
Accelerometer game
Dependencies: 4DGL-uLCD-SE ADXL345 mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "uLCD_4DGL.h" 00004 #include "ADXL345.h" 00005 00006 00007 PwmOut speaker(p21); //create a PwmOut object for the speaker 00008 uLCD_4DGL uLCD(p28, p27, p29); // create a global lcd object 00009 ADXL345 accelerometer(p5, p6, p7, p8);//create a global accelerometer object 00010 Serial pc(USBTX, USBRX);// for debugging 00011 Mutex lcd_mutex;// Create the mutex lock object 00012 00013 int red_car_x, red_car_y,blue_car_x,blue_car_y,frog_x,frog_y,i,j; 00014 int x=0,y=0; 00015 void thread1(void const *args)//thread 1 moves the red car 00016 { 00017 00018 while(true) { 00019 //check for boundary conditions 00020 if(red_car_x>127){ 00021 red_car_x=-16; 00022 red_car_y=32; 00023 00024 } 00025 lcd_mutex.lock();//acquire lock 00026 uLCD.filled_rectangle(red_car_x,red_car_y,red_car_x+15,red_car_y+15,0x000000);//erase previous position 00027 red_car_x=red_car_x+16; 00028 00029 for(i=0;i<10;i++){ 00030 uLCD.filled_rectangle(red_car_x,red_car_y,red_car_x+15,red_car_y+15,0xFF3300);//change position 00031 00032 } 00033 00034 lcd_mutex.unlock();//release the lock 00035 Thread::wait(500); 00036 00037 } 00038 } 00039 00040 00041 void thread2(void const *args)//thread 1 moves the blue car, code similar to thread 1 00042 { 00043 00044 while(true) { 00045 00046 if(blue_car_x>127){ 00047 blue_car_x=-16; 00048 blue_car_y=64; 00049 00050 } 00051 lcd_mutex.lock(); 00052 uLCD.filled_rectangle(blue_car_x,blue_car_y,blue_car_x+15,blue_car_y+15,0x000000); 00053 blue_car_x=blue_car_x+16; 00054 for(i=0;i<5;i++){ 00055 uLCD.filled_rectangle(blue_car_x,blue_car_y,blue_car_x+15,blue_car_y+15,BLUE);//change position 00056 00057 } 00058 00059 lcd_mutex.unlock(); 00060 Thread::wait(200); 00061 00062 } 00063 } 00064 00065 void thread3(void const *args)//thread 3 plays the sound effects 00066 { 00067 00068 00069 while(true) { 00070 00071 speaker.period(1.0/150.0); // 500hz period 00072 speaker =0.25; //25% duty cycle - mid range volume 00073 wait(.02); 00074 speaker=0.0;//stop the speaker 00075 00076 Thread::wait(500); 00077 00078 } 00079 } 00080 00081 int main() 00082 { 00083 00084 A:uLCD.cls();// clear the LCD 00085 00086 uLCD.text_width(2); //2X size text 00087 uLCD.text_height(2); 00088 00089 00090 uLCD.locate(2,2); 00091 00092 uLCD.printf("\n Frogger-G\n");//display the Title 00093 wait(0.1); 00094 00095 // Sound effects at the start of the game 00096 for (i=0; i<500; i=i+100) { 00097 speaker.period(1.0/float(i)); 00098 speaker=0.25; 00099 wait(.1); 00100 } 00101 wait(2); 00102 uLCD.cls(); 00103 uLCD.text_width(2); //2X size text 00104 uLCD.text_height(2); 00105 uLCD.locate(0,0); 00106 uLCD.color( 0xFF00FF); 00107 uLCD.printf("\n Help the frog \n cross \nthe road!\n"); 00108 wait (2); 00109 00110 uLCD.cls(); 00111 00112 00113 red_car_x=0; 00114 red_car_y=32; 00115 blue_car_x=0; 00116 blue_car_y=64; 00117 frog_x=32; 00118 frog_y=96; 00119 //initialize the frog position 00120 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x99FF00); 00121 uLCD.filled_rectangle(red_car_x,red_car_y,red_car_x+15,red_car_y+15,0xff3300); 00122 uLCD.filled_rectangle(blue_car_x,blue_car_y,blue_car_x+15,blue_car_y+15,BLUE); 00123 00124 int readings[3] = {0, 0, 0}; 00125 00126 //Go into standby mode to configure the device. 