XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
NodeIdentificationIndicator.cpp
00001 #include "NodeIdentificationIndicator.h" 00002 00003 NodeIdentificationIndicator::NodeIdentificationIndicator(APIFrame * frame) 00004 : RxBase(frame) 00005 { 00006 offset = getPosition() - 8; 00007 } 00008 00009 int NodeIdentificationIndicator::getReceiveStatus() 00010 { 00011 return data[11]; 00012 } 00013 00014 Address NodeIdentificationIndicator::getRemoteDevice() 00015 { 00016 return Address(data + 14, data + 8); 00017 } 00018 00019 Address NodeIdentificationIndicator::getSenderDevice() 00020 { 00021 return Address(data + 1); 00022 } 00023 00024 unsigned char * NodeIdentificationIndicator::getNIString() 00025 { 00026 int length = getPosition() - 31; 00027 00028 if (length <= 0) 00029 return NULL; 00030 00031 return data + 22; 00032 } 00033 00034 int NodeIdentificationIndicator::getParentNetworkAddress() 00035 { 00036 return (data[offset] << 8) | data[offset + 1]; 00037 } 00038 00039 int NodeIdentificationIndicator::getDeviceType() 00040 { 00041 return data[offset + 2]; 00042 } 00043 00044 int NodeIdentificationIndicator::getSourceEvent() 00045 { 00046 return data[offset + 3]; 00047 } 00048 00049 int NodeIdentificationIndicator::getDigiProfileID() 00050 { 00051 return (data[offset + 4] << 8) | data[offset + 5]; 00052 } 00053 00054 int NodeIdentificationIndicator::getManufacturerID() 00055 { 00056 return (data[offset + 6] << 8) | data[offset + 7]; 00057 } 00058 00059 bool NodeIdentificationIndicator::convert(APIFrame * frame) 00060 { 00061 if (frame == NULL) 00062 return false; 00063 00064 if (frame->getFrameType() != APIFrame::Node_Identification_Indicator) 00065 return false; 00066 00067 return APIFrame::convert(frame); 00068 }
Generated on Tue Jul 12 2022 18:56:10 by
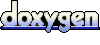