LV8548 Motor Driver Stepper Motor Dc MOtor
Dependents: LV8548_ON_MD_Modlle_kit_DCMtr_And_STEPMtr
LV8548.h
00001 /** 00002 [LV8548.h] 00003 Copyright (c) [2018] [YAMASHO] 00004 This software is released under the MIT License. 00005 http://opensource.org/licenses/mit-license.php 00006 */ 00007 00008 #ifndef MBED_LV8548_H 00009 #define MBED_LV8548_H 00010 00011 #include "mbed.h" 00012 00013 #define MAXCH (2) 00014 00015 #define STEPMAX_FREQ (4800) 00016 #define USE_PWM_PORT (1) // if use stepper then (0) 00017 00018 /** Motor Use */ 00019 enum DriverType { 00020 DCMOTOR /** DC Motor x2 */ 00021 , STEPERMOTOR /** STEPER x1 */ 00022 }; 00023 00024 enum DriverPwmMode { 00025 FWD_OPEN, /** FWD_OPEN */ 00026 FWD_BRAKE, /** FWD_BREAKE */ 00027 RVS_OPEN, /** REVERS OPEN */ 00028 RVS_BRAKE /** REVSRS BRAKE */ 00029 }; 00030 00031 enum DriverPwmOut { 00032 OUTPUT_OFF, /* OFF */ 00033 OUTPUT_START, /* START */ 00034 OUTPUT_BRAKE /* BRAAKE */ 00035 }; 00036 00037 /** Motor Number */ 00038 enum DriverMotorNumber { 00039 MOTOR_A = 0 00040 , MOTOR_B = 1 00041 , MOTOR_MAX 00042 }; 00043 00044 00045 /** Motor Dirction */ 00046 enum DriverMotorDirction { 00047 DIR_CW = 0 00048 , DIR_CCW = 1 00049 , DIR_MAX 00050 }; 00051 00052 /** Motor Step Mode */ 00053 enum DriverStepMode { 00054 FULLSTEP = 0 00055 , HALFSTEP = 1 00056 , MOTORSTEP_MAX 00057 }; 00058 00059 /** Motor Step Mode */ 00060 enum DriverPhaseStep { 00061 STEPPHASE0 = 0, 00062 STEPPHASE1 = 1, 00063 STEPPHASE2 = 2, 00064 STEPPHASE3 = 3, 00065 STEPPHASE4 = 4, 00066 STEPPHASE5 = 5, 00067 STEPPHASE6 = 6, 00068 STEPPHASE7 = 7, 00069 STEPPHASEMAX = 8 /* 必ず8 */ 00070 }; 00071 00072 class LV8548 { 00073 public: 00074 LV8548(PinName in1, PinName in2, PinName in3, PinName in4, DriverType drivertype = DCMOTOR ,uint16_t baseus = 64); 00075 #if USE_PWM_PORT 00076 PwmOut _in1,_in2,_in3,_in4; 00077 #else 00078 DigitalOut _in1,_in2,_in3,_in4; 00079 #endif 00080 DriverType _Drivertype; 00081 uint16_t _baseus; 00082 /***************************************************************************************************/ 00083 DriverPwmMode _Pwmmode[MOTOR_MAX]; // pwmのモード FWD.OPEN FWD.BRAKE RVS.OPEN REV.BRAKE 00084 DriverPwmOut _Pwmout [MOTOR_MAX]; // Start Stop Breke 00085 float _PwmPeriod [MOTOR_MAX]; // ポートのPwm周波数 00086 float _PwmDuty [MOTOR_MAX]; // 各Motor のduty 1.0f-0.0fまで 00087 // uint8_t _testx; 00088 00089 Ticker _Lv8548BaseTimer; 00090 00091 void SetDcPwmFreqency( uint8_t ch ,float Freq ); 00092 void SetDcPwmMode( uint8_t ch ,DriverPwmMode Mode ); 00093 void SetDcPwmDuty( uint8_t ch ,float Duty ); // 01まで 00094 void SetDcOutPut ( uint8_t ch ,DriverPwmOut Mode ); 00095 void SetDcMotor ( uint8_t ch ); 00096 void SetDcMotorStop( uint8_t ch ); 00097 /*******************************************************************************************************************/ 00098 float _StepAngle; 00099 float _StepDeg; // 角度 0-360度 00100 float _StepFreq; // 周波数 00101 float _TimerCounter; 00102 volatile uint8_t _StepPhase; // Step Motorのphase 00103 volatile uint32_t _IntTimerCount; 00104 // volatile uint32_t _PhaseCounter; 00105 volatile uint32_t _StepTimeCount; 00106 volatile bool _StepOperation; // 割込内の動作状況 00107 volatile DriverStepMode _TargetStepMode; // 目標のStep状態 Full(0)/Half(1) 00108 volatile DriverStepMode _NowStepMode; // 動作中のStep状態 Full(0)/Half(1) 00109 volatile DriverMotorDirction _TragetStepDirection; // 目標のDirection Cw/Ccw 00110 volatile DriverMotorDirction _NowStepDirection; // 動作中のDirection cw/CCW 00111 volatile uint32_t _NowStep; // 現状のStep 00112 volatile uint32_t _TargetStep; // ターゲットのStep 00113 void SetStepAngle( uint16_t Angle); // 角度の設定 00114 void SetStepDeg ( uint16_t frequency , uint16_t deg , uint8_t Direction, uint8_t StepMode); 00115 void SetStepTime ( uint16_t frequency , uint16_t time , uint8_t Direction, uint8_t StepMode); 00116 void SetStepStep ( uint16_t frequency , uint16_t step , uint8_t Direction, uint8_t StepMode); 00117 void SetStepStop ( void ); 00118 void SetStepFree ( void ); 00119 void SetStepMotor( void ); 00120 void SetStepTimerInt ( void ); 00121 }; 00122 00123 00124 #endif
Generated on Wed Jul 13 2022 23:00:39 by
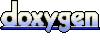