
to colorize a colorful pixel with a simple touch using nfc technology
Dependencies: Chainable_RGB_LED mbed
emulatetag.h
00001 /**************************************************************************/ 00002 /*! 00003 @file emulatetag.h 00004 @author Armin Wieser 00005 @license BSD 00006 00007 Implemented using NFC forum documents & library of libnfc 00008 */ 00009 /**************************************************************************/ 00010 00011 #ifndef __EMULATETAG_H__ 00012 #define __EMULATETAG_H__ 00013 00014 #include "PN532.h " 00015 00016 #define NDEF_MAX_LENGTH 128 // altough ndef can handle up to 0xfffe in size, arduino cannot. 00017 typedef enum {COMMAND_COMPLETE, TAG_NOT_FOUND, FUNCTION_NOT_SUPPORTED, MEMORY_FAILURE, END_OF_FILE_BEFORE_REACHED_LE_BYTES} responseCommand; 00018 00019 class EmulateTag{ 00020 00021 public: 00022 EmulateTag(PN532Interface &interface) : pn532(interface), uidPtr(0), tagWrittenByInitiator(false), tagWriteable(true), updateNdefCallback(0) { } 00023 00024 bool init(); 00025 00026 bool emulate(const uint16_t tgInitAsTargetTimeout = 0); 00027 00028 /* 00029 * @param uid pointer to byte array of length 3 (uid is 4 bytes - first byte is fixed) or zero for uid 00030 */ 00031 void setUid(uint8_t* uid = 0); 00032 00033 void setNdefFile(const uint8_t* ndef, const int16_t ndefLength); 00034 00035 void getContent(uint8_t** buf, uint16_t* length){ 00036 *buf = ndef_file + 2; // first 2 bytes = length 00037 *length = (ndef_file[0] << 8) + ndef_file[1]; 00038 } 00039 00040 bool writeOccured(){ 00041 return tagWrittenByInitiator; 00042 } 00043 00044 void setTagWriteable(bool setWriteable){ 00045 tagWriteable = setWriteable; 00046 } 00047 00048 uint8_t* getNdefFilePtr(){ 00049 return ndef_file; 00050 } 00051 00052 uint8_t getNdefMaxLength(){ 00053 return NDEF_MAX_LENGTH; 00054 } 00055 00056 void attach(void (*func)(uint8_t *buf, uint16_t length)) { 00057 updateNdefCallback = func; 00058 }; 00059 00060 private: 00061 PN532 pn532; 00062 uint8_t ndef_file[NDEF_MAX_LENGTH]; 00063 uint8_t* uidPtr; 00064 bool tagWrittenByInitiator; 00065 bool tagWriteable; 00066 void (*updateNdefCallback)(uint8_t *ndef, uint16_t length); 00067 00068 void setResponse(responseCommand cmd, uint8_t* buf, uint8_t* sendlen, uint8_t sendlenOffset = 0); 00069 }; 00070 00071 #endif
Generated on Fri Jul 15 2022 06:47:11 by
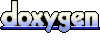