XBee API mode library 1.0
Embed:
(wiki syntax)
Show/hide line numbers
Send.cpp
00001 /* 00002 Copyright (c) 2011, Senio Networks, Inc. 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include "XBee.h" 00024 #include <stdarg.h> 00025 00026 void XBee::sendCommand(const char *command, int8_t param, bool queue) { 00027 sendCommand(command, (uint64_t) param, queue); 00028 } 00029 00030 void XBee::sendCommand(const char *command, int16_t param, bool queue) { 00031 sendCommand(command, (uint64_t) param, queue); 00032 } 00033 00034 void XBee::sendCommand(const char *command, int32_t param, bool queue) { 00035 sendCommand(command, (uint64_t) param, queue); 00036 } 00037 00038 void XBee::sendCommand(const char *command, int64_t param, bool queue) { 00039 sendCommand(command, (uint64_t) param, queue); 00040 } 00041 00042 void XBee::sendCommand(const char *command, uint8_t param, bool queue) { 00043 sendCommand(command, (uint64_t) param, queue); 00044 } 00045 00046 void XBee::sendCommand(const char *command, uint16_t param, bool queue) { 00047 sendCommand(command, (uint64_t) param, queue); 00048 } 00049 00050 void XBee::sendCommand(const char *command, uint32_t param, bool queue) { 00051 sendCommand(command, (uint64_t) param, queue); 00052 } 00053 00054 void XBee::sendCommand(const char *command, uint64_t param, bool queue) { 00055 uint8_t param_buf[8] = { 00056 (uint8_t) (param >> 56), (uint8_t) (param >> 48), 00057 (uint8_t) (param >> 40), (uint8_t) (param >> 32), 00058 (uint8_t) (param >> 24), (uint8_t) (param >> 16), 00059 (uint8_t) (param >> 8), (uint8_t) param 00060 }; 00061 00062 int n = 0; 00063 while (n < 7 && param_buf[n] == 0) n++; 00064 00065 sendCommand(command, param_buf + n, 8 - n, queue); 00066 } 00067 00068 void XBee::sendCommand(const char *command, const char *param, bool queue) { 00069 sendCommand(command, (uint8_t *) param, strlen(param), queue); 00070 } 00071 00072 void XBee::sendCommand(const char *command, const uint8_t *param, int param_length, bool queue) { 00073 char buf[param_length + 4]; 00074 00075 createAtRequest(frame_id, command, param, param_length, queue, buf, sizeof(buf)); 00076 sendFrame(buf, param_length + 4); 00077 frame_id = frame_id % 255 + 1; 00078 } 00079 00080 void XBee::sendRemoteCommand(const char *command, int8_t param) { 00081 sendRemoteCommand(command, (uint64_t) param); 00082 } 00083 00084 void XBee::sendRemoteCommand(const char *command, int16_t param) { 00085 sendRemoteCommand(command, (uint64_t) param); 00086 } 00087 00088 void XBee::sendRemoteCommand(const char *command, int32_t param) { 00089 sendRemoteCommand(command, (uint64_t) param); 00090 } 00091 00092 void XBee::sendRemoteCommand(const char *command, int64_t param) { 00093 sendRemoteCommand(command, (uint64_t) param); 00094 } 00095 00096 void XBee::sendRemoteCommand(const char *command, uint8_t param) { 00097 sendRemoteCommand(command, (uint64_t) param); 00098 } 00099 00100 void XBee::sendRemoteCommand(const char *command, uint16_t param) { 00101 sendRemoteCommand(command, (uint64_t) param); 00102 } 00103 00104 void XBee::sendRemoteCommand(const char *command, uint32_t param) { 00105 sendRemoteCommand(command, (uint64_t) param); 00106 } 00107 00108 void XBee::sendRemoteCommand(const char *command, uint64_t param) { 00109 uint8_t param_buf[8] = { 00110 (uint8_t) (param >> 56), (uint8_t) (param >> 48), 00111 (uint8_t) (param >> 40), (uint8_t) (param >> 32), 00112 (uint8_t) (param >> 24), (uint8_t) (param >> 16), 00113 (uint8_t) (param >> 8), (uint8_t) param 00114 }; 00115 00116 int n = 0; 00117 while (n < 7 && param_buf[n] == 0) n++; 00118 00119 sendRemoteCommand(command, param_buf + n, 8 - n); 00120 } 00121 00122 void XBee::sendRemoteCommand(const char *command, const char *param) { 00123 sendRemoteCommand(command, (uint8_t *) param, strlen(param)); 00124 } 00125 00126 void XBee::sendRemoteCommand(const char *command, const