DisplayAPP_Base
Dependents: GR-Boads_Camera_DisplayApp_base GR-Boads_Camera_DisplayApp_base DisplayApp LED01
DisplayApp.h
00001 /**************************************************************************//** 00002 * @file DisplayApp.h 00003 * @brief DisplayApp API 00004 ******************************************************************************/ 00005 00006 #ifndef DISPLAY_APP_H 00007 #define DISPLAY_APP_H 00008 00009 #include "mbed.h" 00010 #include "rtos.h" 00011 #include "USBSerial.h" 00012 00013 /** A class to communicate a DisplayApp 00014 * 00015 */ 00016 class DisplayApp { 00017 public: 00018 00019 USBSerial PcApp; 00020 /** Touch position structure */ 00021 typedef struct { 00022 uint32_t x; /**< The position of the x-coordinate. */ 00023 uint32_t y; /**< The position of the y-coordinate. */ 00024 bool valid; /**< Whether a valid data.. */ 00025 } touch_pos_t; 00026 00027 /** Constructor: Initializes DisplayApp. 00028 * 00029 * @param tsk_pri Priority of the thread function. (default: osPriorityNormal). 00030 * @param init_pri Priority of before the USB is connected. (default: osPriorityLow). 00031 * @param stack_size stack size (in bytes) requirements for the thread function. (default: DEFAULT_STACK_SIZE). 00032 */ 00033 DisplayApp(osPriority tsk_pri = osPriorityNormal, uint32_t stack_size = DEFAULT_STACK_SIZE); 00034 00035 /** Send RGB888 data 00036 * 00037 * @param buf data buffer address 00038 * @param pic_width picture width 00039 * @param pic_height picture height 00040 * @return send data size 00041 */ 00042 int SendRgb888(uint8_t * buf, uint32_t pic_width, uint32_t pic_height); 00043 00044 /** Send JPEG data 00045 * 00046 * @param buf data buffer address 00047 * @param size data size 00048 * @return send data size 00049 */ 00050 int SendJpeg(uint8_t * buf, uint32_t size); 00051 00052 /** Attach a function to call whenever a serial interrupt is generated 00053 * 00054 * @param func A pointer to a void function, or 0 to set as none 00055 */ 00056 void SetCallback(Callback<void()> func); 00057 00058 /** Attach a function to call when touch panel int 00059 * 00060 * @param obj pointer to the object to call the member function on 00061 * @param method pointer to the member function to be called 00062 */ 00063 template<typename T> 00064 void SetCallback(T* obj, void (T::*method)()) { 00065 // Underlying call thread safe 00066 SetCallback(callback(obj, method)); 00067 } 00068 00069 /** Attach a member function to call when touch panel int 00070 * 00071 * @param obj pointer to the object to call the member function on 00072 * @param method pointer to the member function to be called 00073 */ 00074 template<typename T> 00075 void SetCallback(T* obj, void (*method)(T*)) { 00076 // Underlying call thread safe 00077 SetCallback(callback(obj, method)); 00078 } 00079 /** Get the maximum number of simultaneous touches 00080 * 00081 * @return The maximum number of simultaneous touches. 00082 */ 00083 int GetMaxTouchNum(void); 00084 00085 /** Get the coordinates 00086 * 00087 * @param touch_buff_num The number of structure p_touch. 00088 * @param p_touch Touch position information. 00089 * @return The number of touch points. 00090 */ 00091 int GetCoordinates(int touch_buff_num, touch_pos_t * p_touch); 00092 00093 char Getgetc(); 00094 00095 00096 private: 00097 typedef enum { 00098 POS_SEQ_INIT, 00099 POS_SEQ_START, 00100 POS_SEQ_X, 00101 POS_SEQ_X_POS, 00102 POS_SEQ_X_M, 00103 POS_SEQ_C, 00104 POS_SEQ_Y, 00105 POS_SEQ_Y_POS, 00106 POS_SEQ_Y_M, 00107 POS_SEQ_END, 00108 } pos_seq_t; 00109 00110 Thread displayThread; 00111 pos_seq_t pos_seq; 00112 int pos_x; 00113 int pos_y; 00114 Callback<void()> event; 00115 00116 void touch_int_callback(void); 00117 void display_app_process(); 00118 void SendHeader(uint32_t size); 00119 void SendData(uint8_t * buf, uint32_t size); 00120 }; 00121 #endif
Generated on Thu Jul 21 2022 01:16:51 by
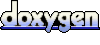