RTno is communicating library and framework which allows you to make your embedded device capable of communicating with RT-middleware world. RT-middleware is a platform software to realize Robotic system. In RTM, robots are developed by constructing robotics technologies\' elements (components) named RT-component. Therefore, the RTno helps you to create your own RT-component with your mbed and arduino. To know how to use your RTno device, visit here: http://ysuga.net/robot_e/rtm_e/rtc_e/1065?lang=en To know about RT-middleware and RT-component, visit http://www.openrtm.org
Dependents: RTnoV3_LED RTnoV3_Template RTnoV3_ADC RTnoV3_Timer ... more
RTnoProfile.cpp
00001 #define RTNO_SUBMODULE_DEFINE 00002 00003 #include "mbed.h" 00004 00005 #include "RTnoProfile.h" 00006 00007 static PortBase *m_ppInPort[MAX_PORT]; 00008 static PortBase *m_ppOutPort[MAX_PORT]; 00009 00010 void RTnoProfile_init() 00011 { 00012 } 00013 00014 int RTnoProfile_addInPort(PortBase* port) 00015 { 00016 int index = RTnoProfile_getNumInPort(); 00017 if(index == MAX_PORT) return -1; 00018 m_ppInPort[index] = port; 00019 return index; 00020 } 00021 00022 00023 int RTnoProfile_addOutPort(PortBase* port) 00024 { 00025 int index = RTnoProfile_getNumOutPort(); 00026 if(index == MAX_PORT) return -1; 00027 m_ppOutPort[index] = port; 00028 return index; 00029 } 00030 00031 int RTnoProfile_getNumInPort() { 00032 for(int i = 0;i < MAX_PORT;i++) { 00033 if(m_ppInPort[i] == NULL) { 00034 return i; 00035 } 00036 } 00037 return MAX_PORT; 00038 } 00039 00040 00041 int RTnoProfile_getNumOutPort() { 00042 for(int i = 0;i < MAX_PORT;i++) { 00043 if(m_ppOutPort[i] == NULL) { 00044 return i; 00045 } 00046 } 00047 return MAX_PORT; 00048 00049 } 00050 00051 PortBase* RTnoProfile_getInPort(const char* name, uint8_t nameLen) 00052 { 00053 for(uint8_t i = 0;i < MAX_PORT;i++) { 00054 if(m_ppInPort[i] == NULL) return NULL; 00055 if(strncmp(name, m_ppInPort[i]->pName, nameLen) == 0) { 00056 return m_ppInPort[i]; 00057 } 00058 } 00059 return NULL; 00060 } 00061 00062 00063 PortBase* RTnoProfile_getOutPort(const char* name, uint8_t nameLen) 00064 { 00065 for(uint8_t i = 0;i < MAX_PORT;i++) { 00066 if(m_ppOutPort[i] == NULL) return NULL; 00067 if(strncmp(name, m_ppOutPort[i]->pName, nameLen) == 0) { 00068 return m_ppOutPort[i]; 00069 } 00070 } 00071 return NULL; 00072 } 00073 00074 PortBase* RTnoProfile_getInPortByIndex(const uint8_t i) 00075 { 00076 return m_ppInPort[i]; 00077 } 00078 00079 PortBase* RTnoProfile_getOutPortByIndex(const uint8_t i) 00080 { 00081 return m_ppOutPort[i]; 00082 }
Generated on Tue Jul 12 2022 18:33:24 by
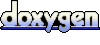