uint8_t *param, int param_length, char options) { 00127 char buf[param_length + 15]; 00128 00129 createRemoteAtRequest(frame_id, command, param, param_length, options, buf, sizeof(buf)); 00130 sendFrame(buf, param_length + 15); 00131 frame_id = frame_id % 255 + 1; 00132 } 00133 00134 bool XBee::send(const char *data, int length, bool confirm = true) { 00135 if (length == 0) 00136 length = strlen(data); 00137 00138 char buf[length + 14]; 00139 00140 createTxRequest(frame_id, data, length, buf, sizeof(buf)); 00141 sendFrame(buf, length + 14); 00142 frame_id = frame_id % 255 + 1; 00143 00144 if (confirm) { 00145 int index = seekFor(ZigBeeTransmitStatus, 3.0); 00146 if (index != -1) { 00147 char delivery; 00148 scan(index, DeliveryStatus, &delivery); 00149 buf[INDEX(index + 2)] = 0x00; 00150 return delivery == 0; 00151 } 00152 } 00153 00154 return false; 00155 } 00156 00157 bool XBee::sendConfirm(const char *data, int length) { 00158 return send(data, length, true); 00159 } 00160 00161 int XBee::printf(const char *format, ...) { 00162 va_list argv; 00163 va_start(argv, format); 00164 char buf[256]; 00165 int length = vsnprintf(buf, sizeof buf, format, argv); 00166 return length > 0 && sendConfirm(buf, length) ? length : -1; 00167 } 00168 00169 void XBee::send(char c) { 00170 while (!writeable()) wait_us(100); 00171 putc(c); 00172 } 00173 00174 void XBee::send2(char c) { 00175 switch (c) { 00176 case 0x7E: 00177 case 0x7D: 00178 case 0x11: 00179 case 0x13: 00180 send(ESCAPE); 00181 send(c ^ 0x20); 00182 break; 00183 default: 00184 send(c); 00185 } 00186 } 00187 00188 void XBee::sendFrame(const char *frame, int length) { 00189 char checksum = 255; 00190 if (debug) leds = leds | 8; //**LEDS=1xxx 00191 00192 send(PREAMBLE); 00193 00194 if (apiMode == 2) { 00195 send2((length >> 8) & 255); 00196 send2(length & 255); 00197 for (int i = 0; i < length; i++) { 00198 send2(frame[i]); 00199 checksum -= frame[i]; 00200 } 00201 send2(checksum); 00202 } else { 00203 send((length >> 8) & 255); 00204 send(length & 255); 00205 for (int i = 0; i < length; i++) { 00206 send(frame[i]); 00207 checksum -= frame[i]; 00208 } 00209 send(checksum); 00210 } 00211 if (debug) leds = leds & 7; //**LEDS=0xxx 00212 } 00213 00214 int XBee::createTxRequest(char frame_id, const char *data, int data_length, char *buf, int buf_size) { 00215 if (data_length + 14 > buf_size) return -1; 00216 00217 buf[0] = 0x10; // frame type 00218 buf[1] = frame_id; 00219 for (int i = 0; i < 8; i++) buf[i + 2] = destination64[i]; 00220 buf[10] = destination16[0]; // 16 bit dest address 00221 buf[11] = destination16[1]; // 16 bit dest address 00222 buf[12] = 0; 00223 buf[13] = 0; 00224 for (int i = 0; i < data_length; i++) buf[i + 14] = data[i]; 00225 00226 return data_length + 14; 00227 } 00228 00229 int XBee::createAtRequest(char frame_id, const char *command, const uint8_t *param, int param_length, bool queue, char *buf, int buf_size) { 00230 if (param_length + 4 > buf_size) return -1; 00231 00232 buf[0] = queue ? 0x09 : 0x08; // frame type 00233 buf[1] = frame_id; 00234 buf[2] = command[0]; 00235 buf[3] = command[1]; 00236 for (int i = 0; i < param_length; i++) buf[i + 4] = param[i]; 00237 00238 return param_length + 4; 00239 } 00240 00241 int XBee::createRemoteAtRequest(char frame_id, const char *command, const uint8_t *param, int param_length, char options, char *buf, int buf_size) { 00242 if (param_length + 4 > buf_size) return -1; 00243 00244 buf[0] = 0x17; // frame type 00245 buf[1] = frame_id; 00246 memcpy(buf + 2, destination64, 8); 00247 buf[10] = destination16[0]; 00248 buf[11] = destination16[1]; 00249 buf[12] = options; 00250 buf[13] = command[0]; 00251 buf[14] = command[1]; 00252 for (int i = 0; i < param_length; i++) buf[i + 15] = param[i]; 00253 00254 return param_length + 15; 00255 }
Generated on Wed Jul 20 2022 22:15:57 by
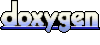