
RTnoV3_LED is example program which uses mbed. To know about RTno, visit: http://ysuga.net/robot_e/rtm_e/rtc_e/1065?lang=en
Dependencies: EthernetNetIf mbed RTnoV3
main.cpp
00001 #include "mbed.h" 00002 /** 00003 * RTno_Template.pde 00004 * RTno is RT-middleware and arduino. 00005 * 00006 * Using RTno, arduino device can communicate any RT-components 00007 * through the RTno-proxy component which is launched in PC. 00008 * Connect arduino with USB, and program with RTno library. 00009 * You do not have to define any protocols to establish communication 00010 * between arduino and PC. 00011 * 00012 * Using RTno, you must not define the function "setup" and "loop". 00013 * Those functions are automatically defined in the RTno libarary. 00014 * You, developers, must define following functions: 00015 * int onInitialize(void); 00016 * int onActivated(void); 00017 * int onDeactivated(void); 00018 * int onExecute(void); 00019 * int onError(void); 00020 * int onReset(void); 00021 * These functions are spontaneously called by the RTno-proxy 00022 * RT-component which is launched in the PC. 00023 * @author Yuki Suga 00024 * This code is written/distributed for public-domain. 00025 */ 00026 00027 #include <RTno.h> 00028 00029 /** 00030 * This function is called at first. 00031 * conf._default.baudrate: baudrate of serial communication 00032 * exec_cxt.periodic.type: reserved but not used. 00033 */ 00034 void rtcconf(config_str& conf, exec_cxt_str& exec_cxt) { 00035 // If you want to use Serial Connection, configure below: 00036 conf._default.connection_type = ConnectionTypeSerialUSB; // USBTX & USBRX (In Windows, Driver must be updated.) 00037 conf._default.baudrate = 57600; // This value is required when you select ConnectionTypeSerial* 00038 exec_cxt.periodic.type = ProxySynchronousExecutionContext; 00039 } 00040 00041 00042 /** 00043 * Declaration Division: 00044 * 00045 * DataPort and Data Buffer should be placed here. 00046 * 00047 * available data types are as follows: 00048 * TimedLong 00049 * TimedDouble 00050 * TimedFloat 00051 * TimedLongSeq 00052 * TimedDoubleSeq 00053 * TimedFloatSeq 00054 * 00055 * Please refer following comments. If you need to use some ports, 00056 * uncomment the line you want to declare. 00057 **/ 00058 TimedLong in0; 00059 InPort<TimedLong> in0In("in0", in0); 00060 00061 DigitalOut led1(LED1); 00062 DigitalOut led2(LED2); 00063 DigitalOut led3(LED3); 00064 DigitalOut led4(LED4); 00065 ////////////////////////////////////////// 00066 // on_initialize 00067 // 00068 // This function is called in the initialization 00069 // sequence. The sequence is triggered by the 00070 // PC. When the RTnoRTC is launched in the PC, 00071 // then, this function is remotely called 00072 // through the USB cable. 00073 // In on_initialize, usually DataPorts are added. 00074 // 00075 ////////////////////////////////////////// 00076 int RTno::onInitialize() { 00077 /* Data Ports are added in this section. 00078 */ 00079 00080 addInPort(in0In); 00081 00082 // Some initialization (like port direction setting) 00083 led1 = 1; 00084 led2 = 0; 00085 led3 = 0; 00086 led4 = 0; 00087 return RTC_OK; 00088 } 00089 00090 //////////////////////////////////////////// 00091 // on_activated 00092 // This function is called when the RTnoRTC 00093 // is activated. When the activation, the RTnoRTC 00094 // sends message to call this function remotely. 00095 // If this function is failed (return value 00096 // is RTC_ERROR), RTno will enter ERROR condition. 00097 //////////////////////////////////////////// 00098 int RTno::onActivated() { 00099 // Write here initialization code. 00100 led2 = 1; 00101 led3 = 0; 00102 led4 = 0; 00103 return RTC_OK; 00104 } 00105 00106 ///////////////////////////////////////////// 00107 // on_deactivated 00108 // This function is called when the RTnoRTC 00109 // is deactivated. 00110 ///////////////////////////////////////////// 00111 int RTno::onDeactivated() 00112 { 00113 // Write here finalization code. 00114 led2 = 0; 00115 led3 = 0; 00116 led4 = 0; 00117 return RTC_OK; 00118 } 00119 00120 ////////////////////////////////////////////// 00121 // This function is repeatedly called when the 00122 // RTno is in the ACTIVE condition. 00123 // If this function is failed (return value is 00124 // RTC_ERROR), RTno immediately enter into the 00125 // ERROR condition.r 00126 ////////////////////////////////////////////// 00127 int RTno::onExecute() { 00128 static int flag; 00129 if(flag) { 00130 led3 = 1; 00131 flag = 0; 00132 } else { 00133 led3 = 0; 00134 flag++; 00135 } 00136 /** 00137 * Usage of InPort with premitive type. 00138 */ 00139 if(in0In.isNew()) { 00140 in0In.read(); 00141 led4 = in0.data; 00142 } 00143 00144 return RTC_OK; 00145 } 00146 00147 00148 ////////////////////////////////////// 00149 // on_error 00150 // This function is repeatedly called when 00151 // the RTno is in the ERROR condition. 00152 // The ERROR condition can be recovered, 00153 // when the RTno is reset. 00154 /////////////////////////////////////// 00155 int RTno::onError() 00156 { 00157 return RTC_OK; 00158 } 00159 00160 //////////////////////////////////////// 00161 // This function is called when 00162 // the RTno is reset. If on_reset is 00163 // succeeded, the RTno will enter into 00164 // the INACTIVE condition. If failed 00165 // (return value is RTC_ERROR), RTno 00166 // will stay in ERROR condition.ec 00167 /////////////////////////////////////// 00168 int RTno::onReset() 00169 { 00170 return RTC_OK; 00171 }
Generated on Fri Jul 15 2022 15:09:46 by
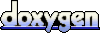