00127 accelerometer.setPowerControl(0x00); 00128 00129 //Full resolution, +/-16g, 4mg/LSB. 00130 accelerometer.setDataFormatControl(0x0B); 00131 00132 //3.2kHz data rate. 00133 accelerometer.setDataRate(ADXL345_3200HZ); 00134 00135 //Measurement mode. 00136 accelerometer.setPowerControl(0x08); 00137 //Initialize all threads 00138 Thread t1(thread1); 00139 Thread t2(thread2); 00140 Thread t3(thread3); 00141 00142 while (1) { 00143 00144 00145 for(i=0;i<5;i++) 00146 //average accelerometer readings for accuracy 00147 { 00148 accelerometer.getOutput(readings); 00149 pc.printf("%i, %i, %i ", (int16_t)readings[0], (int16_t)readings[1], (int16_t)readings[2]); 00150 x=x+int16_t(readings[0]); 00151 y=y+int16_t(readings[1]); 00152 } 00153 x=int(x/5); 00154 y=int(y/5); 00155 00156 //debug 00157 pc.printf("%d, %d ", int(x),int(y) ); 00158 00159 //move the frog according to accelerometer readings 00160 //thresholds obtained through trial and error 00161 //move right 00162 if(y>0){ 00163 lcd_mutex.lock(); 00164 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x000000); 00165 frog_x=frog_x+16; 00166 00167 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x99FF00); 00168 lcd_mutex.unlock(); 00169 } 00170 //move up 00171 if(x<70){ 00172 lcd_mutex.lock(); 00173 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x000000); 00174 frog_y=frog_y-16; 00175 00176 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x99FF00); 00177 lcd_mutex.unlock(); 00178 } 00179 //move left 00180 if(y<-70){ 00181 lcd_mutex.lock(); 00182 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x000000); 00183 frog_x=frog_x-16; 00184 00185 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x99FF00); 00186 lcd_mutex.unlock(); 00187 } 00188 //move down 00189 if(x>180){ 00190 lcd_mutex.lock(); 00191 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x000000); 00192 frog_y=frog_y+16; 00193 00194 uLCD.filled_rectangle(frog_x,frog_y,frog_x+15,frog_y+15,0x99FF00); 00195 lcd_mutex.unlock(); 00196 } 00197 00198 //check collisions 00199 if ((frog_x==red_car_x & frog_y==red_car_y)|(frog_x==blue_car_x & frog_y==blue_car_y)) 00200 { 00201 lcd_mutex.lock(); 00202 uLCD.cls(); 00203 00204 uLCD.text_width(2); //2X size text 00205 uLCD.text_height(2); 00206 uLCD.color(RED); 00207 uLCD.locate(2,2); 00208 uLCD.printf("\n GAME \n OVER!\n");// display message 00209 speaker.period(1.0/500.0); // 500hz period 00210 speaker =0.5; //50% duty cycle - max volume 00211 wait(3); 00212 speaker=0.0; // turn off audio 00213 00214 wait(2); 00215 lcd_mutex.unlock(); 00216 goto A;//restart the game 00217 } 00218 //check if frog has crossed the road 00219 if (frog_y<32) 00220 { 00221 00222 lcd_mutex.lock(); 00223 uLCD.cls(); 00224 00225 uLCD.text_width(2); //2X size text 00226 uLCD.text_height(2); 00227 uLCD.color(0xffff00); 00228 uLCD.locate(2,2); 00229 uLCD.printf("\n You \n WIN!\n");//display message 00230 00231 for (i=0; i<500; i=i+100) { 00232 speaker.period(1.0/float(i)); 00233 speaker=0.25; 00234 wait(.1); 00235 } 00236 wait(5); 00237 lcd_mutex.unlock(); 00238 goto A;//restart the game 00239 } 00240 00241 00242 Thread::wait(50); // wait 0.5s 00243 } 00244 00245 00246 00247 }
Generated on Fri Jul 15 2022 21:36:02 by
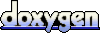