A library interface to shift register such as NXP 74HCT595
Dependents: DiscoTech filter_implement System_Project_V6 LM35functionshield ... more
ShiftReg.h
00001 /* mbed Shift Register Library, such as for NXP 74HC595 00002 * Copyright (c) 2012, YoongHM 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef _SHIFTREG_H 00024 #define _SHIFTREG_H 00025 00026 #include "mbed.h" 00027 00028 /** A interface to drive shifter register as such 74HCT595 00029 * 00030 * @code 00031 * #include "mbed.h" 00032 * #include "ShiftReg.h" 00033 * 00034 * ShiftReg HC595(p21, p22, p23); 00035 * 00036 * int main() { 00037 * // clear shift and store registers initially 00038 * HC595.ShiftByte(0x00, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00039 * 00040 * while(1) { 00041 * // Demostrate to shift in bit by bit 00042 * HC595.ShiftBit(1); HC595.Latch(); wait(0.2); 00043 * for (int i = 0; i < 8; i++) { 00044 * HC595.ShiftBit(0); HC595.Latch(); wait(0.2); 00045 * } 00046 00047 * // Demostrate to shift in byte-by-byte 00048 * // HC595.ShiftByte(0x80, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00049 * HC595.ShiftByte(0x40, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00050 * HC595.ShiftByte(0x20, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00051 * HC595.ShiftByte(0x10, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00052 * HC595.ShiftByte(0x08, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00053 * HC595.ShiftByte(0x04, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00054 * HC595.ShiftByte(0x02, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00055 * HC595.ShiftByte(0x01, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00056 * HC595.ShiftByte(0x00, ShiftReg::MSBFirst); HC595.Latch(); wait(0.2); 00057 * } 00058 * } 00059 * @endcode 00060 */ 00061 00062 class ShiftReg 00063 { 00064 public: 00065 /** Bit order out format */ 00066 enum BitOrd { 00067 MSBFirst = 0x80, /**< Most significant bit first */ 00068 LSBFirst = 0x01 /**< Least significant bit first */ 00069 }; 00070 00071 /** Create a ShiftReg interface to shift register 00072 * 00073 * @param data Pin to serial input to shift register 00074 * @param store Pin to store register 00075 * @param clock Pin to shift into register 00076 */ 00077 ShiftReg 00078 (PinName data 00079 ,PinName store 00080 ,PinName clock 00081 ); 00082 00083 /** Shift out 8-bit data via the serial pin 00084 * 00085 * @param data Data to be shifted out via the serial pin 00086 * @param order Bit order to shift out data. Default is MSBFirst 00087 */ 00088 void 00089 ShiftByte 00090 (int8_t data 00091 ,BitOrd ord = MSBFirst 00092 ); 00093 00094 /** Shift out 1-bit data via the serial pin 00095 * 00096 * @param data Data to be shifted out via the serial pin 00097 */ 00098 void 00099 ShiftBit 00100 (int8_t data = 0 00101 ); 00102 00103 /** Latch data out 00104 */ 00105 void 00106 Latch(); 00107 00108 private: 00109 DigitalOut _ds; // Serial in 00110 DigitalOut _st; // store register or latch 00111 DigitalOut _sh; // shift register 00112 BitOrd _ord; // Bit order to shift out data 00113 }; 00114 00115 #endif // _SHIFTREG_H
Generated on Tue Jul 12 2022 19:29:32 by
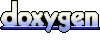