
A demo application that receive and print tempreture and humidity from STM431J by USB400J that pluged-in to mbed application board.
Dependencies: C12832 USB400Serial USBHost mbed
main.cpp
00001 #include "mbed.h" 00002 #include "C12832.h" 00003 #include "USB400Serial.h" 00004 00005 C12832 lcd(p5, p7, p6, p8, p11); 00006 00007 DigitalOut led1(LED1); 00008 DigitalOut led2(LED2); 00009 DigitalOut led3(LED3); 00010 DigitalOut led4(LED4); 00011 Serial pc(USBTX, USBRX); 00012 00013 uint8_t u8CRC8Table[256] = { 00014 0x00, 0x07, 0x0e, 0x09, 0x1c, 0x1b, 0x12, 0x15, 00015 0x38, 0x3f, 0x36, 0x31, 0x24, 0x23, 0x2a, 0x2d, 00016 0x70, 0x77, 0x7e, 0x79, 0x6c, 0x6b, 0x62, 0x65, 00017 0x48, 0x4f, 0x46, 0x41, 0x54, 0x53, 0x5a, 0x5d, 00018 0xe0, 0xe7, 0xee, 0xe9, 0xfc, 0xfb, 0xf2, 0xf5, 00019 0xd8, 0xdf, 0xd6, 0xd1, 0xc4, 0xc3, 0xca, 0xcd, 00020 0x90, 0x97, 0x9e, 0x99, 0x8c, 0x8b, 0x82, 0x85, 00021 0xa8, 0xaf, 0xa6, 0xa1, 0xb4, 0xb3, 0xba, 0xbd, 00022 0xc7, 0xc0, 0xc9, 0xce, 0xdb, 0xdc, 0xd5, 0xd2, 00023 0xff, 0xf8, 0xf1, 0xf6, 0xe3, 0xe4, 0xed, 0xea, 00024 0xb7, 0xb0, 0xb9, 0xbe, 0xab, 0xac, 0xa5, 0xa2, 00025 0x8f, 0x88, 0x81, 0x86, 0x93, 0x94, 0x9d, 0x9a, 00026 0x27, 0x20, 0x29, 0x2e, 0x3b, 0x3c, 0x35, 0x32, 00027 0x1f, 0x18, 0x11, 0x16, 0x03, 0x04, 0x0d, 0x0a, 00028 0x57, 0x50, 0x59, 0x5e, 0x4b, 0x4c, 0x45, 0x42, 00029 0x6f, 0x68, 0x61, 0x66, 0x73, 0x74, 0x7d, 0x7a, 00030 0x89, 0x8e, 0x87, 0x80, 0x95, 0x92, 0x9b, 0x9c, 00031 0xb1, 0xb6, 0xbf, 0xb8, 0xad, 0xaa, 0xa3, 0xa4, 00032 0xf9, 0xfe, 0xf7, 0xf0, 0xe5, 0xe2, 0xeb, 0xec, 00033 0xc1, 0xc6, 0xcf, 0xc8, 0xdd, 0xda, 0xd3, 0xd4, 00034 0x69, 0x6e, 0x67, 0x60, 0x75, 0x72, 0x7b, 0x7c, 00035 0x51, 0x56, 0x5f, 0x58, 0x4d, 0x4a, 0x43, 0x44, 00036 0x19, 0x1e, 0x17, 0x10, 0x05, 0x02, 0x0b, 0x0c, 00037 0x21, 0x26, 0x2f, 0x28, 0x3d, 0x3a, 0x33, 0x34, 00038 0x4e, 0x49, 0x40, 0x47, 0x52, 0x55, 0x5c, 0x5b, 00039 0x76, 0x71, 0x78, 0x7f, 0x6A, 0x6d, 0x64, 0x63, 00040 0x3e, 0x39, 0x30, 0x37, 0x22, 0x25, 0x2c, 0x2b, 00041 0x06, 0x01, 0x08, 0x0f, 0x1a, 0x1d, 0x14, 0x13, 00042 0xae, 0xa9, 0xa0, 0xa7, 0xb2, 0xb5, 0xbc, 0xbb, 00043 0x96, 0x91, 0x98, 0x9f, 0x8a, 0x8D, 0x84, 0x83, 00044 0xde, 0xd9, 0xd0, 0xd7, 0xc2, 0xc5, 0xcc, 0xcb, 00045 0xe6, 0xe1, 0xe8, 0xef, 0xfa, 0xfd, 0xf4, 0xf3 00046 }; 00047 00048 #define proccrc8(u8CRC, u8Data) (u8CRC8Table[u8CRC ^ u8Data]) 00049 00050 void serial_task(void const*) 00051 { 00052 USB400Serial serial; 00053 int i; 00054 int dataLength; 00055 int optionalLength; 00056 uint8_t c; 00057 uint8_t crc; 00058 int sensor = 0; 00059 int humidity = 0; 00060 int temperature = 0; 00061 00062 while(1) { 00063 00064 while(!serial.connect()) 00065 Thread::wait(500); 00066 00067 while (1) { 00068 00069 if (!serial.connected()) 00070 break; 00071 00072 if (serial.available()) { 00073 c = serial.getc(); 00074 00075 if (c == 0x55) { 00076 crc = 0; 00077 00078 c = serial.getc(); 00079 crc = proccrc8(crc, c); 00080 dataLength = c; 00081 00082 c = serial.getc(); 00083 crc = proccrc8(crc, c); 00084 dataLength = (dataLength << 8) | c; 00085 00086 c = serial.getc(); 00087 crc = proccrc8(crc, c); 00088 optionalLength = c; 00089 00090 c = serial.getc(); 00091 crc = proccrc8(crc, c); // packet type 00092 00093 c = serial.getc(); 00094 crc = proccrc8(crc, c); // CRC 00095 00096 if (crc == 0) { 00097 crc = 0; 00098 00099 for (i = 0; i < dataLength; i++) { 00100 c = serial.getc(); 00101 crc = proccrc8(crc, c); 00102 00103 if (i == 4) { 00104 // printf("Sensor: %02X ", c); 00105 sensor = c; 00106 } 00107 //A5-04-01 00108 if (i == 6) { 00109 humidity = c; 00110 } 00111 if (i == 7) { 00112 temperature = c; 00113 } 00114 } 00115 00116 // printf("hum:%3.1f%% temp:%2.2fC\r\n", humidity*0.4, temperature*0.16); 00117 00118 if (sensor == 0x34) { 00119 lcd.locate(0,12); 00120 lcd.printf("%02X Temp:%2.2f C Hum:%3.1f %%\r\n", sensor, temperature*0.16, humidity*0.4); 00121 } 00122 00123 if (sensor == 0x52) { 00124 lcd.locate(0,21); 00125 lcd.printf("%02X Temp:%2.2f C Hum:%3.1f %%\r\n", sensor, temperature*0.16, humidity*0.4); 00126 } 00127 00128 for (i = 0; i < optionalLength; i++) { 00129 c = serial.getc(); 00130 crc = proccrc8(crc, c); 00131 } 00132 00133 c = serial.getc(); 00134 crc = proccrc8(crc, c); 00135 00136 } 00137 } 00138 } 00139 } 00140 } 00141 } 00142 00143 int main() 00144 { 00145 lcd.cls(); 00146 lcd.locate(0,3); 00147 lcd.printf("mbed application board!"); 00148 00149 Thread serialTask(serial_task, NULL, osPriorityNormal, 256 * 4); 00150 while(1) { 00151 led1 = !led1; 00152 Thread::wait(500); 00153 } 00154 }
Generated on Wed Jul 13 2022 00:58:06 by
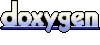