XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
Tx16TransmitOptions.cpp
00001 #include "Tx16TransmitOptions.h" 00002 00003 Tx16TransmitOptions::Tx16TransmitOptions() { } 00004 00005 Tx16TransmitOptions::Tx16TransmitOptions(unsigned char value):OptionsBase(value) 00006 { } 00007 00008 Tx16TransmitOptions::Tx16TransmitOptions(bool disable_retries_and_route_repair, bool force_long_header, bool disable_long_header, bool invoke_traceroute) 00009 { 00010 value = 0x00; 00011 if (disable_retries_and_route_repair) 00012 value |= 0x01; 00013 if (force_long_header) 00014 value |= 0x02; 00015 if (disable_long_header) 00016 value |= 0x04; 00017 if (invoke_traceroute) 00018 value |= 0x08; 00019 } 00020 00021 Tx16TransmitOptions * Tx16TransmitOptions::ForceLongHeader = new Tx16TransmitOptions(0x02); 00022 00023 Tx16TransmitOptions * Tx16TransmitOptions::DisableLongHeader = new Tx16TransmitOptions(0x04); 00024 00025 Tx16TransmitOptions * Tx16TransmitOptions::InvokeTraceroute = new Tx16TransmitOptions(0x08); 00026 00027 bool Tx16TransmitOptions::getForceLongHeader() 00028 { 00029 if ((value & 0x02) == 0x02) 00030 return true; 00031 else return false; 00032 } 00033 00034 void Tx16TransmitOptions::setForceLongHeader(bool status) 00035 { 00036 if (status) 00037 value |= 0x02; 00038 else 00039 value &= 0xFD; 00040 } 00041 00042 bool Tx16TransmitOptions::getDisableLongHeader() 00043 { 00044 if ((value & 0x04) == 0x04) 00045 return true; 00046 else return false; 00047 } 00048 00049 void Tx16TransmitOptions::setDisableLongHeader(bool status) 00050 { 00051 if (status) 00052 value |= 0x04; 00053 else 00054 value &= 0xFB; 00055 } 00056 00057 bool Tx16TransmitOptions::getInvokeTraceroute() 00058 { 00059 if ((value & 0x08) == 0x08) 00060 return true; 00061 else return false; 00062 } 00063 00064 void Tx16TransmitOptions::setInvokeTraceroute(bool status) 00065 { 00066 if (status) 00067 value |= 0x08; 00068 else 00069 value &= 0xF7; 00070 }
Generated on Tue Jul 12 2022 18:56:10 by
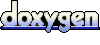