, 1, 1, 1, 1}, // 3 00016 { 1, 1, 0, 0, 1, 1, 0}, // 4 00017 { 1, 1, 0, 1, 1, 0, 1}, // 5 00018 { 1, 1, 1, 1, 1, 0, 0}, // 6 00019 { 0, 0, 0, 0, 1, 1, 1}, // 7 00020 { 1, 1, 1, 1, 1, 1, 1}, // 8 00021 { 1, 1, 0, 0, 1, 1, 1} // 9 00022 }; 00023 void seg1(int num) 00024 { 00025 ww=1; 00026 xx=1; 00027 yy=1; 00028 zz=1; 00029 latchpin=0; 00030 for(int i=0;i<=7;i++) 00031 { 00032 datapin=state[num][i]; 00033 clockpin=1; 00034 clockpin=0; 00035 } 00036 latchpin=1; 00037 ww=0; 00038 } 00039 void seg2(int num) 00040 { 00041 ww=1; 00042 xx=1; 00043 yy=1; 00044 zz=1; 00045 latchpin=0; 00046 for(int i=0;i<=7;i++) 00047 { 00048 datapin=state[num][i]; 00049 clockpin=1; 00050 clockpin=0; 00051 } 00052 latchpin=1; 00053 xx=0; 00054 } 00055 void seg3(int num) 00056 { 00057 ww=1; 00058 xx=1; 00059 yy=1; 00060 zz=1; 00061 latchpin=0; 00062 for(int i=0;i<=7;i++) 00063 { 00064 datapin=state[num][i]; 00065 clockpin=1; 00066 clockpin=0; 00067 } 00068 latchpin=1; 00069 yy=0; 00070 } 00071 void seg4(int num) 00072 { 00073 ww=1; 00074 xx=1; 00075 yy=1; 00076 zz=1; 00077 latchpin=0; 00078 for(int i=0;i<=7;i++) 00079 { 00080 datapin=state[num][i]; 00081 clockpin=1; 00082 clockpin=0; 00083 } 00084 latchpin=1; 00085 zz=0; 00086 } 00087 int main() 00088 { 00089 int round=0,round2=0,round3=0,round4=0,r=0;; 00090 while(1) 00091 { 00092 seg1(round); 00093 r++; 00094 if (r>=30) 00095 { 00096 round++; 00097 r=0; 00098 } 00099 wait_ms(5); 00100 seg2(round2); 00101 wait_ms(5); 00102 seg3(round3); 00103 wait_ms(5); 00104 seg4(round4); 00105 wait_ms(5); 00106 if(round == 10) 00107 { 00108 round=0;round2++; 00109 if(round2 == 10) 00110 { 00111 round2=0;round3++; 00112 if(round3 == 10) 00113 { 00114 round3=0; 00115 round4++; 00116 if(round4 == 10) 00117 { 00118 round4=0; 00119 } 00120 } 00121 } 00122 } 00123 } 00124 }
Generated on Mon Aug 1 2022 01:43:34 by
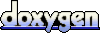