
Interrupt-driven keypad interface library. Compatible with RTOS. Able to handle various keypad size below 4x4.
main.cpp
00001 #include "mbed.h" 00002 00003 #include "Keypad.h" 00004 00005 Serial PC(USBTX, USBRX); 00006 00007 // Define your own keypad values 00008 char Keytable[] = { '1', '2', '3', // r0 00009 '4', '5', '6', // r1 00010 '7', '8', '9', // r2 00011 // c0 c1 c2 00012 }; 00013 00014 int32_t Index = -1; 00015 int State; 00016 00017 uint32_t cbAfterInput(uint32_t index) 00018 { 00019 Index = index; 00020 return 0; 00021 } 00022 00023 int main() 00024 { 00025 PC.printf("I am Demo Keypad\r\n"); 00026 00027 // r0 r1 r2 r3 c0 c1 c2 c3 00028 Keypad keypad(p21, p22, NC, NC, p23, p24, NC, NC); 00029 keypad.attach(&cbAfterInput); 00030 keypad.start(); // energize the columns c0-c3 of the keypad 00031 00032 while (1) { 00033 __wfi(); 00034 if (Index > -1) { 00035 PC.printf("Interrupted"); 00036 PC.printf("Index:%d => Key:%c\r\n", Index, Keytable[Index]); 00037 Index = -1; 00038 } 00039 } 00040 }
Generated on Fri Jul 15 2022 23:39:33 by
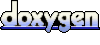