
For Arch or Xadow M0, use on-board button to provide multifunction.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // For Arch and Xadow M0, use on-board button for normal input function rather than reset function 00002 // single click - LED1 on 00003 // double click - LED2 on 00004 // long click - LED1 and LED2 on, and then the board will restart 00005 // 00006 // Note: To enter ISP mode, press the button and then power on (power-on reset) 00007 00008 #include "mbed.h" 00009 #include "pinmap.h" 00010 00011 #define BUTTON_DOWN 0 00012 00013 InterruptIn button(P0_1); // on-board button of Arch or Xadow M0 00014 BusOut leds(LED1, LED2); 00015 00016 uint8_t button_down_event = 0; 00017 00018 void button_callback() 00019 { 00020 button_down_event = 1; // set button down event flag 00021 } 00022 00023 int button_detect() 00024 { 00025 int t = 0; 00026 00027 while (1) { 00028 if (button.read() != BUTTON_DOWN) { 00029 if (t < 30) { 00030 return 0; // for anti shake 00031 } else { 00032 break; 00033 } 00034 } 00035 00036 if (t > 3000) { // More than 3 seconds 00037 return -1; // long click 00038 } 00039 00040 t++; 00041 wait_ms(1); 00042 } 00043 00044 if (t > 4000) { // More than 0.4 seconds 00045 return 1; // single click 00046 } 00047 00048 while (true) { 00049 if (button.read() == BUTTON_DOWN) { 00050 wait_ms(1); 00051 if (button.read() == BUTTON_DOWN) { 00052 return 2; // double click 00053 } 00054 00055 t += 10; 00056 } 00057 00058 if (t > 400) { 00059 return 1; // The interval of double click supposes to be less than 0.4 seconds, so it's single click 00060 } 00061 00062 t++; 00063 wait_ms(1); 00064 } 00065 } 00066 00067 00068 int main() { 00069 // start 00070 leds = 1; 00071 wait(0.2); 00072 leds = 3; 00073 wait(0.2); 00074 leds = 2; 00075 wait(0.2); 00076 leds = 0; 00077 00078 pin_function(P0_0, 1); // use RESET pin as 00079 button.fall(button_callback); 00080 00081 while(1) { 00082 if (button_down_event != 0) { 00083 int clicks = button_detect(); 00084 if (clicks == 1) { 00085 leds = 0x01; 00086 } else if (clicks == 2) { 00087 leds = 0x02; 00088 } else if (clicks == -1) { // long press 00089 leds = 0x03; 00090 while (button.read() == BUTTON_DOWN) { } // wait until button is released. note: remove this line to boot into ISP mode after reset 00091 NVIC_SystemReset(); // reset 00092 } 00093 00094 wait(1); 00095 leds = 0; 00096 00097 button_down_event = 0; // clear button down event flag 00098 } 00099 00100 // sleep(); 00101 // or do something else 00102 } 00103 }
Generated on Wed Jul 13 2022 01:09:53 by
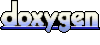