XBee and XBee-PRO ZigBee RF modules provide cost-effective wireless connectivity to electronic devices. They are interoperable with other ZigBee PRO feature set devices, including devices from other vendors.
IOSamples.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_IOSamples 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_IOSamples 00003 00004 #include "Pin.h" 00005 #include <map> 00006 00007 using namespace std; 00008 /** 00009 * A collection of digital and analog samples reported by XBee module. 00010 */ 00011 class IOSamples 00012 { 00013 private : 00014 unsigned int SUPPLY_VOLTAGE; 00015 00016 map<Pin *, unsigned int> analog; 00017 00018 map<Pin *, unsigned char> digital; 00019 00020 public: 00021 /** 00022 * Get all the avaliable analog values. 00023 * 00024 * @returns map<Pin,int> 00025 */ 00026 map<Pin *, unsigned int> * getAnalogs(); 00027 00028 /** 00029 * Get analog value from one pin. 00030 * 00031 * @param Pin XBee S1 or S2 pin object. 00032 * @returns 0xFFFF means sample not avaliable. 00033 */ 00034 unsigned int getAnalog(Pin * pin); 00035 00036 /** 00037 * Get all the avaliable digital values. 00038 * 00039 * @returns map<Pin,unsigned char>: 00040 * LOW = 0, 00041 * HIGH = 1, 00042 * UNMONITORED = 2, 00043 */ 00044 map<Pin *, unsigned char> * getDigitals(); 00045 00046 /** 00047 * Get digital value from one pin. 00048 * 00049 * @param Pin XBee S1 or S2 pin object. 00050 * @returns 00051 * LOW = 0, 00052 * HIGH = 1, 00053 * UNMONITORED = 2, 00054 */ 00055 unsigned char getDigital(Pin * pin); 00056 00057 /** 00058 * Get supply voltage, S2 only. 00059 * 00060 * @returns voltage 00061 */ 00062 unsigned int getSupplyVoltage(); 00063 00064 /** 00065 * Set supply voltage, S2 only. 00066 * 00067 * @param voltage 00068 */ 00069 void setSupplyVoltage(unsigned int voltage); 00070 00071 /** 00072 * Cleat all the sample values. 00073 */ 00074 void clear(); 00075 }; 00076 00077 #endif
Generated on Tue Jul 12 2022 18:56:10 by
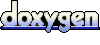