
initial
Embed:
(wiki syntax)
Show/hide line numbers
mbed_i2c.h
Go to the documentation of this file.
00001 00002 /****************************************************************************** 00003 * $Id: mbed_i2c.h $ 00004 *****************************************************************************/ 00005 /** 00006 * @defgroup MBED_System_Layer MBED System Layer 00007 * @brief MBED System Layer APIs. 00008 * To interface with any platform, eMPL needs access to various 00009 * system layer functions. 00010 * 00011 * @{ 00012 * @file mbed_i2c.h 00013 * @brief Serial communication functions needed by eMPL to 00014 * communicate to the MPU devices. 00015 * @details This driver assumes that eMPL is with a sub-master clock set 00016 * to 20MHz. The following MBEDs are supported: 00017 */ 00018 #ifndef _MBED_I2C_H_ 00019 #define _MBED_I2C_H_ 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #include "i2c_api.h" 00026 00027 void mbed_i2c_init(PinName sda, PinName scl); 00028 00029 /** 00030 * @brief Set up the I2C port and configure the MBED as the master. 00031 * @return 0 if successful. 00032 */ 00033 void mbed_i2c_enable(void); 00034 /** 00035 * @brief Disable I2C communication. 00036 * This function will disable the I2C hardware and should be called prior to 00037 * entering low-power mode. 00038 * @return 0 if successful. 00039 */ 00040 void mbed_i2c_disable(void); 00041 00042 /** 00043 * @brief Write to a device register. 00044 * 00045 * @param[in] slave_addr Slave address of device. 00046 * @param[in] reg_addr Slave register to be written to. 00047 * @param[in] length Number of bytes to write. 00048 * @param[out] data Data to be written to register. 00049 * 00050 * @return 0 if successful. 00051 */ 00052 int mbed_i2c_write(unsigned char slave_addr, 00053 unsigned char reg_addr, 00054 unsigned char length, 00055 unsigned char const *data); 00056 /** 00057 * @brief Read from a device. 00058 * 00059 * @param[in] slave_addr Slave address of device. 00060 * @param[in] reg_addr Slave register to be read from. 00061 * @param[in] length Number of bytes to read. 00062 * @param[out] data Data from register. 00063 * 00064 * @return 0 if successful. 00065 */ 00066 int mbed_i2c_read(unsigned char slave_addr, 00067 unsigned char reg_addr, 00068 unsigned char length, 00069 unsigned char *data); 00070 00071 int mbed_i2c_clear(PinName sda, PinName scl); 00072 00073 #ifdef __cplusplus 00074 } 00075 #endif 00076 00077 #endif /* _MBED_I2C_H_ */ 00078 00079 /** 00080 * @} 00081 */
Generated on Wed Jul 13 2022 20:01:33 by
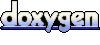